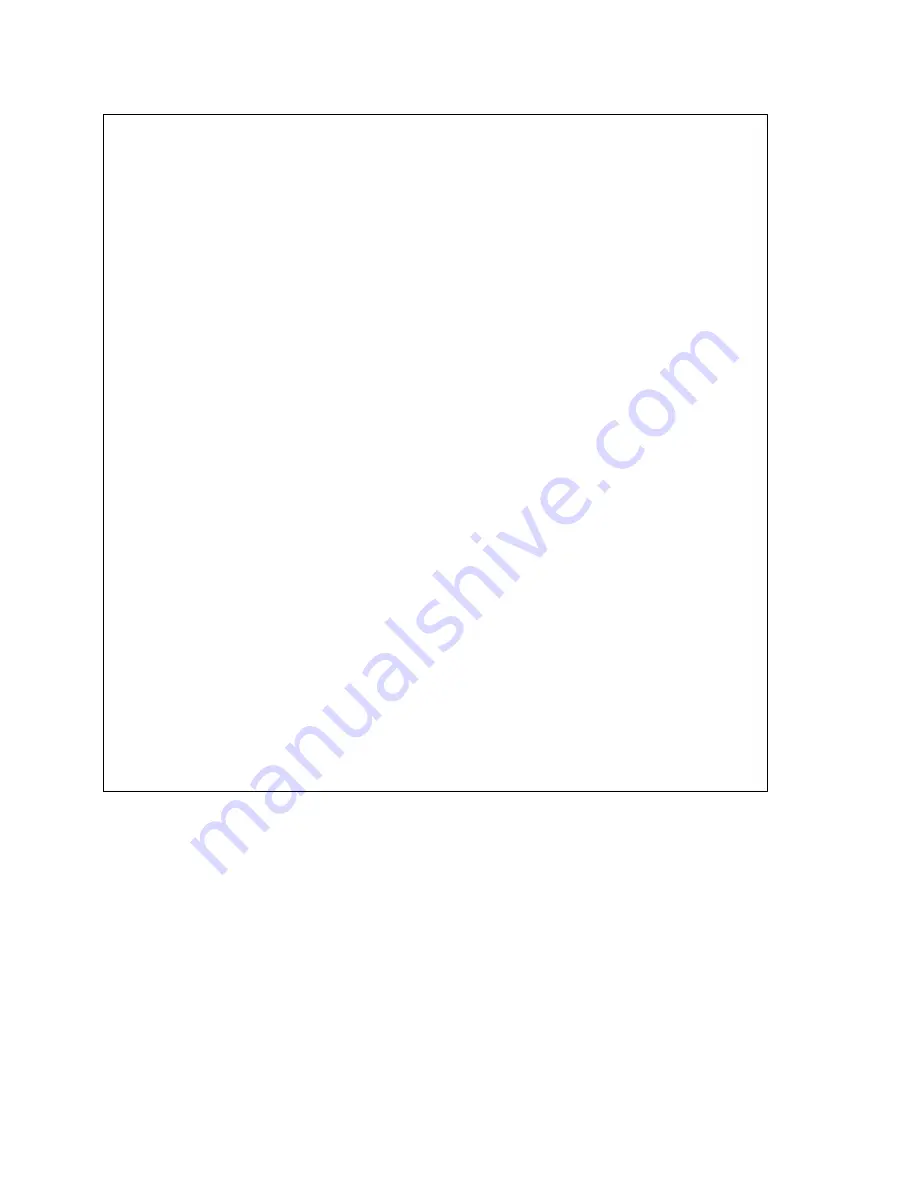
27
Figure 9 M-Module Driver Example Using Arrays
3.4.2.3
Pointer Unpacking
Pointer can be de-referenced and converted to a Lua type using one of the functions
alien.tostring
,
alien.toint
,
alien.toshort
,
alien.tolong
,
alien.tofloat
, and
alien.todouble.
These functions should be used with care as they do not check whether the
dereference or the typecast is safe. More robust unpacking can be performed using the
alien.struct.unpack
function.
3.4.2.4
Callback Functions
I some rare cases an M-Module driver will take a function pointer as a parameter and call that
function to perform a certain task. This is known as a callback function. Alien provides the
require "alien"
local libm228 = alien.load("/usr/scripts/user/libm228.so")
-----------------------------------------------------------
-- Function Prototypes
-----------------------------------------------------------
local m228_Init = libm228.m228_Init
m228_Init:types("int", "int", "int", "int", "ref int")
local m228_ReadFifoBuffer = libm228.m228_ReadFifoBuffer
m228_ReadFifoBuffer:types("int", "int", "int", "int", "pointer",
"pointer", "pointer", "ref int")
local m228_Close = libm228.m228_Close
m228_Close:types("int", "int")
-----------------------------------------------------------
-- Start of Script --
-----------------------------------------------------------
result, handle = m228_Init(1,1,1, 0)
time_array = alien.array("int", 256)
real_array = alien.array("double", 256)
raw_array = alien.array("short", 256)
result, num_read = m228_ReadFifoBuffer(handle, 0,
time_array.length, time_array.buffer, real_array.buffer,
raw_array.buffer, 0)
for offset=0, 256, 1
do
print(string.format('0x%x', raw_array[i])
end
m228_Close(handle)
print("Done!")