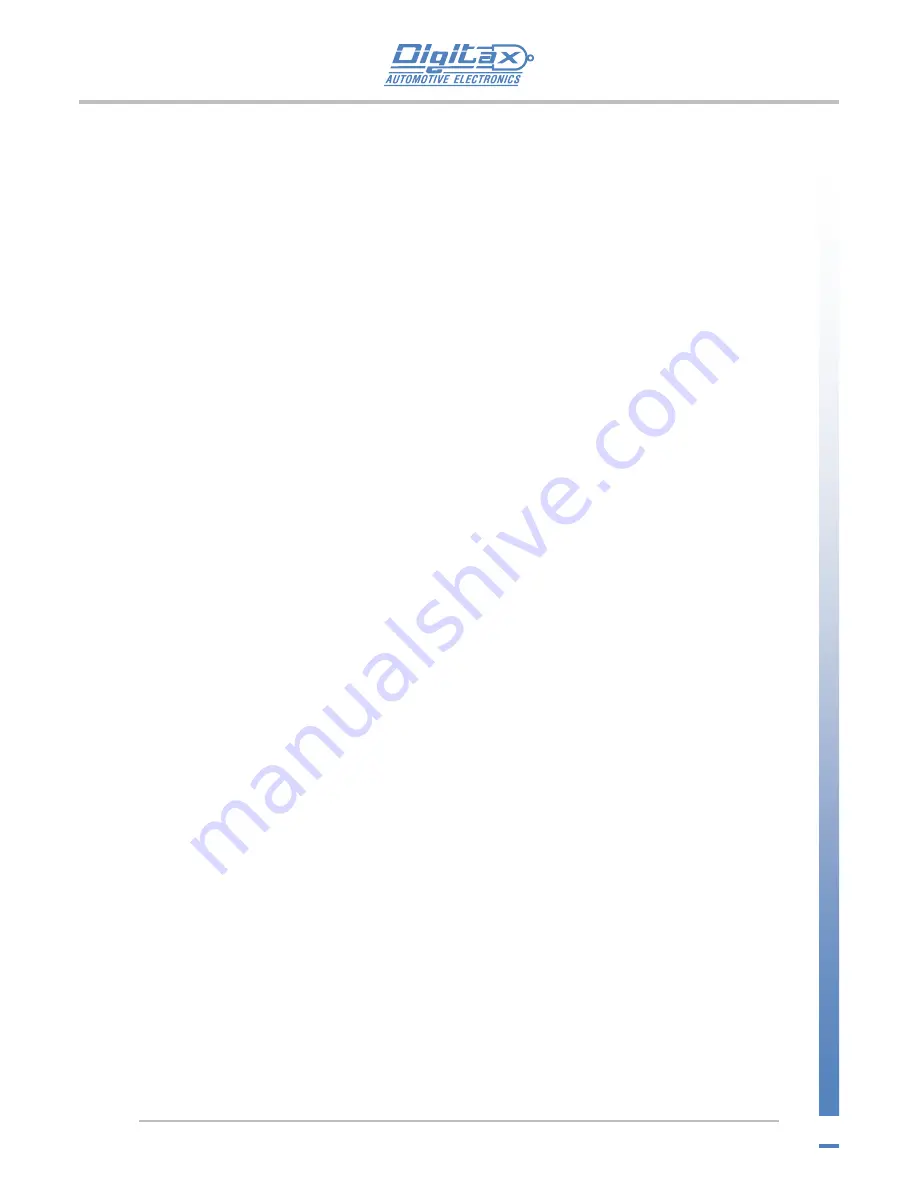
The information contained here are property of DIGITAX Automotive Electronics Italy, and extremely confidential. Any disclosure, copying, distribution to third
party is strictly prohibited.
51
6.
AITP (Light Dimmer)
This application shows how to use AITP to interact with the light dimmer. You can change display
brightness, retrieve light intensity, activate the automatic brightness regulation, etc…
Sample code:
using
System;
using
System.Collections.Generic;
using
System.ComponentModel;
using
System.Data;
using
System.Drawing;
using
System.Text;
using
System.Windows.Forms;
namespace
Digitax.Sample.Dimmer
{
//simple application to interact with the light dimmer
public
partial
class
DiimerSample
:
Form
{
//aitp instance
Aitp.Wrapper41.
AitpCore
_aitp =
new
Aitp.Wrapper41.
AitpCore
();
byte
_lightInt = 0x00;
byte
_dimmer = 0x00;
byte
[] _dimmTable =
new
byte
[8];
byte
_dimVal = (
byte
)20;
public
DiimerSample()
{
InitializeComponent();
this
.C=
new
CancelEventHandler
(DiimerSample_Closing);
//init aitp in MDT/MCU "COM4:" 19200 on ForceOne "COM3:" 38400
_aitp.AITPInit(
"COM3:"
, 38400);
_aitp.EvAITPDimmerValueAndTableT=
new
Aitp.Wrapper41.
AitpCore
.
delAITPActivateDimmerValueAndTableTrigger
(_aitp_EvAITPDimmerValueAndTableTrigger);
_aitp.EvAITPLightIntensityT=
new
Aitp.Wrapper41.
AitpCore
.
delAITPActivateLightIntensityTrigger
(_aitp_EvAITPLightIntensityTrigger);
//init light dimmer
_aitp.AITPInitLightDimmer();
tbDimVal.Text = _dimVal.ToString();
_aitp.AITPSetDimmerValue(_dimVal);
_aitp.AITPAskLightIntensity();
_aitp.AITPAskDimmerValueAndTable();
}
void
_aitp_EvAITPLightIntensityTrigger(
byte
LightIntensity,
uint
lparam)
{
_lightInt = LightIntensity;
UpdForm();
}
void
_aitp_EvAITPDimmerValueAndTableTrigger(
byte
DimmerValue,
byte
[] DimmerTable,
uint
lparam)
{
_dimmer = DimmerValue;
_dimmTable = DimmerTable;
UpdForm();
}
private
delegate
void
delUpdForm
();
private
void
UpdForm()
{
if
(InvokeRequired)
{
this
.BeginInvoke(
new
delUpdForm
(UpdForm));
return
;
}
//update text
tbDimmer.Text = _dimmer.ToString();
tbLight.Text = _lightInt.ToString();
StringBuilder
sb =
new
StringBuilder
();
foreach
(
byte
val
in
_dimmTable)
{
sb.Append(val);
sb.Append(
" "
);
}
tbTable.Text = sb.ToString();
}
private
void
btMinus_Click(
object
sender,
EventArgs
e)
{
//decrease light intesity
if
(_dimVal > 1 && !cbAuto.Checked)
{
_dimVal--;
tbDimVal.Text = _dimVal.ToString();
_aitp.AITPSetDimmerValue(_dimVal);
}
}
private
void
btPlus_Click(
object
sender,
EventArgs
e)
{