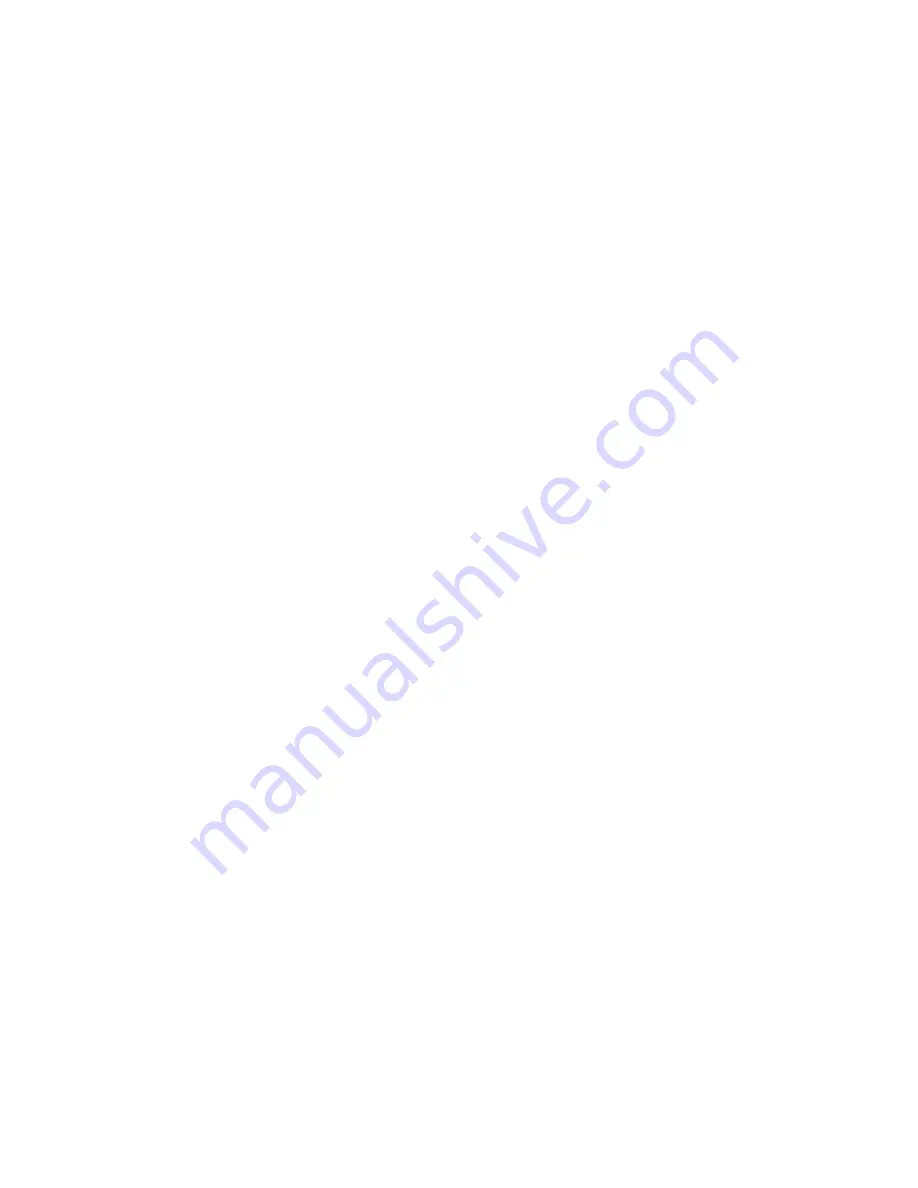
COMMERCIAL IN CONFIDENCE
Page 15
Reading the CAL Controllers Setpoint
An 8 byte message must be transmitted to the CAL Controller as follows:
byte 0
:
Slave address
xx
byte 1
:
Read Register Function code
03 hex
byte 2
:
High Byte of Register address
00 hex
byte 3
:
Low byte of Register address
7F hex
byte 4
:
Number of Registers to read (high byte)
00 hex
byte 5
:
Number of Registers to read (low byte)
01 hex
byte 6
:
CRC lo byte
xx
byte 7
:
CRC hi byte
xx
Note that the CRC must be transmitted with the lo byte first. Bytes must be transmitted in a single
burst, without gaps between each byte - any gap of longer than 1.5 times a character width will cause
the CAL Controller to ignore the message.
The following example shows how to construct a message to read the setpoint, the various sections of
this code would normally be held in separate functions, and would be optimised for better speed, but
this example shows the thought process involved (note also that C uses zero based arrays):
unsigned char mess[8], reply[8];
void BuildMessageToReadSetPoint()
{
unsigned char highbyte,lowbyte;
unsigned short crc,thisbyte,i,shift,lastbit; /* 16 bit word values */
mess[0] = 0x01;
/* slave address */
mess[1] = 0x03;
/* read function */
mess[2] = 0x00;
/* address hi byte */
mess[3] = 0x7F;
/* address lo byte */
mess[4] = 0x00;
/* number of data points hi byte */
mess[5] = 0x01;
/* number of data points lo byte */
/* compute the CRC over the first 6 chars of the message */
crc=0xffff;
for (i=0; i<=5; i++)
{
thisbyte = mess[i];
crc = crc ^ thisbyte;
for (shift = 1; shift <= 8; shift++)
{
lastbit = crc & 0x0001;
crc = (crc >> 1) & 0x7fff;
if (lastbit == 0x0001)
{
crc = crc ^ 0xa001 ;
}
}
}
highbyte = (crc >> 8) & 0xff;
lowbyte = crc & 0xff;
mess[6] = lowbyte;
mess[7] = highbyte;
}
the 8 characters in the message can now be transmitted to the communications port.