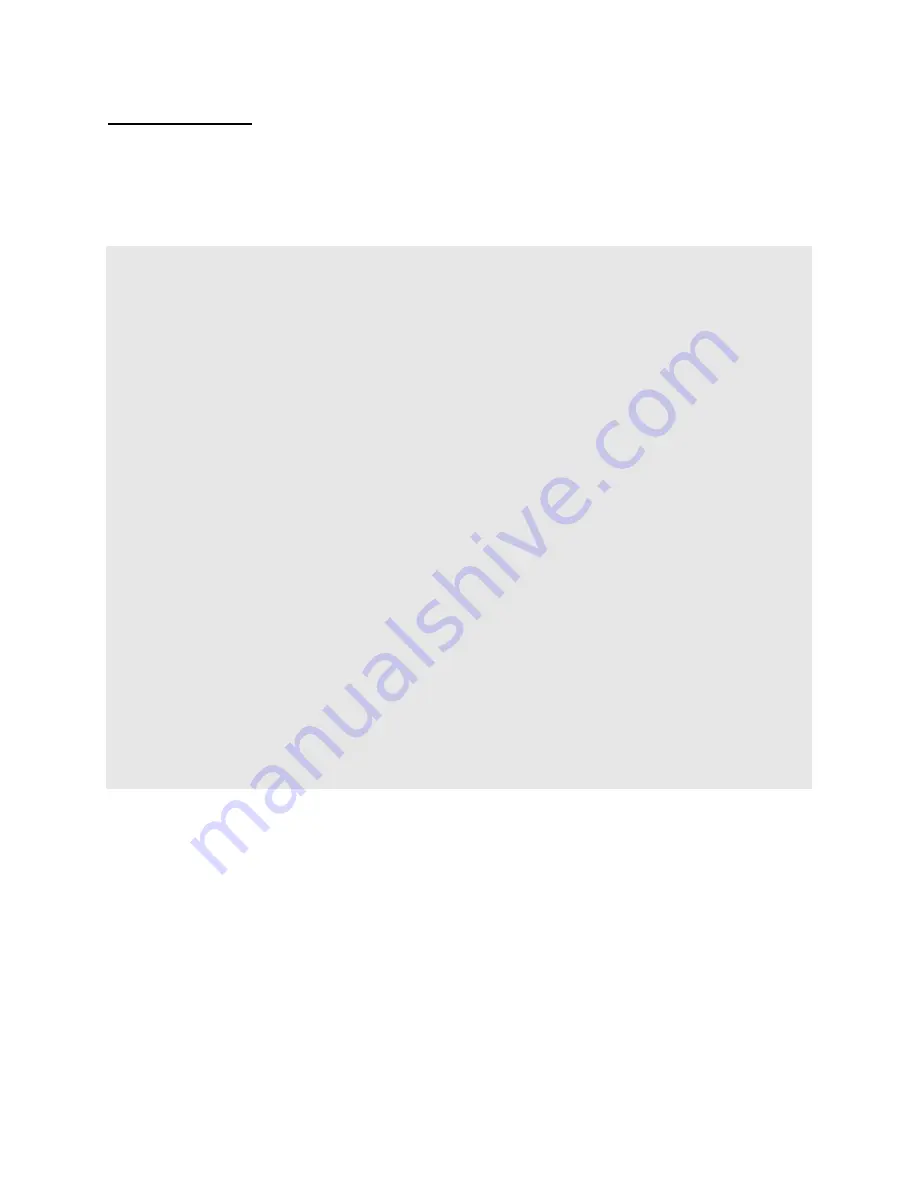
Parallax, Inc. • BASIC Stamp HomeWork Board ver 1.1
Page 17
Advanced LED Control
Another advantage to this program style is that we can cause the LED to follow the operation of a different value
within our program. We can do this because
Out15
is actually a bit-sized variable [a value that can change]. We
can copy another bit-sized variable to it and cause the LED to follow the value of that variable.
Listing 1d is a bit advanced, but you can handle it. Take a look and then we'll go into all the details.
' {$STAMP BS2}
' Program: LED Blink.BS2 (Version D)
' Purpose: Blinks an LED connected to the BASIC Stamp (advanced)
' ---------------------------------------------------------------
LedPin VAR Out15 ' LED on/off control
LedCtrl VAR Dir15 ' LED i/o pin control
blinkVal VAR Byte ' blink control value
blinkBit VAR Nib ' blink control bit
' ---------------------------------------------------------------
Initialize:
LedCtrl = %1 ' make LED pin an output
Start:
DEBUG CLS
FOR blinkBit = 0 TO 7 ' test all bits
FOR blinkVal = 0 TO 255 ' test all values
' report
DEBUG Home
DEBUG "Blink Value = ", DEC blinkVal, " ", CR
DEBUG "Blink Bit = ", DEC blinkBit, CR
DEBUG CR
DEBUG "Blink Value = ", BIN8 blinkVal, CR
DEBUG "Blink Bit = ", REP " "\(7 - blinkBit), "^ "
' control the LED
LedPin = blinkVal.LowBit(blinkBit)
PAUSE 5
NEXT
NEXT
GOTO Start
In this program we'll need a couple variables. The first is called
blinkVal
and it is defined as a Byte (8 bits). You'll
remember that a Byte can hold values from zero to 255. The other variable is called
blinkBit
and is defined as a
Nib (4 bits). Being a Nib,
blinkBit
can hold values from 0 to 15, but we only need it to go up to seven. We will use
blinkBit
to point at a bit in
blinkVal
and the LED will follow that bit value. It will be on when the bit is a one; off when
the bit is a zero.
The main part of the program starts by opening the
DEBUG
window and clearing it with
CLS
(clear screen). The
DEBUG
statement allows the BASIC Stamp send messages to the PC. This will let us know what's going on
inside our program as it's running.
The bulk of the code is two
FOR-NEXT
loops; one nested inside the other. The outside loop is controlled by
blinkBit
and will run eight times (0 to 7). For each iteration of
blinkBit
, the inner loop, controlled by
blinkVal
will run
256 times (0 to 255). This is where the action takes place.