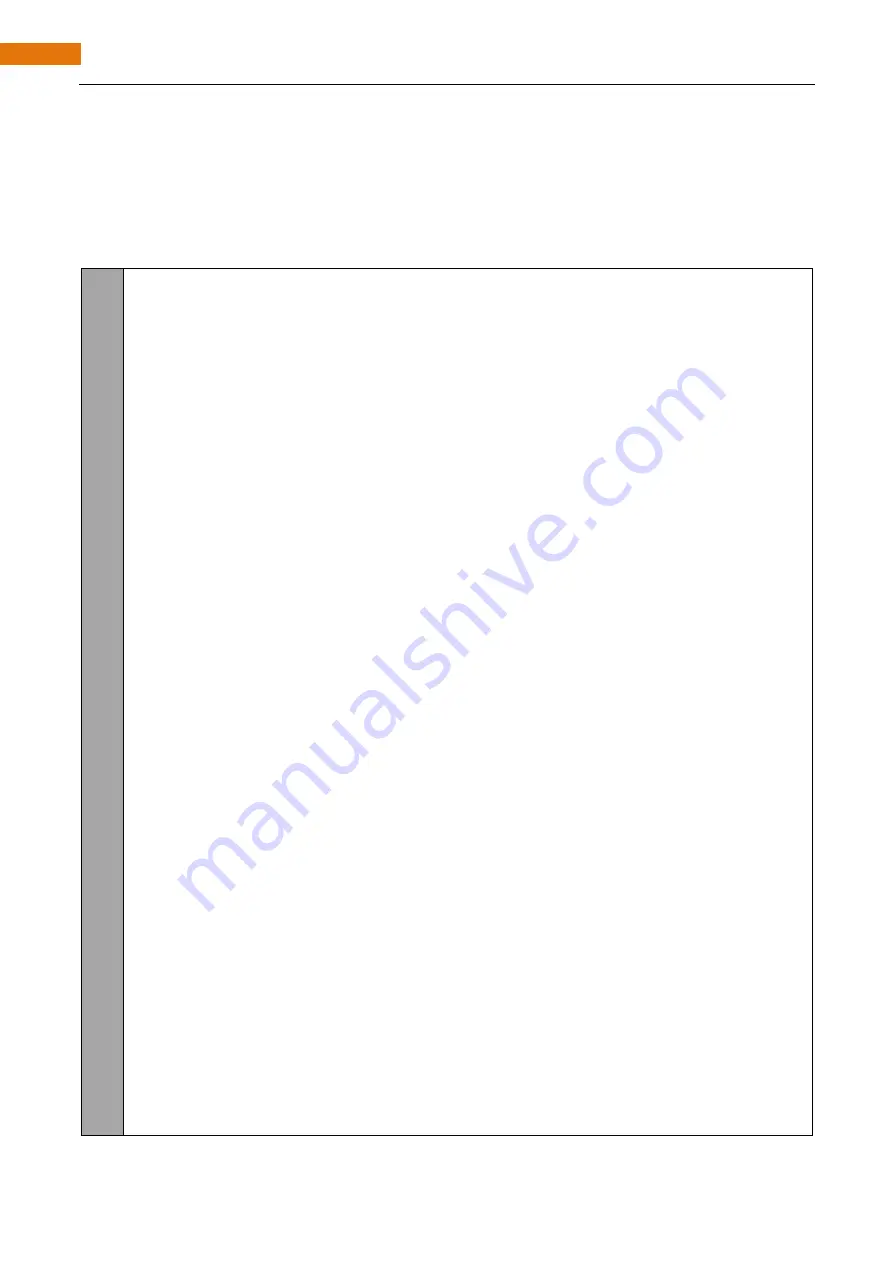
6
RFID
www.freenove.com █
Sketch
Sketch 1
Before writing the code, we need import the library RFID.zip first.
This sketch will read the unique ID number (UID) of the card, recognize the type of the card and display the
information through serial port.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
#include <SPI.h>
#include <RFID.h>
//D10:pin of card reader SDA. D9:pin of card reader RST
RFID rfid
(
10
,
9
);
unsigned
char
status
;
unsigned
char
str
[
MAX_LEN
];
//MAX_LEN is 16: size of the array
void
setup
()
{
Serial
.
begin
(
9600
);
SPI
.
begin
();
rfid
.
init
();
//initialization
Serial
.
println
(
"Please put the card to the induction area..."
);
}
void
loop
()
{
//Search card, return card types
if
(
rfid
.
findCard
(
PICC_REQIDL
,
str
)
==
MI_OK
)
{
Serial
.
println
(
"Find the card!"
);
// Show card type
ShowCardType
(
str
);
//Anti-collision detection, read card serial number
if
(
rfid
.
anticoll
(
str
)
==
MI_OK
)
{
Serial
.
(
"The card's number is : "
);
//Display card serial number
for
(
int
i
=
0
;
i
<
4
;
i
++)
{
Serial
.
(
0x0F
&
(
str
[
i
]
>>
4
),
HEX
);
Serial
.
(
0x0F
&
str
[
i
],
HEX
);
}
Serial
.
println
(
""
);
}
//card selection (lock card to prevent redundant read, removing the line will make
the sketch read cards continually)
rfid
.
selectTag
(
str
);
}