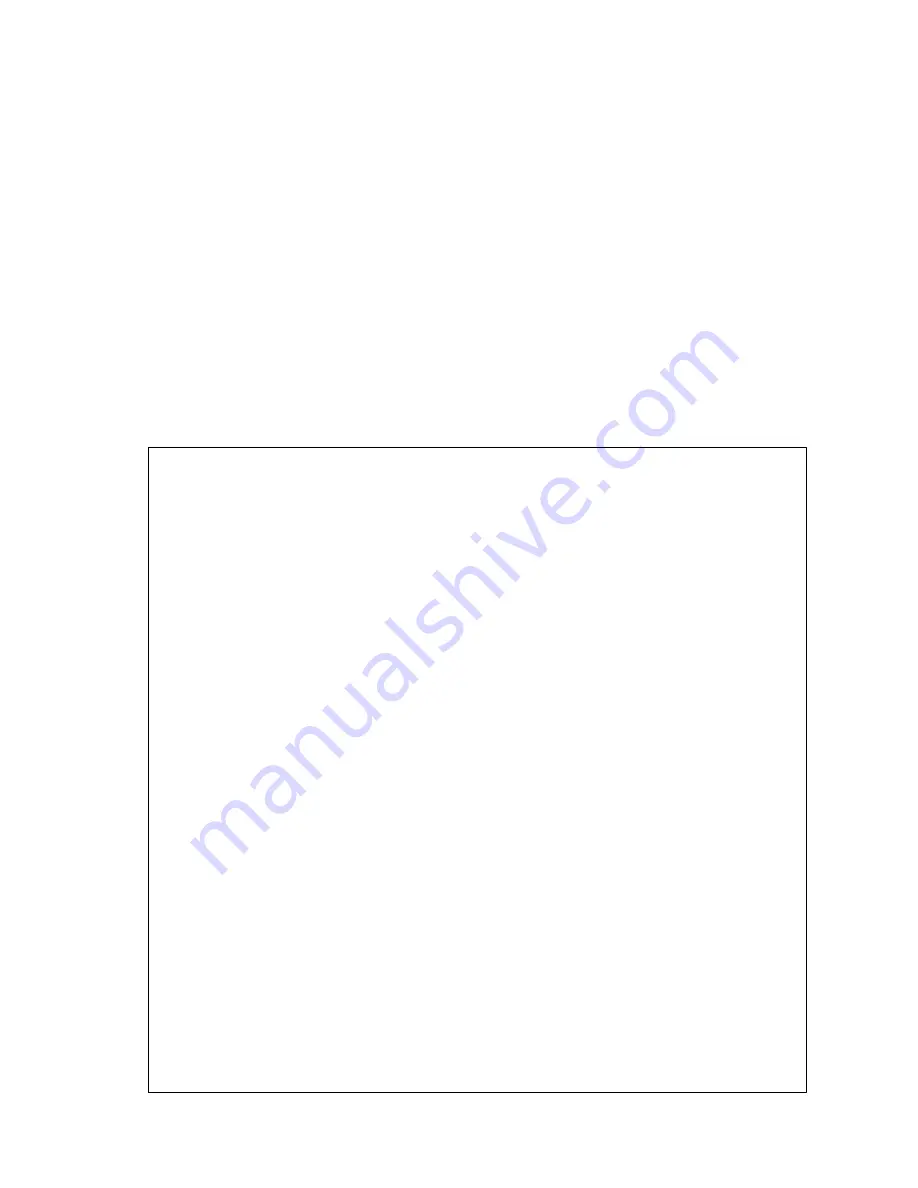
24
You may find it strange that there are two variables specified on the left side of the equal sign.
The first variable is the return value of the function. The second and subsequent variables are the
return values of the parameters that are passed by reference. Alien supports by-reference types
by allocating space for the argument on the Lua stack then initializing this stack space to the Lua
number (typecasting where needed) specified in the function call (0 in the example above).
When the C library function returns, Alien takes the value and returns it (converting where
appropriate) after the functions normal return value.
To put this all together, the example source code in Figure 7 uses the M228 M-module driver to
read 20 locations of the M-modules ID PROM. This example uses three driver functions:
m228_Init
,
m228_GetIdentWord
, and
m228_Close
. Note that these examples use the
m228_GetIdentWord
function not the EM405-8 Extensions Library function
mreadid
. This
same example can be performed using just the EM405-8 Extensions Library. The example prints
a line to the standard output for each ID location indicating the ID address and the value read.
Note that the functions
and
string.format
are part of the Lua standard libraries.
require "alien"
require "alien.struct"
local libm228 = alien.load("/usr/scripts/user/libm228.so")
-----------------------------------------------------------
-- Function Prototypes
-----------------------------------------------------------
local m228_Init = libm228.m228_Init
m228_Init:types("int", "int", "int", "int", "ref int")
local m228_GetIdentWord = libm228.m228_GetIdentWord
m228_GetIdentWord:types("int", "int", "int", "ref int")
local m228_Close = libm228.m228_Close
m228_Close:types("int", "int")
-----------------------------------------------------------
-- Start of Script --
-----------------------------------------------------------
id_query = 1
reset = 1
module = 1
result, handle = m228_Init(module,id_query,reset, 0)
for offset=0, 19, 1
do
result, value = m228_GetIdentWord(handle, offset, 0)
print(string.format('0x%x: 0x%x', offset, value))
end
m228_Close(handle)
print("Done!")