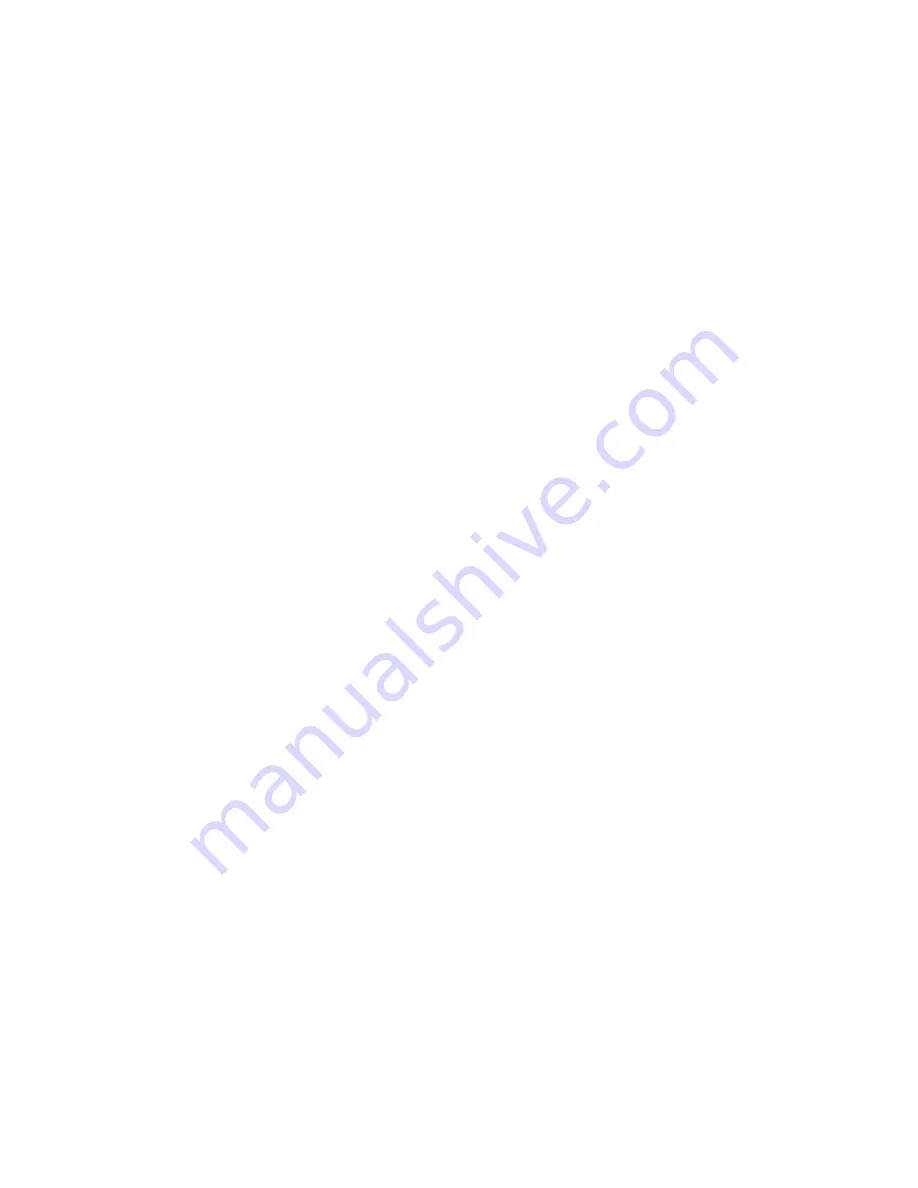
while (XBee.available() < 4)
; // Wait for pin and three value numbers to be received
char pin = XBee.read(); // Read in the pin number
int value = ASCIItoInt(XBee.read()) * 100; // Convert next three
value += ASCIItoInt(XBee.read()) * 10; // chars to a 3-digit
value += ASCIItoInt(XBee.read()); // number.
value = constrain(value, 0, 255); // Constrain that number.
// Print a message to let the control know of our intentions:
XBee.print("Setting pin ");
XBee.print(pin);
XBee.print(" to ");
XBee.println(value);
pin = ASCIItoInt(pin); // Convert ASCCI to a 0-13 value
pinMode(pin, OUTPUT); // Set pin as an OUTPUT
analogWrite(pin, value); // Write pin accordingly
}
// Read Digital Pin
// Send 'r' or 'R' to enter
// Then send a digital pin # to be read
// The Arduino will print the digital reading of the pin to XBee.
void readDPin()
{
while (XBee.available() < 1)
; // Wait for pin # to be available.
char pin = XBee.read(); // Read in the pin value
// Print beggining of message
XBee.print("Pin ");
XBee.print(pin);
pin = ASCIItoInt(pin); // Convert pin to 0-13 value
pinMode(pin, INPUT); // Set as input
// Print the rest of the message:
XBee.print(" = ");
XBee.println(digitalRead(pin));
}
// Read Analog Pin
// Send 'a' or 'A' to enter
// Then send an analog pin # to be read.
// The Arduino will print the analog reading of the pin to XBee.
void readAPin()
{
while (XBee.available() < 1)
; // Wait for pin # to be available
char pin = XBee.read(); // read in the pin value
// Print beginning of message
XBee.print("Pin A");
XBee.print(pin);
pin = ASCIItoInt(pin); // Convert pin to 0-6 value
// Printthe rest of the message:
Page 19 of 24