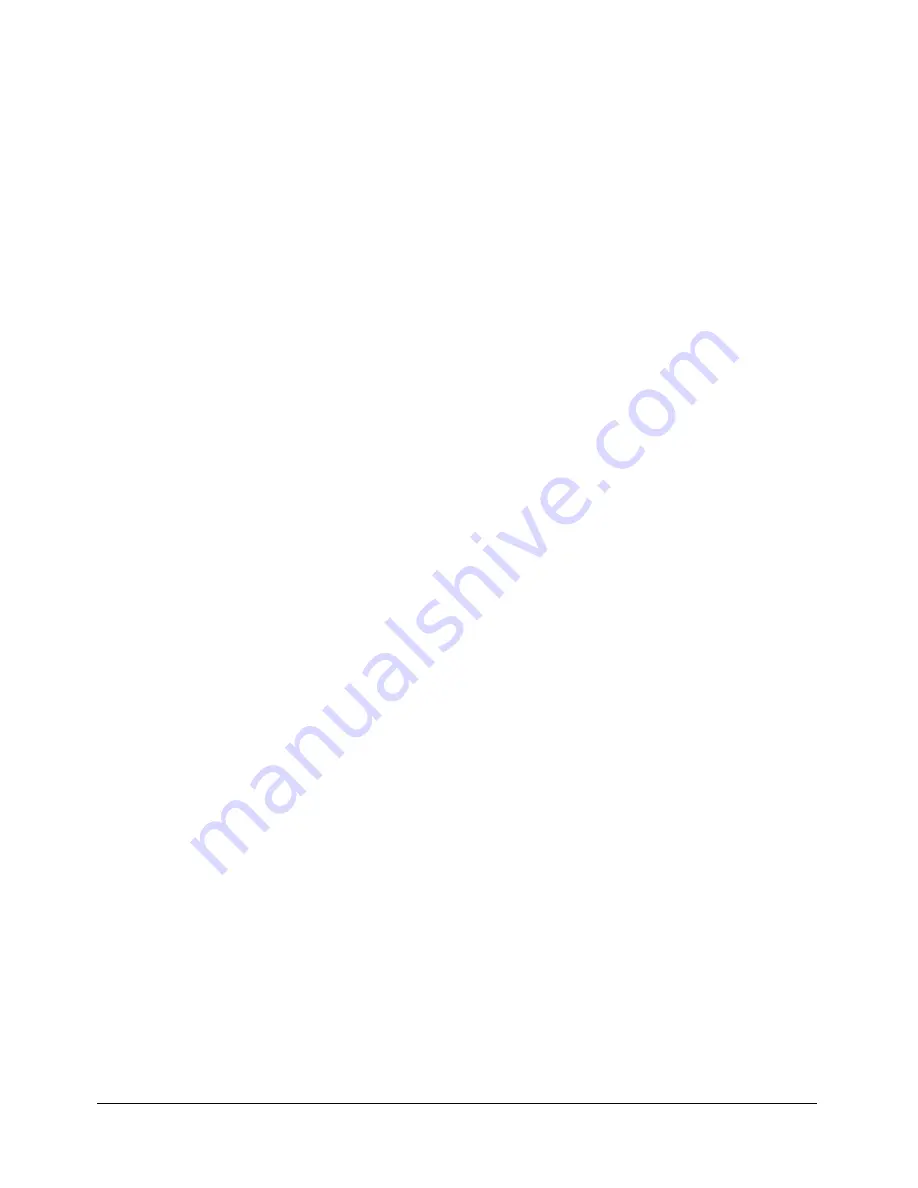
56
Chapter 2: Creating Custom Classes with ActionScript 2.0
Multiple inheritance, or inheriting from more than one class, is not allowed in ActionScript 2.0.
However, classes can effectively inherit from multiple classes if you use individual
extends
statements, as shown in the following example:
// not allowed
class C extends A, B {}
// allowed
class B extends A {}
class C extends B {}
You can also use interfaces to provide a limited form of
multiple inheritance
. See
“Interfaces”
on page 47
and
“Creating and using interfaces” on page 58
.
Creating dynamic classes
By default, the properties and methods of a class are fixed. That is, an instance of a class can’t
create or access properties or methods that weren’t originally declared or defined by the class. For
example, consider a Person class that defines two properties,
name
and
age
:
class Person {
var name:String;
var age:Number;
}
If, in another script, you create an instance of the Person class and try to access a property of the
class that doesn’t exist, the compiler generates an error. For example, the following code creates a
new instance of the Person class (
a_person
) and then tries to assign a value to a property named
hairColor
, which doesn’t exist:
var a_person:Person = new Person();
a_person.hairColor = "blue"; // compiler error
This code causes a compiler error because the Person class doesn’t declare a property named
hairColor
. In most cases, this is exactly what you want to happen. Compiler errors may not seem
desirable, but they are very beneficial to programmers; good error messages help you to write
correct code, by pointing out mistakes early in the coding process.
In some cases, however, you might want to add and access properties or methods of a class at
runtime that aren’t defined in the original class definition. The
dynamic
class modifier lets you do
just that. For example, the following code adds the
dynamic
modifier to the Person class
discussed previously:
dynamic class Person2 {
var name:String;
var age:Number;
}
Now, instances of the Person class can add and access properties and methods that aren’t defined
in the original class, as shown in the following example:
var a_person:Person2 = new Person2();
a_person.hairColor = "blue";//no compiler error because class is dynamic
trace(a_person.hairColor);
Содержание FLEX
Страница 1: ...Flex ActionScript Language Reference ...
Страница 8: ......
Страница 66: ...66 Chapter 2 Creating Custom Classes with ActionScript 2 0 ...
Страница 76: ......
Страница 133: ...break 133 See also for for in do while while switch case continue throw try catch finally ...
Страница 135: ...case 135 See also break default strict equality switch ...
Страница 146: ...146 Chapter 5 ActionScript Core Language Elements See also break continue while ...
Страница 229: ...while 229 i 3 The following result is written to the log file 0 3 6 9 12 15 18 See also do while continue for for in ...
Страница 808: ...808 Chapter 7 ActionScript for Flash ...
Страница 810: ...810 Appendix A Deprecated Flash 4 operators ...
Страница 815: ...Other keys 815 Num Lock 144 186 187 _ 189 191 192 219 220 221 222 Key Key code ...
Страница 816: ...816 Appendix B Keyboard Keys and Key Code Values ...
Страница 822: ...822 Index ...