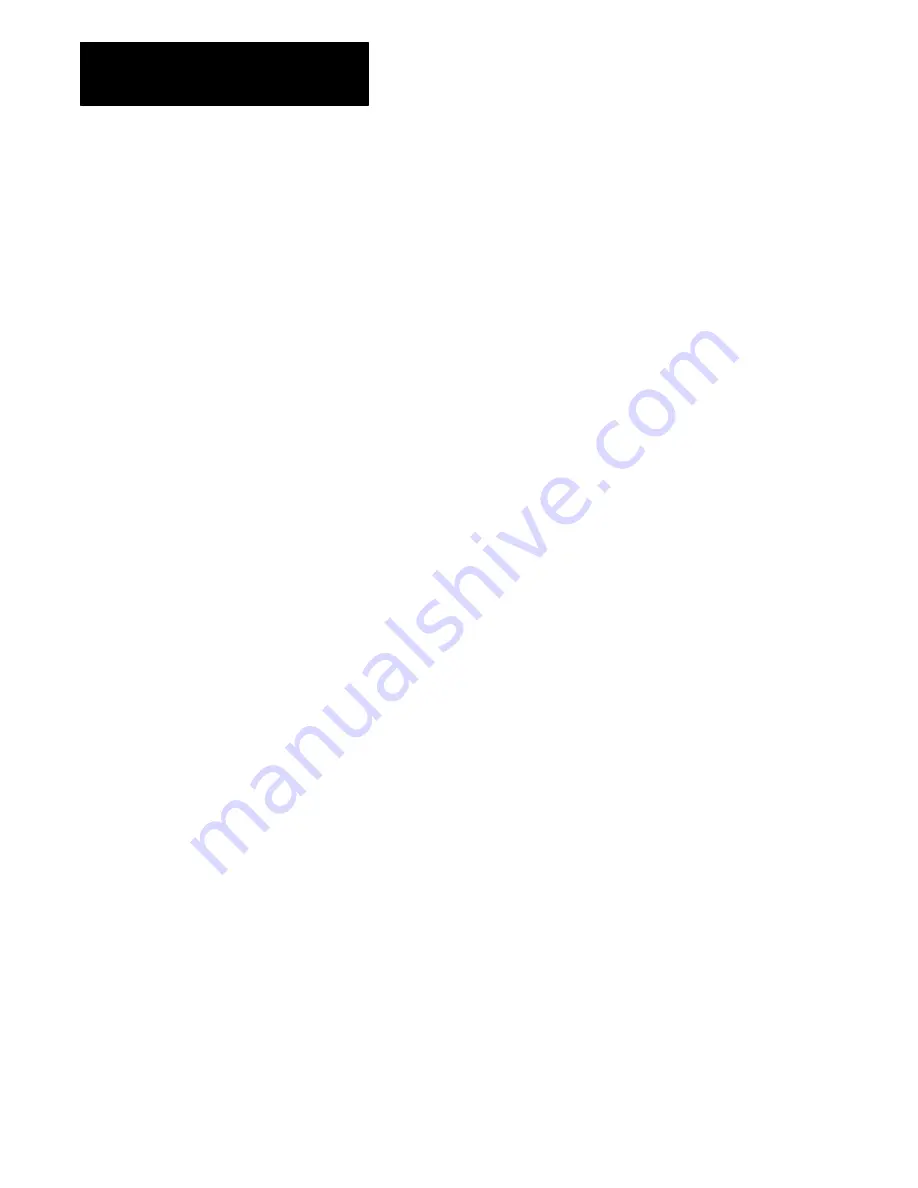
Programming Examples
Appendix B
B-14
confirm.c file
/***************************************************************************
**
**
UBYTE get_confirmation (KTX_DUALPORT far * dp,
**
UBYTE trans_num, USHORT timeout)
**
**
This routine waits for <timeout> seconds for a confirmation to
**
become available. It then validates the transaction number and
**
returns status to the calling routine. It is the caller’s
**
responsibility to acknowledge the confirmation.
**
**
INPUTS
**
KTX_DUALPORT far *dp – points to the base of the KTX dualport
**
UBYTE trans_num
– transaction_number
**
USHORT timeout
– time (in seconds) to wait for completion
**
**
OUTPUT
**
UBYTE status – completion status of the command
**
***************************************************************************/
#include <time.h>
#include <stdlib.h>
#include ”ktx_dp.h”
/* 1784–KTX Scanner Dualport definition
*/
#include ”ktx_err.h”
/* 1784–KTX Scanner error codes
*/
#include ”ktxconst.h”
/* 1784–KTX Scanner constants
*/
UBYTE
get_confirmation (KTX_DUALPORT far *dp, UBYTE trans_num, USHORT timeout)
{
/**** Initial values ****/
time_t
t0 = time(NULL);
UBYTE
status = SUCCESS;
/**** Wait for confirmation ****/
while (dp–>int_status_to_host != CONFIRMATION_INTERRUPT) {
if ((time(NULL) – t0) > timeout) {
status = CONFIRMATION_TIMED_OUT;
break;
}
}
/**** Check interrupt type ****/
switch (dp–>int_status_to_host) {
/**** Handle non–confirmation types ****/
case PROCESSING_PROBLEM_INTERRUPT :
unrecoverable_error(dp);
break;
/**** Confirmations: match up transaction #’s ****/
/**** If they match, return completion status ****/
case CONFIRMATION_INTERRUPT :
if (dp–>confirmation_buffer.transaction_num != trans_num)
status = TRANS_NUM_MISMATCH;
else
status = dp–>confirmation_buffer.conf_status;
default:
break;
}
return status;
}