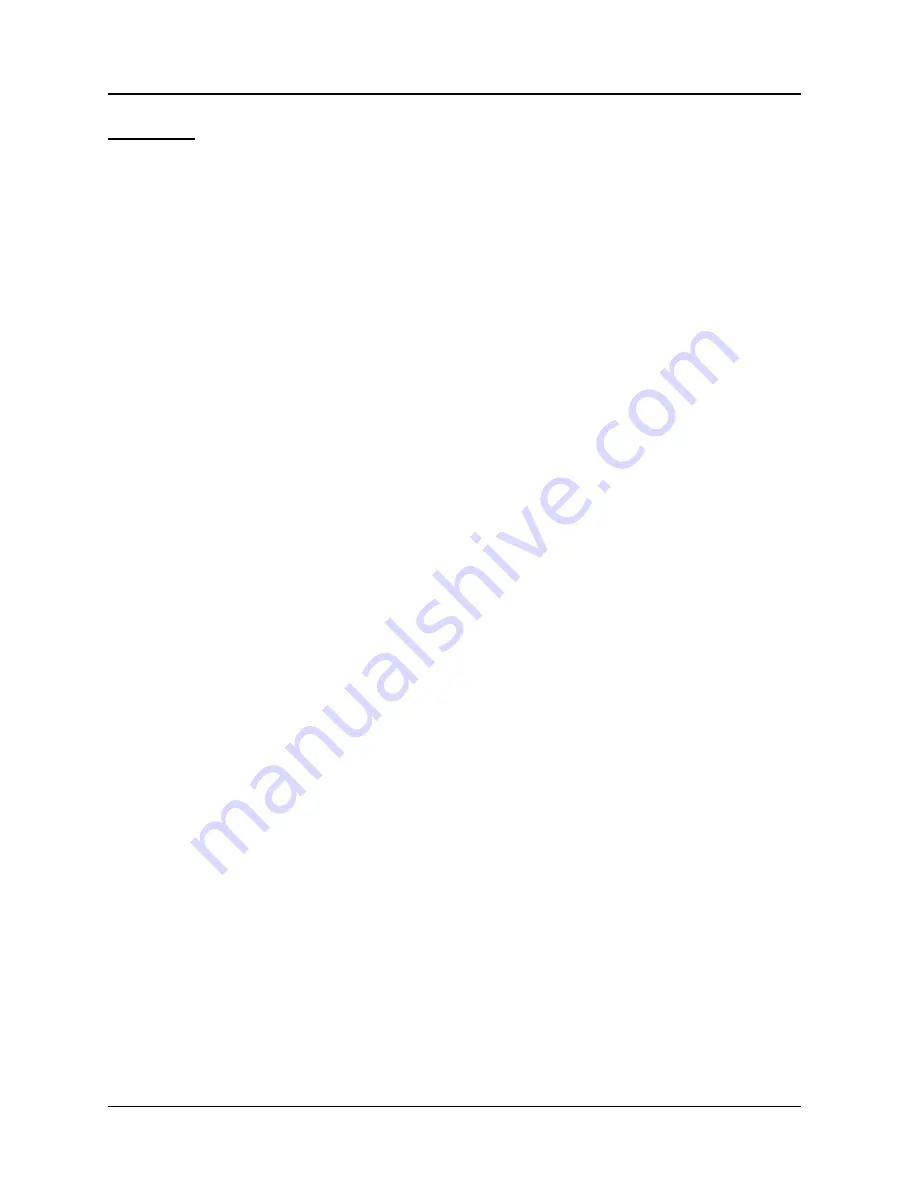
STEMBoT 2 User’s Manual
========================================================================
4.3.2.0 Text
Writing text to the LCD is done through the
print()
function of the
graphics()
module.
The
print()
function takes four parameters: the string to be written, the x location, the y
location, and the color. For ease of use, three additional functions are provided: printTop(),
printMiddle(), and printBottom(). These functions accept only two arguments, the string and
color, and print to the top of the LCD, the middle, and the bottom, respectively.
# This program is for demonstrating the operation of the LCD by
# printing "Hello, world!" in white text on a blue background.
import graphics
#imports the module used for LCD operations
import color
#imports the module for LCD colors
import uasyncio
#imports the module used for concurrent programming
bgColor=color.RGB(0,0,255)
#blue
textColor=color.RGB(255,255,255)
#white
async def main():
#main function header
graphics.paint(bgColor)
#fill screen with color
graphics.print("Hello, World!",40,120,textColor)
#print to LCD
Program 7:
Hello, world!
Text that has been written using the
print()
function can be erased using the
erase()
function. This function accepts four arguments as well: the number of characters to be erased,
the x location, the y location, and the color. The x and y locations should be the same as those
sent to the
print()
function. The color should be the background color on which the characters
have been written.
For an example using the
print()
and
erase()
functions, set up a serial connection to
the SB2, and type in the following lines:
import graphics
import color
red=color.RGB(255,0,0)
black=color.RGB(0,0,0)
graphics.paint(red)
graphics.print(“Hello, world!”,40,120,black)
Now, after making sure the text has shown up, send the following command to the SB2:
graphics.erase(13,40,120,red)
This line tells the SB2 how many characters there are in “Hello, world!” (be sure to
include spaces), the placement of the text, and the background color. The
erase()
function
works by drawing a filled rectangle (section 4.3.3.1) around the previously printed text. If a color
other than the background color is sent, the LCD will simply print a box of that color over the
text.
========================================================================
Revision 1.1.0 : January 2021
Summary of Contents for STEMBoT 2
Page 1: ...User s Manual Rev 1 1 0 ...