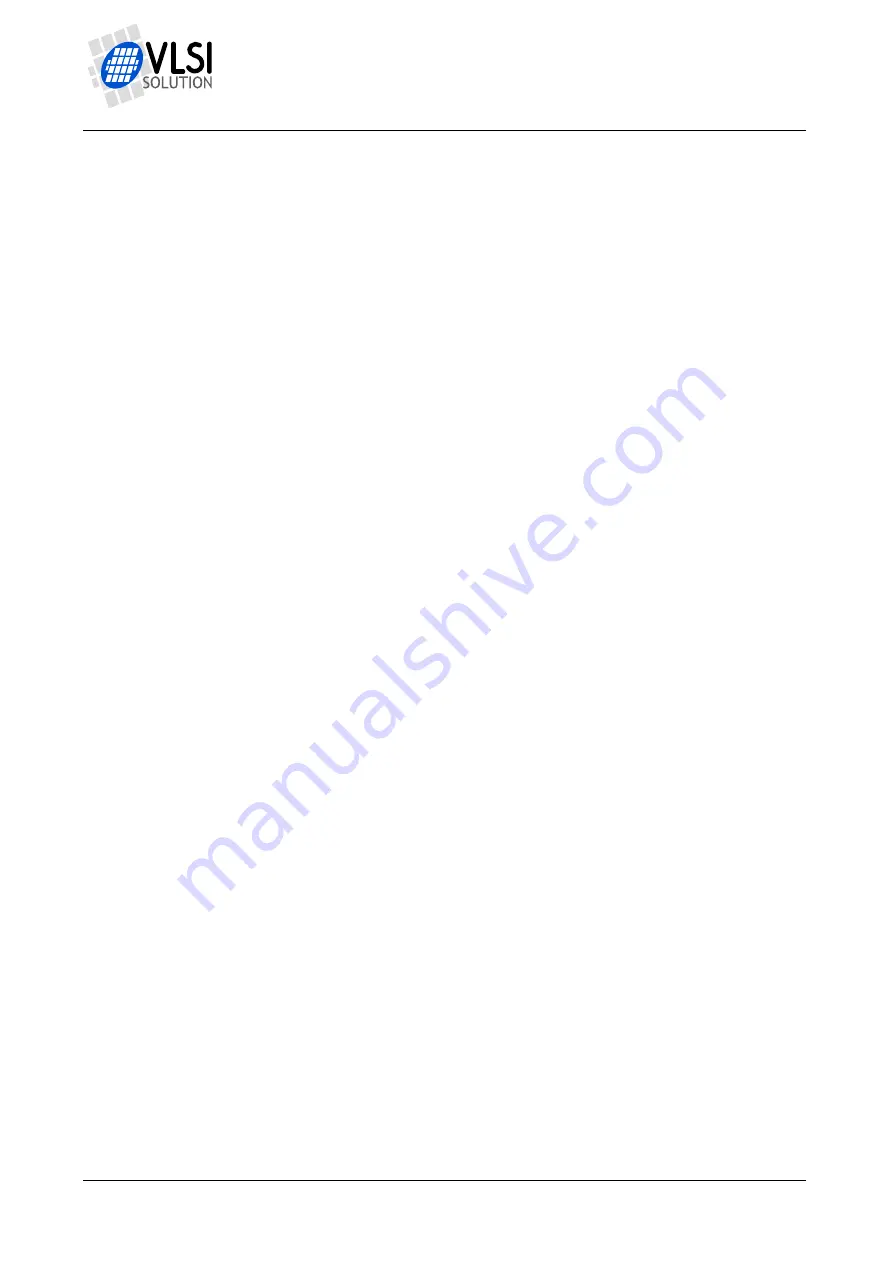
HH
VS1005 VSOS A
UDIO
S
UBSYSTEM
VS1005g
6.2
VSOS Audio Output Example Program
The following audio program example creates a low-intensity sine wave to the left chan-
nel, then outputs the samples.
#include <vo_stdio.h>
#include <stdlib.h>
#include <math.h>
#include <saturate.h>
#include <apploader.h>
#define SIN_TAB_SIZE 96
#define SIN_AMPLITUDE 1000 /* Max 32767 */
static const s_int16 __y sinTab[SIN_TAB_SIZE];
int main(void) {
// Remember to never allocate buffers from stack space. So, if you
// allocate the space inside your function, never forget "static"!
static s_int16 myBuf[2*SIN_TAB_SIZE];
int i;
/* Build sine table */
for (i=0; i<SIN_TAB_SIZE; i++) {
sinTab[i] = (s_int16)(sin(i*2.0*M_PI/SIN_TAB_SIZE)*SIN_AMPLITUDE);
}
while (1) {
// Clear buffer
memset(myBuf, 0, sizeof(myBuf));
// Create sine wave to the left channel.
for (i=0; i<SIN_TAB_SIZE; i++) {
myBuf[i*2] = sinTab[i];
}
// Write result
fwrite(myBuf, sizeof(s_int16), 2*SIN_TAB_SIZE, stdaudioout);
}
// Not really needed because there was a while(1) before
return EXIT_SUCCESS;
}
Rev. 3.57
2019-04-10
Page