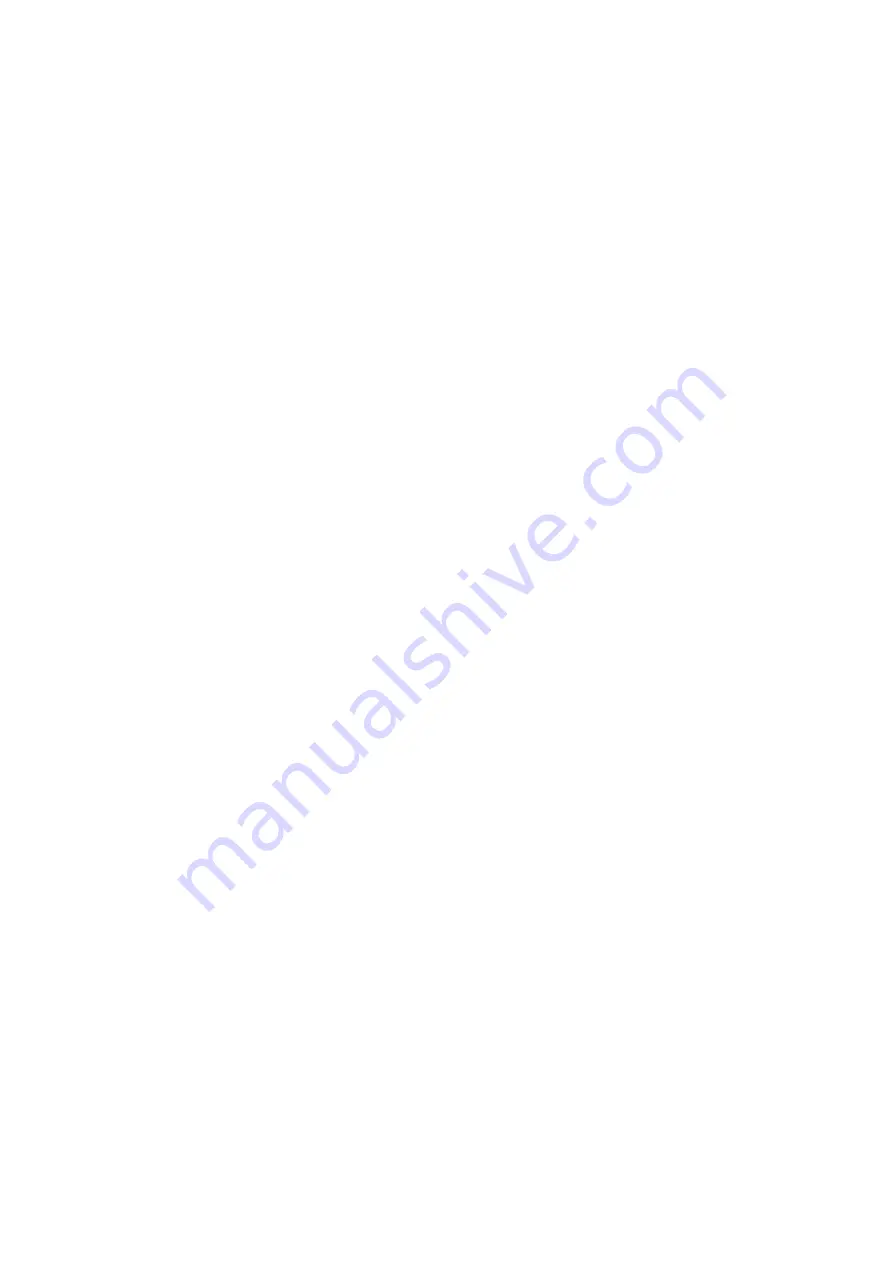
66
9.6.10
Using the API in C#
1.
Ensure that the DLL file is placed in the same folder as your application executable.
2.
Import the functions you need from the DLL into your source code with the Declare
statement:
[
DllImport
("can_api.dll", EntryPoint = "CAN_Open",
CallingConvention = CallingConvention.Cdecl)]
static extern Int32
CAN_Open(string SerialNrORComPortORNet,
string
szBitrate,
string
acceptance_code,
string
acceptance_mask,
Int32
flags,
UInt32
Mode);
3.
Create a definition of the CAN_MSG structure for the CAN_Write and CAN_Read
functions, if needed.
public struct
CAN_MSG
{
public UInt32
Id;
public byte
Size
;
[MarshalAs(UnmanagedType.
ByValArray, SizeConst = 8
)]
public byte[]
Data
;
public byte
Flags
;
public UInt16
TimeStamp
;
}
The keyword MarshalAs is used for all structure members to ensure that the
structure size corresponds to what the DLL expects.
4.
In order to communicate with the channel with other functions after opening it
with CAN_Open, you need to create a variable to store the handle value.
Int
myHandle;
myHandle
=
CAN_Open
((
"COM3"
,
"50"
,
"00000000"
,
"00000000"
,
1
,
2
);
5.
This concludes the basic setup process of using the DLL in C#. Imported functions
can then be easily called from the DLL with the parameters created above.