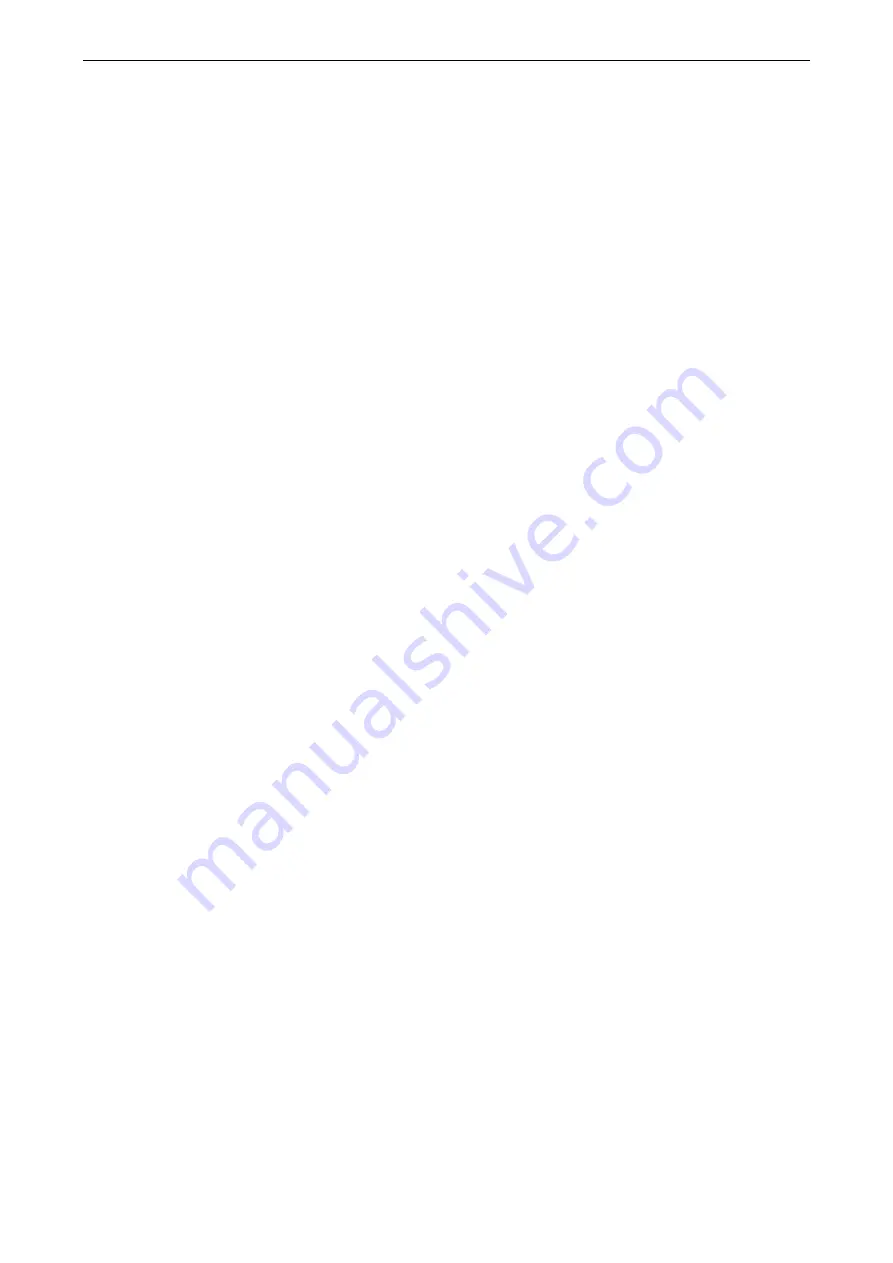
DA-681 Linux
Software Configuration
2-17
exit(0);
}
/*
* The convenient watchdog API --- libswtd.c
*/
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
// following for sWatchDog implement
#define IOCTL_SWATCHDOG_ENABLE 1
#define IOCTL_SWATCHDOG_DISABLE 2
#define IOCTL_SWATCHDOG_GET 3
#define IOCTL_SWATCHDOG_ACK 4
int swtd_open(void)
{
return open("/dev/swtd", O_RDWR);
}
int swtd_enable(int fd, unsigned long time)
{
return ioctl(fd, IOCTL_SWATCHDOG_ENABLE, &time);
}
int swtd_disable(int fd)
{
return ioctl(fd, IOCTL_SWATCHDOG_DISABLE, NULL);
}
int swtd_get(int fd, int *mode, unsigned long *time)
{
struct {
int
mode;
unsigned long time;
} nowset;
int
ret;
ret = ioctl(fd, IOCTL_SWATCHDOG_GET, &nowset);
*mode = nowset.mode;
*time = nowset.time;
return ret;
}
int swtd_ack(int fd)
{
return ioctl(fd, IOCTL_SWATCHDOG_ACK, NULL);
}
int swtd_close(int fd)
{
return close(fd);
}
The makefile is shown below:
all:
gcc xxxx.c libswtd.c –o xxxx
Example 2:
#include <stdio.h>
#include <stdlib.h>
#include <signal.h>
#include <string.h>
#include <sys/stat.h>
#include <sys/ioctl.h>
#include <sys/select.h>
#include <sys/time.h>
#include <moxadevice.h>
static void mydelay(unsigned long msec)
{