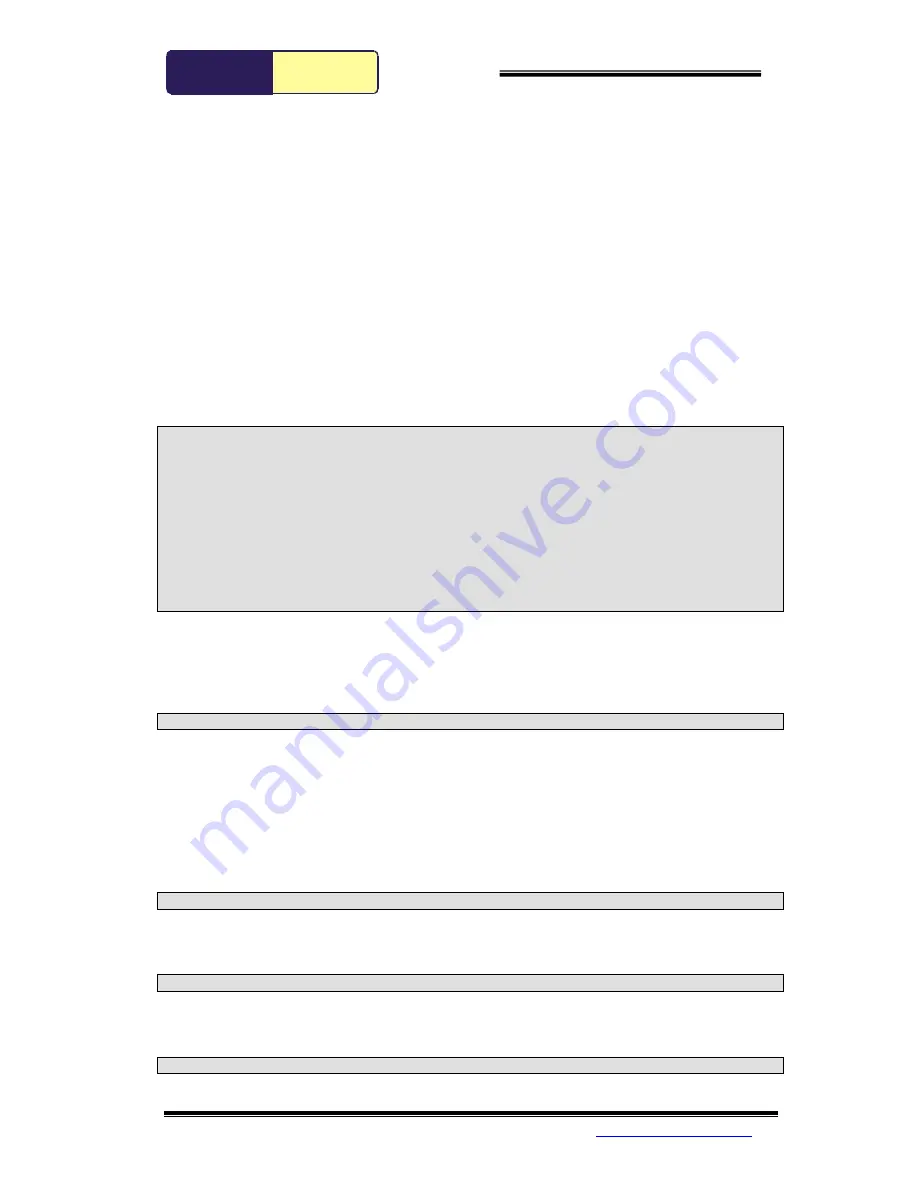
Ether I/O 24 Users Manual V1.3
Elexol Pty Ltd Version 1.3
Page 34
http://www.elexol.com
E L E
X O L
E L E C T R O N I C
S O L U T I O N S
Basic Programming (continued)
7.
Using the EEPROM
The Ether I/O 24 module EEPROM is programmed using several fixed length packets. Packet length is
always 5 bytes with the first byte being the ‘ character. Where all 5 bytes are not specifically needed, such
as a Read command where only the command and address need to be sent to the module, the packet is
padded with bytes whose value is 0. There are five different EEPROM specific and 1 special command in
the EEPROM set that resets the module. Because this command is used to activate the data stored into the
EEPROM it is placed in the EEPROM command set.
The five EEPROM commands are Read, Erase, Write, Write Enable and Write Disable. We will show
examples of how each command is used and describe when to use it.
The EEPROM Read command is used whenever we want to know the values stored in the EEPROM of the
module. The read command will respond with a 4-byte packet containing the R from the Read command
the address and the data from the EEPROM.
Dim ReadDone As Boolean
ReadDone = False
TimeOut = Timer + 0.2
Winsock1.SendData "'R" + Chr$(EEAddress) + Chr$(0) + Chr$(0)
While ReadDone = False And Timer < TimeOut
If Winsock1.BytesReceived <> 0 Then
Winsock1.GetData a$, vbString
If Len(a$) = 4 And Left$(a$, 2) = "R" + Chr$(EEAddress) Then
EEData(EEAddress) = Asc(Mid$(a$, 4, 1)) + Asc(Mid$(a$, 3, 1)) * 256!
ReadDone = True
End If
End If
Wend
If ReadDone = False Then Call MsgBox("No Response from Module", vbCritical, "Module Error"): Exit Sub
The EEPROM Write command is used to write a byte to the EEPROM memory. It is recommended to read
the EEPROM in the module after any write command to ensure the data is correct after the write cycle.
Allow a 10ms delay between writing the EEPROM word and reading it, this gives time for the EEPROM to
correctly program the memory location.
Winsock1.SendData "'W" + Chr$(EEAddress) + Chr$(EEDataMSB) + Chr$(EEDataLSB)
This code writes the Values in EEDataMSB and EEDataLSB to the EEPROM memory at location
EEAddress. It should be noted that this command will not work unless an EEPROM Write Enable
command has first been sent. This command should be followed by an EEPROM Read Command to verify
that the data has been written to the EEPROM successfully.
Erasing an EEPROM memory location is accomplished by using the EEPROM Erase Command. The only
data that is needed for the Erase function is the Address to erase so we use the extra 2 bytes to the
command to confirm that the command is valid.
Winsock1.SendData "'E" + Chr$(EEAddress) + Chr$(&HAA) + Chr$(&H55)
As with the Write command an EEPROM Write Enable Command must be sent before the Erase command
will work. The code for executing an EEPROM Write Enable command is as follows.
Winsock1.SendData "'1" + Chr$(0) + Chr$(&HAA) + Chr$(&H55)
After the Write or Erase commands are complete you should protect the EEPROM from accidental writes
by sending the EEPROM Write Disable Command. Here is the code for the write disable command.
Winsock1.SendData "'0" + Chr$(0) + Chr$(0) + Chr$(0)