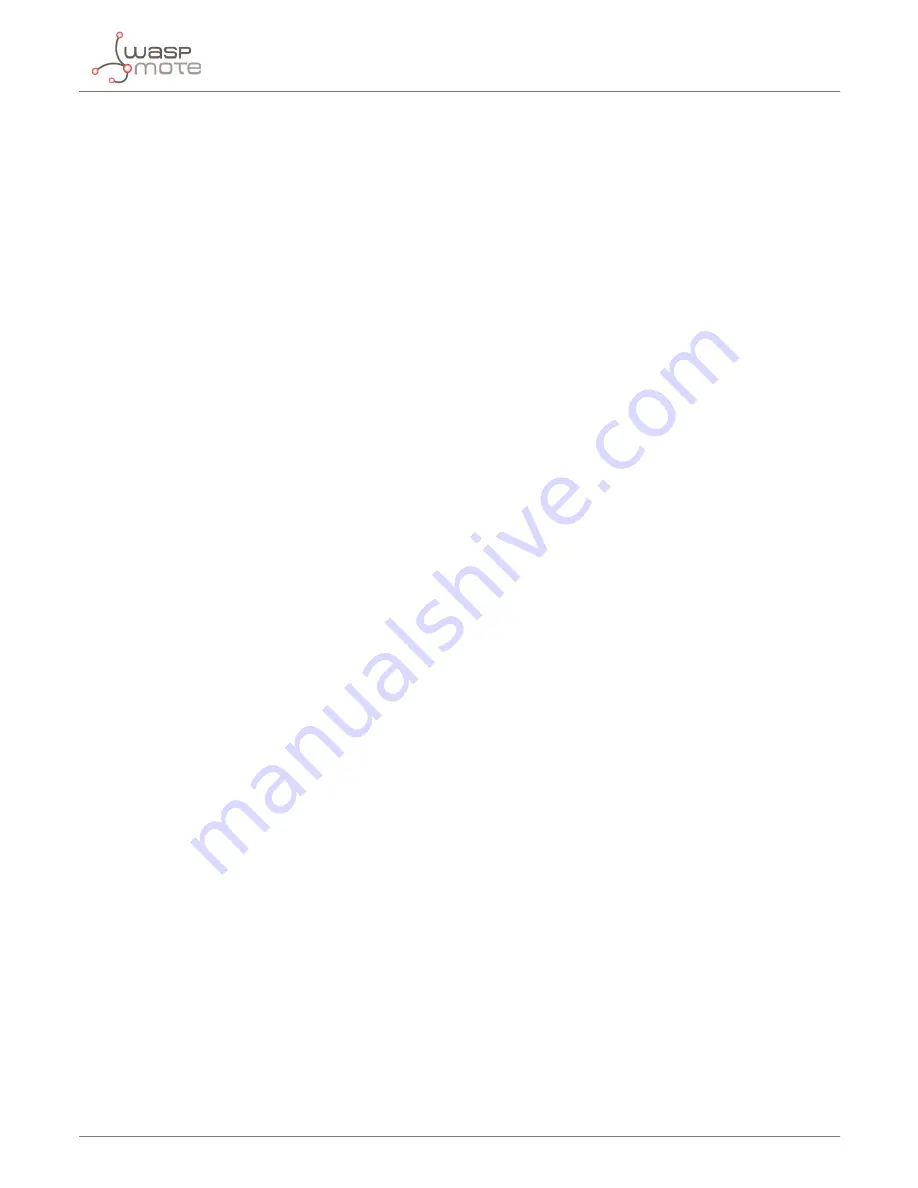
-19-
v7.0
Libelium’s library
8.10. Writing in a block and checking it
This function writes 16 bytes in a block, and then checks the correct data was written. This block must be authenticated before
writing. This function is useful to do these 2 steps in just one.
Example of use:
{
uint8_t state; // stores the status of the executed command
uint8_t aux[16]; // auxiliar buffer
uint8_t UID[4]; // stores the UID (unique identification) of a card
uint8_t key[6]; // stores the key or password
...
memset(key, 0xFF, 6); // the key by default, edit if needed
state = RFID13.init(UID, aux); // inits systems and look for cards
state = RFID13.authenticate(UID, 2, key); // authenticates the block
memset(aux, 0x00, 16); // stores 0’s in aux, 16 bytes
state = RFID13.writeAndCheck(aux, 2); // writes the content of aux in block 2, then checks
// its content is OK
}
8.11. Writing in a block with authentication and checking it
This function authenticates a block of the card first, then writes 16 bytes in the block, and then checks the correct data was
written. It is useful to do these 3 steps in just one.
Example of use:
{
uint8_t state; // stores the status of the executed command
uint8_t aux[16]; // auxiliar buffer
uint8_t UID[4]; // stores the UID (unique identification) of a card
uint8_t key[6]; // stores the key or password
...
memset(key, 0xFF, 6); // the key by default, edit if needed
state = RFID13.init(UID, aux); // inits systems and look for cards
state = RFID13.authenticate(UID, 2, key); // authenticates the block
memset(aux, 0x00, 16); // stores 0’s in aux, 16 bytes
writeAndCheckWithAuth(UID, key, aux, 2); // authenticates block 2, then writes the content of
// aux in it and checks its content is OK
}
8.12. Setting keys in a sector
This function authenticates a block of the card first, then sets the new keys A and B, and the access bits for a sector. After that, it
authenticates again and reads the trailer block. This function is useful to do these 4 steps in just one.
This function should be executed with care because if any of the internal functions is not successfully executed, then the sector
could be damaged or left unaccessible. That is why it is highly recommended to read the output status in order to check if all the
process was completed. Please place the card still and close distance from the RFID/NFC module’s antenna when setting new
keys; if the whole process is not properly completed, the access to that sector might be lost forever.
There are many options for the configuration of the access bits. We suggest to use the same options that the card has by default:
{0xFF, 0x07, 0x80, 0x69}. With this configuration:
•
data blocks must be authenticated with the key A, and then can be read/written
•
key A can only be changed (written) after an authentication with key A
•
access bits can be read or written after an authentication with key A
•
in the sector trailer, the key B has no use and can be read/written after an authentication with key A. It can be used as
additional storage space, for instance.