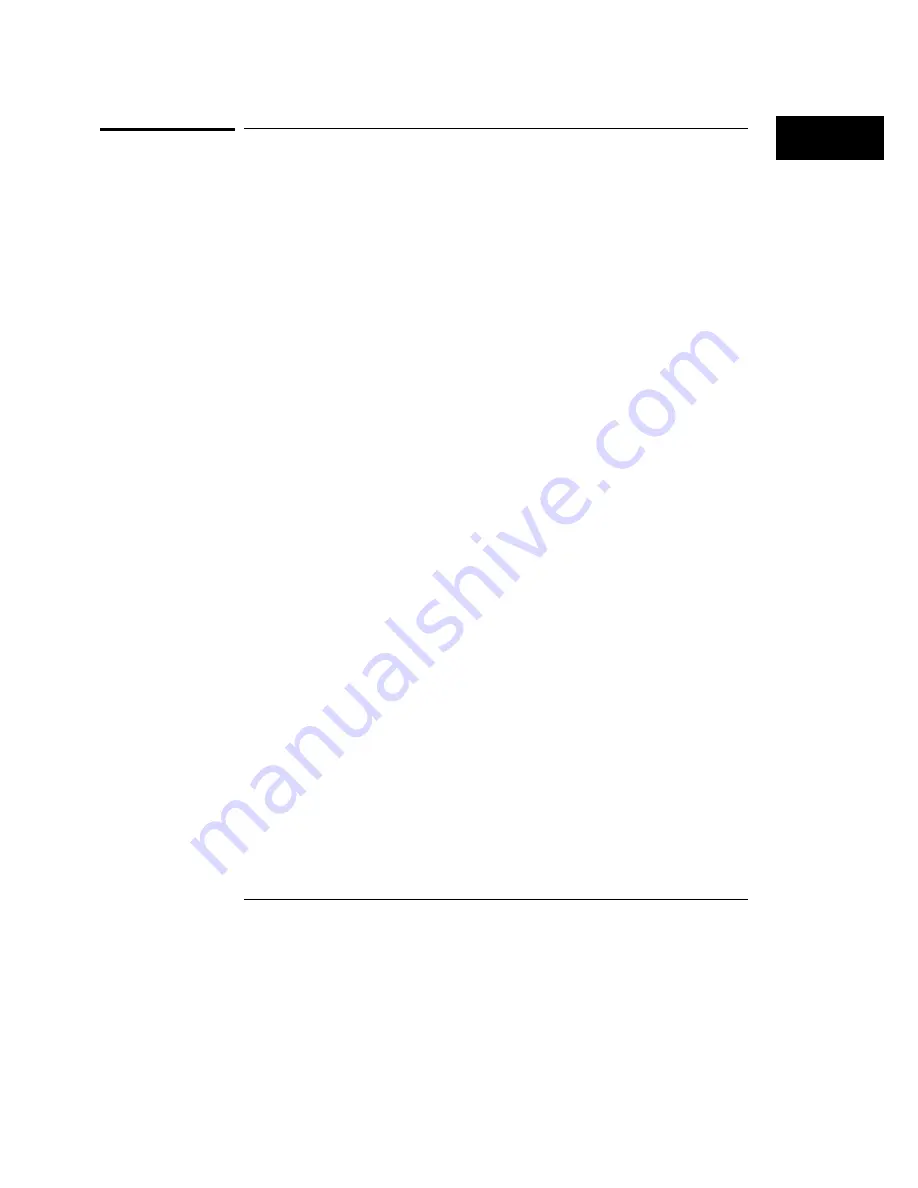
Example program
This C program demonstrates the basic command structure used to program
the logic analyzer, and how that command structure is embedded into the C
language.
/*HP Instrument C program using the HP-IB interface */
#include <stdio.h> /* Include file for printf, etc */
#include <stdlib.h> /* Include file for malloc, etc */
#include "CHPIB.H" /* HPIB Library constant declarations */
#include "CFUNC.H" /* HPIB library function prototypes */
/* Globals used throughout the program */
long isc;
long analyzer;
int error;
/* errorhandle - This function prints an error message of the screen */
/* regarding the command that caused the error. */
void errorhandle( char *function, char *cmdcause )
{
if ( error != NOERR )
{
printf("HPIB error in call to %s with %s, error = %s\n",
function, cmdcause, errstr(error) );
exit(1);
}
}
/* sendstringcmd - this function interfaces to the actual hpib output */
/* functions. It also checks for errors and calls the */
/* errorhandler function above */
void sendstringcmd( char *cmd)
{
error = IOOUTPUTS( analyzer, cmd, strlen(cmd) );
errorhandle("IOOUTPUTS", cmd);
}
int main()
{
isc = 7; /* Assign 7 to isc global */
analyzer = 707; /* Assign device address (707) to analyzer */
error = IORESET ( isc ); /* Reset the isc */
Programming Getting Started
Example program
25
Summary of Contents for 54620A
Page 6: ...6 ...
Page 9: ...1 Introduction to Programming ...
Page 21: ...2 Programming Getting Started ...
Page 35: ...3 Programming over HP IB ...
Page 40: ...40 ...
Page 41: ...4 Programming over RS 232 C ...
Page 48: ...48 ...
Page 49: ...5 Programming and Documentation Conventions ...
Page 53: ...Programming and Documentation Conventions The command tree 53 ...
Page 60: ...60 ...
Page 61: ...6 Status Reporting ...
Page 63: ...Status Reporting Data Structures Figure 4 Status Reporting 63 ...
Page 68: ...68 ...
Page 69: ...7 Installing and Using the Programmer s Reference ...
Page 76: ...76 ...