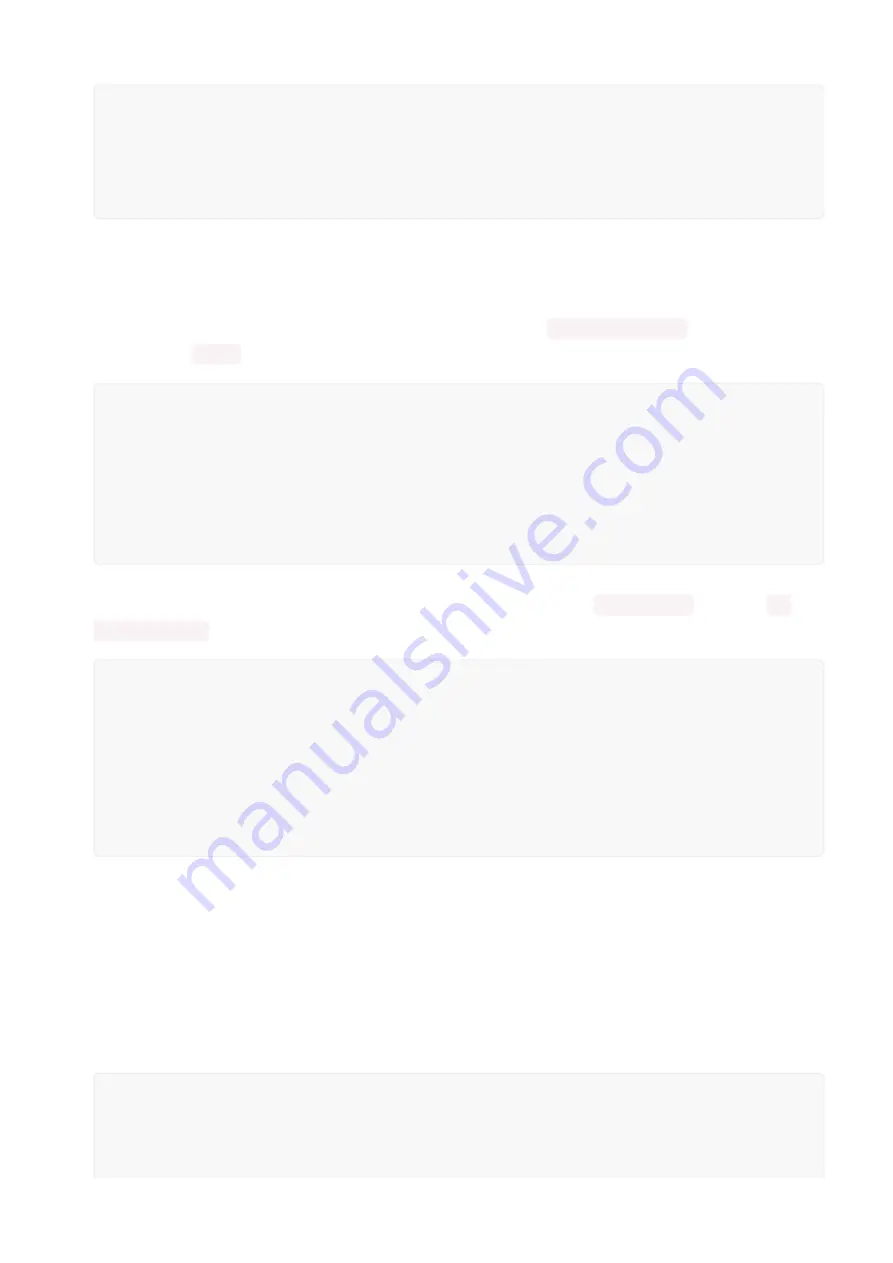
print("Fetching JSON data from %s"%JSON_GET_URL)
response = requests.get(JSON_GET_URL)
print('-'*40)
print("JSON Response: ", response.json())
print('-'*40)
response.close()
HTTP POST with Requests
Requests can also POST data to a server by calling the
requests.post
method,
passing it a
data
value.
data = '31F'
print("POSTing data to {0}: {1}".format(JSON_POST_URL, data))
response = requests.post(JSON_POST_URL, data=data)
print('-'*40)
json_resp = response.json()
# Parse out the 'data' key from json_resp dict.
print("Data received from server:", json_resp['data'])
print('-'*40)
response.close()
You can also post json-formatted data to a server by passing
json_data
into the
re
quests.post
method.
json_data = {"Date" : "July 25, 2019"}
print("POSTing data to {0}: {1}".format(JSON_POST_URL, json_data))
response = requests.post(JSON_POST_URL, json=json_data)
print('-'*40)
json_resp = response.json()
# Parse out the 'json' key from json_resp dict.
print("JSON Data received from server:", json_resp['json'])
print('-'*40)
response.close()
Advanced Requests Usage
Want to send custom HTTP headers, parse the response as raw bytes, or handle a
response's http status code in your CircuitPython code?
We've written an example to show advanced usage of the requests module below.
# SPDX-FileCopyrightText: 2021 ladyada for Adafruit Industries
# SPDX-License-Identifier: MIT
import
board
import
busio
©Adafruit Industries
Page 23 of 54