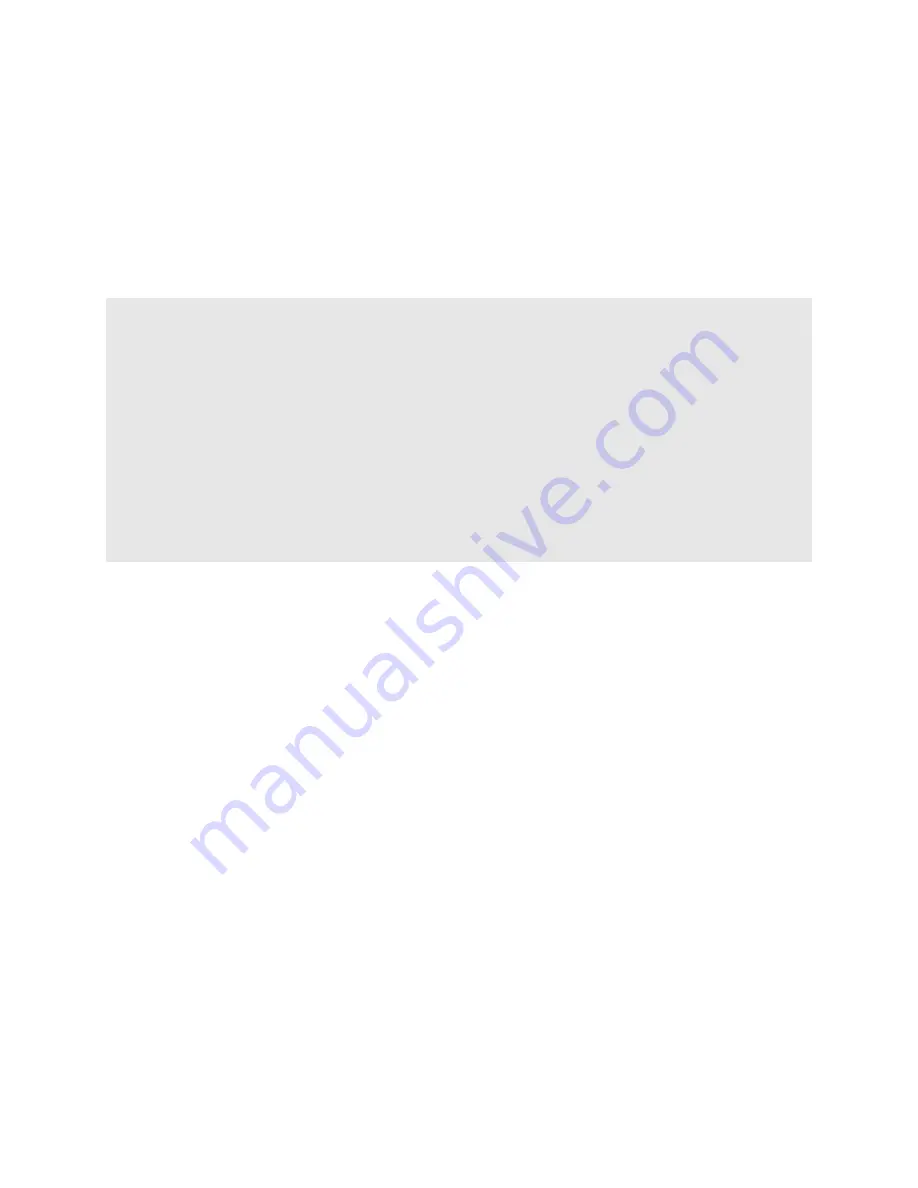
Parallax, Inc. • BASIC Stamp HomeWork Board ver 1.1
Page 15
Next, we make the LED control pin go to zero volts with
LOW
. With zero volts on both sides of the LED, no
current can flow and it turns off. Again, we use
PAUSE
to create a delay so that we can actually see that it is off.
Finally, we start the process over by jumping to the line labeled
Start
with the
GOTO
command.
Download and run the program – you should see the LED blink happily on and off. If you don't, double-check
your connections; especially the orientation of the LED. If it doesn't blink you may have it in backward.
Now, as exciting as that was, we can do more and write "better" code. Better code is code that not only works,
but is easy for us and others to understand. Let's improve our little program just a bit. Take a look at Listing 1b.
' {$STAMP BS2}
' Program: LED Blink.BS2 (Version B)
' Purpose: Blinks an LED connected to the BASIC Stamp
' ---------------------------------------------------------------
LedPin CON 15 ' LED control pin
' ---------------------------------------------------------------
Start:
HIGH LedPin ' turn LED on
PAUSE 500 ' wait one-half second
LOW LedPin ' turn LED off
PAUSE 500 ' wait one-half second
GOTO Start ' do it again
In this version we introduce constant (
CON
) values. Constants give us a way to assign a useful name to a value
that doesn't change. There a couple of good reasons for using constants in our program:
1. If we need to change the pin that controls the LED, we only have to change one place in the program.
Using constants prevents program errors by not catching all the changes required when circuit
connections are modified.
2. By using a constant, we now have more of an idea of what is being controlled.
HIGH 15
could be
controlling anything; a light, a bell, a heater. We're forced to explain the process of our program in
comments. By using the constant name
LedPin
, others reading our code know what is being controlled
and we can spend less energy explaining with comments.
Okay, so I'll bet you're wondering just how constants work.. They're actually used by the editor when the program
is being compiled to run on the BASIC Stamp. At the beginning of the compilation process the editor will replace
all the appearances of a constant name with its defined value. In our program,
HIGH LedPin
will be replaced with
HIGH 15
and the program will work just as in our first version. And don't worry, this replacement is an internal
process used by the compiler – our source code listing will not be changed.
Give it a try. You'll see that the program runs exactly the same as it did the first time. This time, though, our listing
makes a bit more sense. Ready for more?
One of the things that makes
HIGH
and
LOW
easy is that each command actually does two operations – that's
right, two. You see, to control a Stamp output pin we have to deal with two internal registers. The first is called
Dirs
. The
Dirs
register is 16 bits wide; one bit for each I/O pin. When a bit in the
Dirs
register is zero (default at
start-up), the associated I/O pin will be an input. When we make a
Dirs
bit a one, the associated I/O pin will be an
output.
The other register that we need to concern ourselves with is called
Outs
. Like
Dirs
,
Outs
is 16 bits wide. In this
case, the bits in
Outs
are connected to the I/O pins when the associated
Dirs
bits are set to one. When a bit in