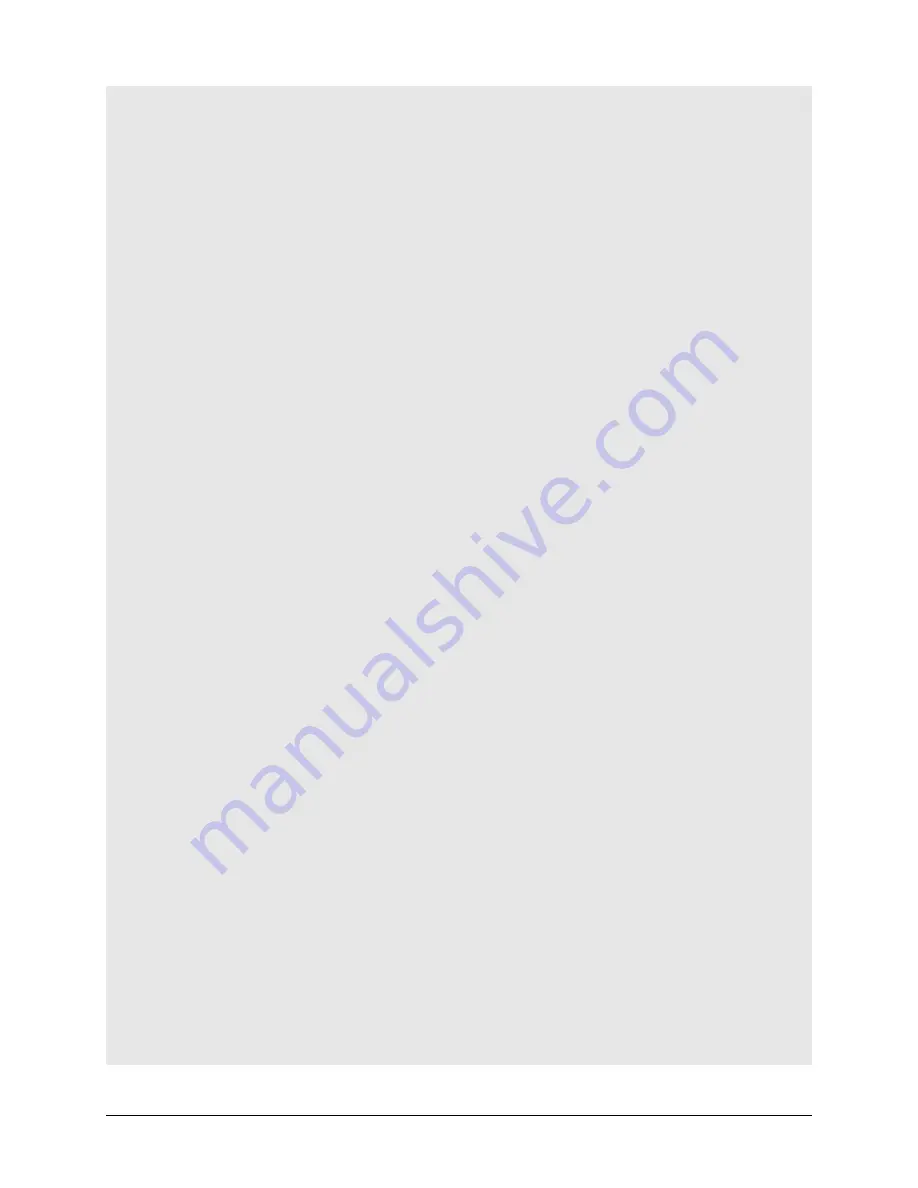
www.vtiinstruments.com
EMX-75XX Index
47
/* By default, ports are set to input direction and normal
polarity. This means we should be able to read the current state of the pins on the 5V
range, as long as we don't have an OverCurrent condition. Let's check for OverCurrent
before we read the data back. */
if (Dio.Normal.Ports.get_Item("PORT1").LatchedOverCurrent == true)
{
//The port is in an OverCurrent state, so we would want to
take some action here.
}
/* We want to check the Latched OverCurrent because while the
OverCurrent tells us if we are in an OverCurrent state now, the LatchedOverCurrent bit
will tell us if we have ever had one. If we have ever had an OverCurrent condition,
the port will be stuck in the reset state until we fix the inputs and call
ResetOverCurrent. Now that we've verified there's no OverCurrent condition,
Let's read some data. */
int data = Dio.Normal.Ports.get_Item("PORT1").Data;
/* We can also change our Polarity if we want to read the data inverted. */
Dio.Normal.Ports.get_Item("PORT1").Polarity =
VTEXDioPolarityEnum.VTEXDioPolarityInverse;
data = Dio.Normal.Ports.get_Item("PORT1").Data;
/*Now let's pick another port and set it to an output, and output
some data on it. Changing the direction to Output allows us to write data as well as
read it back. Note that we also have to set a voltage on the port before we can write
data.*/
Dio.Normal.Ports.get_Item("PORT2").Direction =
VTEXDioDirectionEnum.VTEXDioDirectionOutput;
Dio.Normal.Ports.get_Item("PORT2").VoltageRange = 3.3;
Dio.Normal.Ports.get_Item("PORT2").Data = 128;
/* Changing the Polarity of an output changes both the output and
readback polarity. This means the user will always read back what they wrote, but the
opposite signal will be placed on the line. */
Dio.Normal.Ports.get_Item("PORT2").Polarity =
VTEXDioPolarityEnum.VTEXDioPolarityInverse;
Dio.Normal.Ports.get_Item("PORT2").Data = 128; //Looks the same as
above, really outputting 127
data = Dio.Normal.Ports.get_Item("PORT2").Data; //Will return 128.
/* The Configure call lets us set all the values for a particular
port quickly. Here we will configure the port with a direction of Output, a Polarity
of Normal, a VoltageSource of User, and a voltage of 5V TTL Emulation (which has no
effect, since the voltage source is set to User. */
Dio.Normal.Ports.get_Item("PORT3").Configure(VTEXDioDirectionEnum.VTEXDioDirectionOutp
ut, VTEXDioPolarityEnum.VTEXDioPolarityNormal,
VTEXDioVoltageSourceEnum.VTEXDioVoltageSourceUser, -1.0);
/* We can also work with multiple ports at once. The ReadPorts and
WritePorts functions allow us to set or get data from several ports at a time. Setting
data on ports with an Input direction is ignored. */
Dio.Normal.WritePorts(VTEXDioDataWidthEnum.VTEXDioDataWidth16, 2,
255); //Writes a data of 255 on port 2, and 0 on port 3.
Dio.Close();
}
catch (COMException e)
{