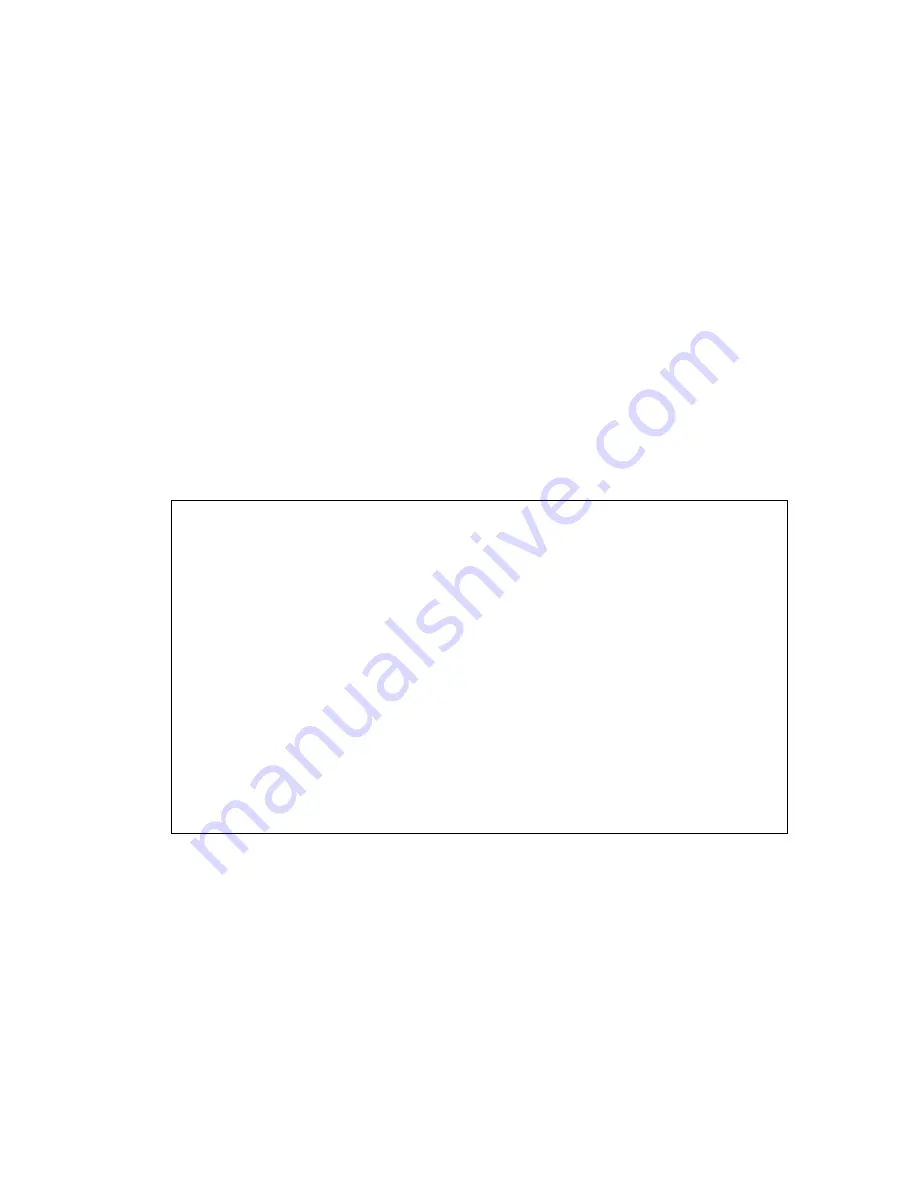
TME-104P-CSR-LX800-R1V11.doc
Rev 1.11
43 (50)
4.5
Programming Examples
The following programming examples are made for a Linux operation system. If other operation systems are used
some header files could be unnecessary or they can have different names.
The "iopl()" function is a Linux specific one, in Windows XP a tool called "porttalk" can be used instead.
Be careful with the interpretation of the "outb" order in our examples:
Linux:
"outb(value, address)"
DOS, Windows:
"outb(address, value)"
The following example is meant to be compiled using gcc under Linux.
LIVE-LED
The Live-LED can be programmed by users. The cathode of the mounted LED is connected to a GPIO pin of the
Super I/O. If the input has ground potential the LED is on.
The Live-LED (red) can be controlled with bit 0 of I/O port 1220h (Super I/O GP10). The BIOS signals with it that
the POST is in progress. After that, the LED may be freely used by any application program.
The following Linux program changes the state of the Live-LED.
#include <stdio.h>
#include <sys/io.h>
#define PORT 0x1220
#define MASK 0x01
int main()
{
unsigned char data;
if (iopl(3)) {
//get port access permissions (must be root)
perror("iopl"); return 1;
}
data = inb(PORT);
//read GPIOs
if (data & MASK) {
//isolate LED bit (inverse logic!)
printf("Live LED was off, switching it on.\n");
outb(data & ~MASK, PORT);
} else {
printf("Live LED was on, switching it off.\n");
outb(data | MASK, PORT);
}
iopl(0);
return 0;
}