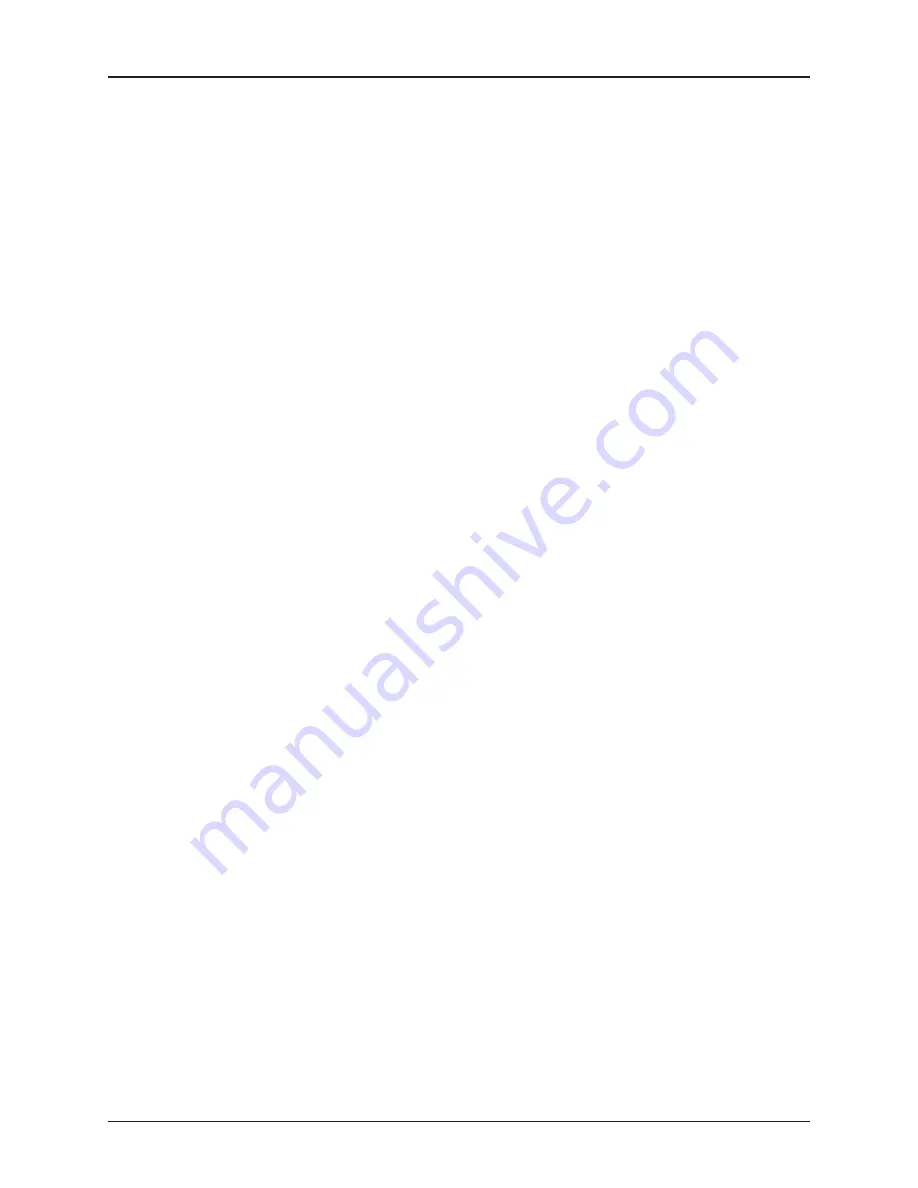
It is possible to implement delays of 5ms, 2ms, 1ms, 500
m
s, 200
m
s and 100
m
s by utilizing other
PIT modes.
The PIT can generate an interrupt whenever the PIT rolls over. The system programmer must
initialize the interrupt vector, enable PIC interrupts, etc. The following code fragment shows the
basic interrupt handling function.
static volatile signed long int dCounter;
#pragma interrupt (vInterruptHandler)
static void far vInterruptHandler (void)
{
/*
* clear the source of the interrupt by resetting the rollover
* flag, thus:
*/
outbyte (CONTROL_STATUS, inbyte (CONTROL_STATUS) & ~INTERRUPT_MASK);
/*
* perform the relevant actions to acknowledge the interrupt
* in the PIC, etc ...
*/
dCounter--;
}
The following code fragment used in conjunction with the previous code fragment illustrates
another method of implementing a timed delay. The dCounter variable is declared to be volatile
which prevents any C compilers, which conform to the ANSI standard, from optimizing accesses
to the dCounter variable.
outbyte (CONTROL_STATUS, MODE_RUN_STOP);
outbyte (TIMER_BYTE_0, 0);
outbyte (TIMER_BYTE_1, 0);
outbyte (TIMER_BYTE_2, 0);
outbyte (TIMER_BYTE_3, 0);
outbyte (CONTROL_STATUS, MODE_PIT_100Hz | MODE_RUN_GO);
dCounter = 500; /* 500 * (1 / 100) == 5 seconds */
/*
* install the interrupt for the PIT counter, modify the
* PIC settings, etc. and ensure interrupts are enabled.
*/
while (dCounter > 0)
; /* do nothing ... */
outbyte (CONTROL_STATUS, MODE_RUN_STOP);
The following code fragment uses the LDT to measure the elapsed time to a resolution of 1
m
s.
In this example, the LDT is zeroed at the start of the test and so there is no need to subtract the
LDT’s initial value from its final value.
static UINT32 dElapsedTime;
outbyte (CONTROL_STATUS, MODE_RUN_STOP);
outbyte (TIMER_BYTE_0, 0);
outbyte (TIMER_BYTE_1, 0);
outbyte (TIMER_BYTE_2, 0);
outbyte (TIMER_BYTE_3, 0);
outbyte (CONTROL_STATUS, MODE_LDT | MODE_RUN_GO);
/*
* perform action to be timed ...
*/
9-16
PP 110/01x
Additional Local I/O Functions
Artisan Technology Group - Quality Instrumentation ... Guaranteed | (888) 88-SOURCE | www.artisantg.com