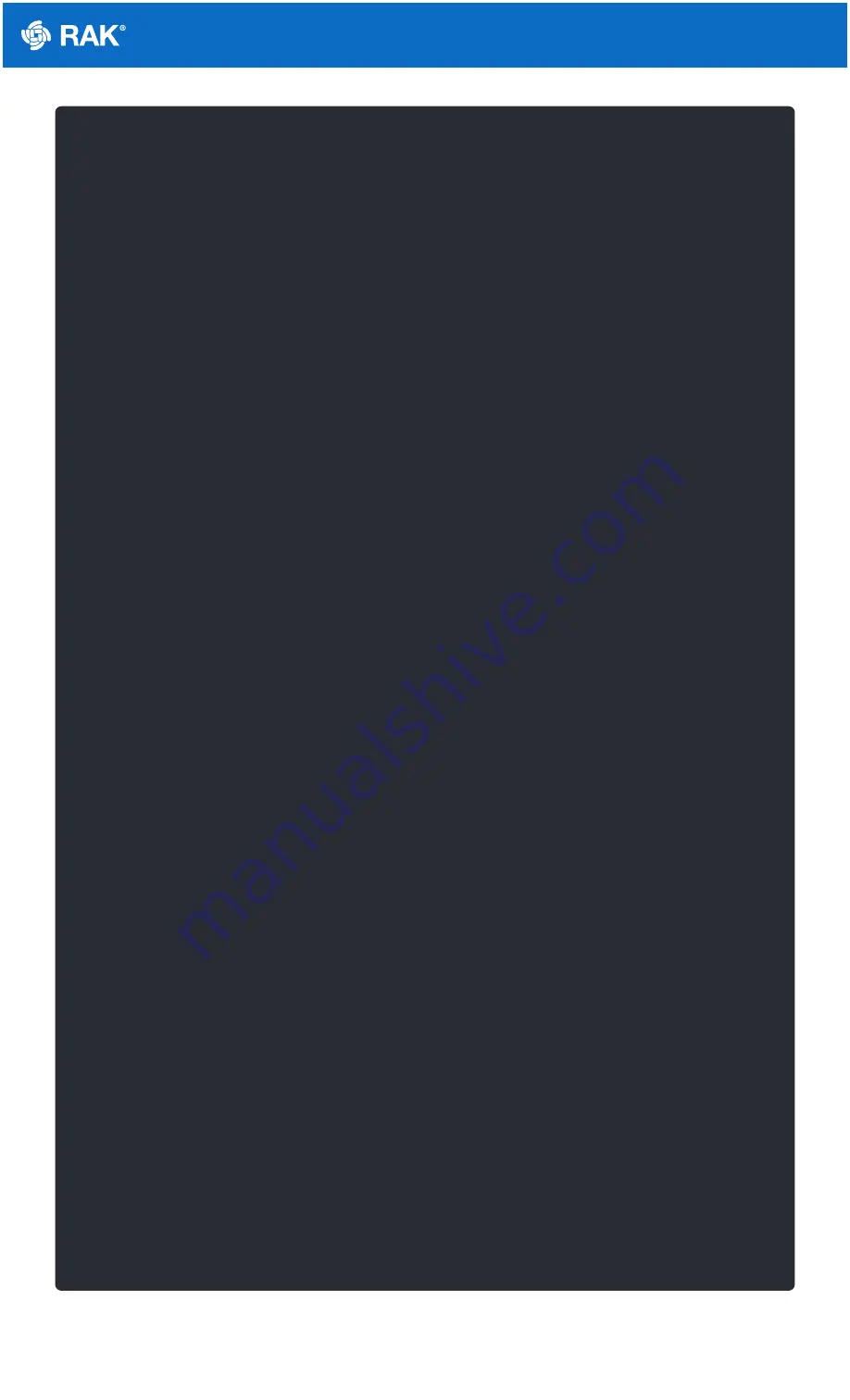
Documentation Center
7. Your device's I2C address should be displayed on the Serial Monitor, as shown in Figure 14.
/*
* Scan the I2C Address of your LCD using
* this example code. Make sure your SDA and SCL
* line is connected properly.
*
* Follow the connection of LCD with I2C Backpack to RAK13002.
*/
#
include
<Wire.h>
//include Wire.h library
void
setup
()
{
Wire
.
begin
();
// Wire communication begin
Serial
.
begin
(
9600
);
// The baudrate of Serial monitor is set in 9600
while
(
!
Serial
);
// Waiting for Serial Monitor
Serial
.
println
(
"\nI2C Scanner"
);
}
void
loop
()
{
byte error
,
address
;
//variable for error and I2C address
int
nDevices
;
Serial
.
println
(
"Scanning..."
);
nDevices
=
0
;
for
(
address
=
1
;
address
<
127
;
address
++
)
{
// The i2c_scanner uses the return value of
// the Wire.endTransmission to see if
// a device did acknowledge to the address.
Wire
.
beginTransmission
(
address
);
error
=
Wire
.
endTransmission
();
if
(
error
==
0
)
{
Serial
.
(
"I2C device found at address 0x"
);
if
(
address
<
16
)
Serial
.
(
"0"
);
Serial
.
(
address
,
HEX
);
Serial
.
println
(
" !"
);
nDevices
++
;
}
else
if
(
error
==
4
)
{
Serial
.
(
"Unknown error at address 0x"
);
if
(
address
<
16
)
Serial
.
(
"0"
);
Serial
.
println
(
address
,
HEX
);
}
}
if
(
nDevices
==
0
)
Serial
.
println
(
"No I2C devices found\n"
);
else
Serial
.
println
(
"done\n"
);
delay
(
5000
);
// wait 5 seconds for the next I2C scan
}
c