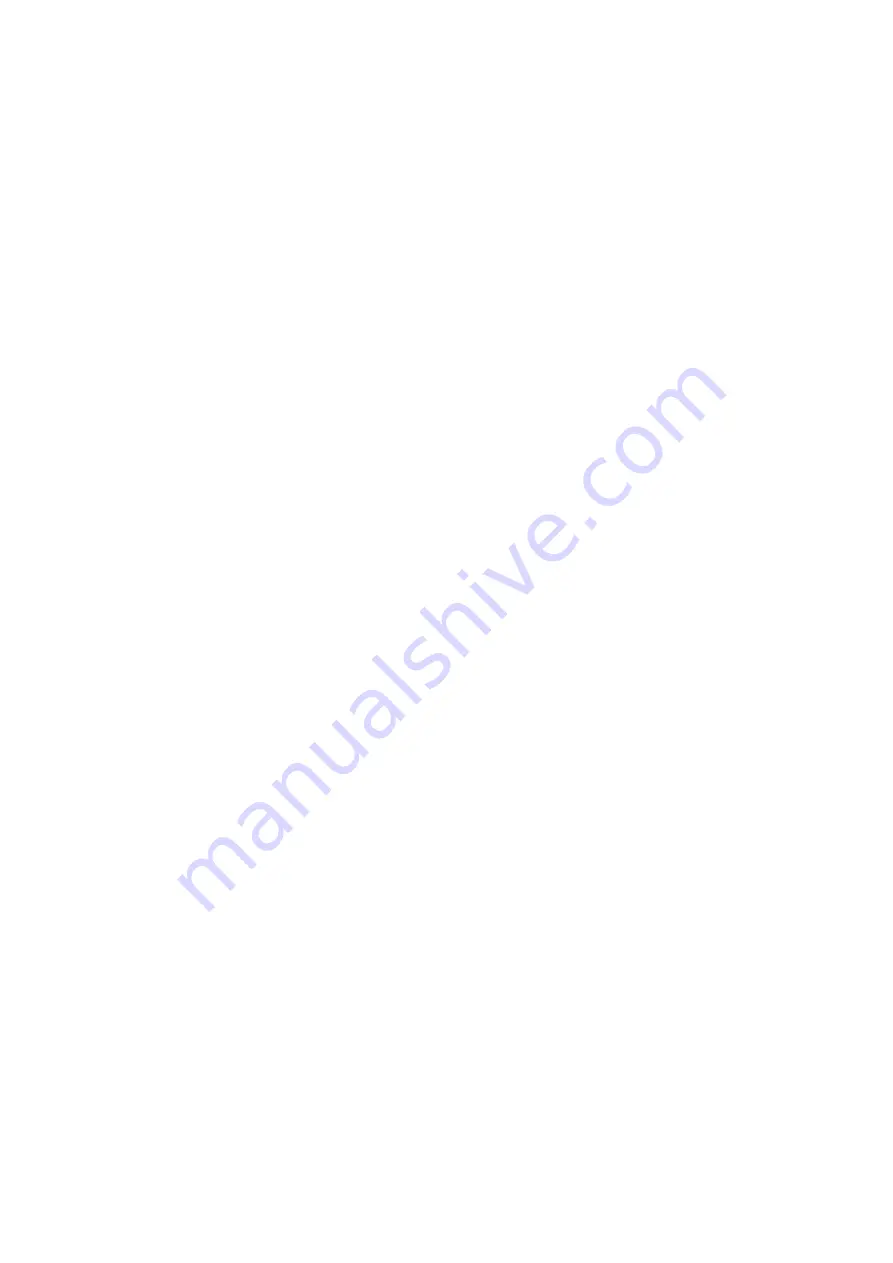
Code developed in Arduino 1.0.5, on a Fio classic board.
**Updated for Arduino 1.6.4 5/2015**
****************************************************************/
#include <SparkFunMiniMoto.h> // Include the MiniMoto library
// Create two MiniMoto instances, with different address settings.
MiniMoto motor0(0xC4); // A1 = 1, A0 = clear
MiniMoto motor1(0xC0); // A1 = 1, A0 = 1 (default)
#define FAULTn 16 // Pin used for fault detection.
// Nothing terribly special in the setup() function- prep the
// serial port, print a little greeting, and set up our fault
// pin as an input.
void setup()
{
Serial.begin(9600);
Serial.println("Hello, world!");
pinMode(FAULTn, INPUT);
}
// The loop() function just spins the motors one way, then the
// other, while constantly monitoring for any fault conditions
// to occur. If a fault does occur, it will be reported over
// the serial port, and then operation continues.
void loop()
{
Serial.println("Forward!");
motor0.drive(100);
motor1.drive(100);
delayUntil(1000);
Serial.println("Stop!");
motor0.stop();
motor1.stop();
delay(1000);
Serial.println("Reverse!");
motor0.drive(-100);
motor1.drive(-100);
delayUntil(1000);
Serial.println("Brake!");
motor0.brake();
motor1.brake();
delay(1000);
}
// delayUntil() is a little function to run the motor either for
// a designated time OR until a fault occurs. Note that this is
// a very simple demonstration; ideally, an interrupt would be
// used to service faults rather than blocking the application
// during motion and polling for faults.
void delayUntil(unsigned long elapsedTime)
{
// See the "BlinkWithoutDelay" example for more details on how
// and why this loop works the way it does.
unsigned long startTime = millis();
while (sta elapsedTime > millis())
{
// If FAULTn goes low, a fault condition *may* exist. To be
// sure, we'll need to check the FAULT bit.
if (digitalRead(FAULTn) == LOW)
{