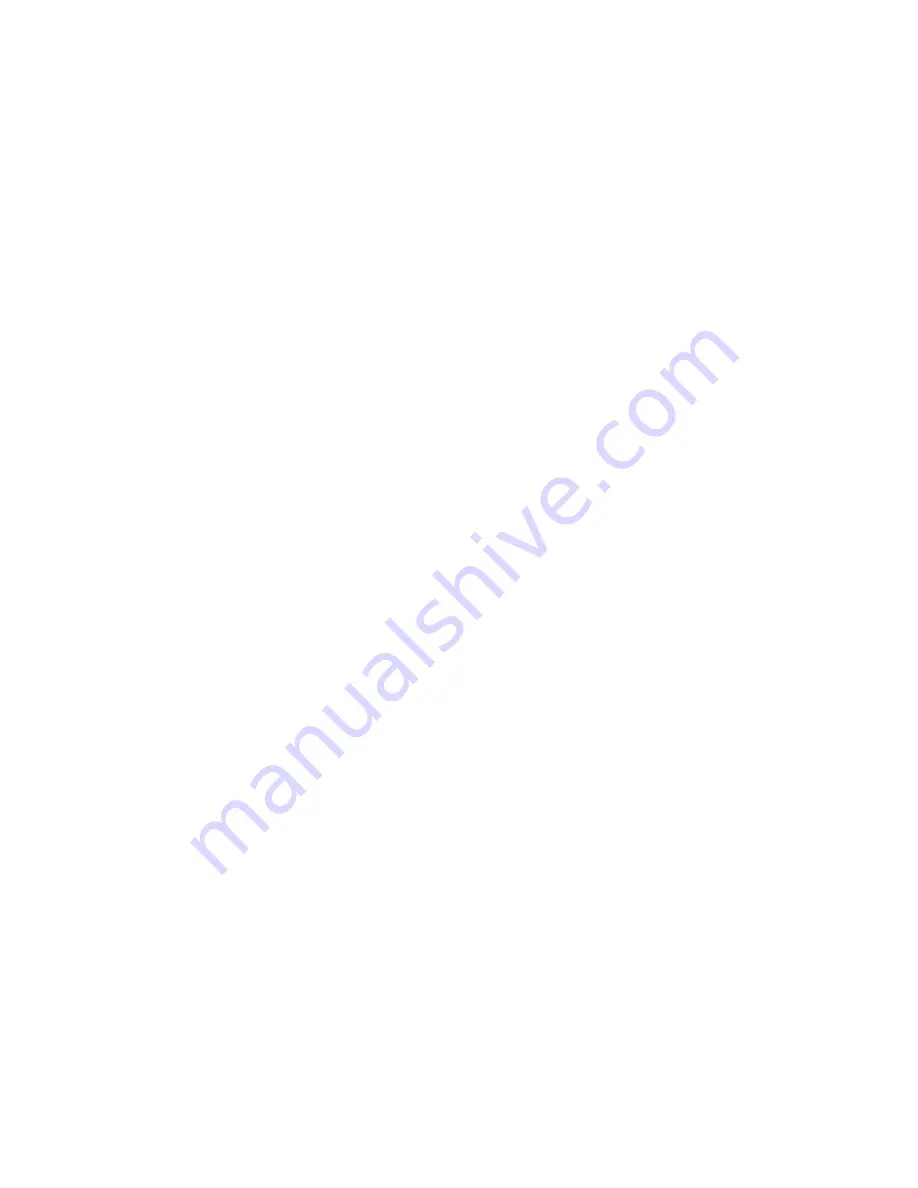
switch (c)
{
case 'w': // If received 'w'
case 'W': // or 'W'
writeAPin(); // Write analog pin
break;
case 'd': // If received 'd'
case 'D': // or 'D'
writeDPin(); // Write digital pin
break;
case 'r': // If received 'r'
case 'R': // or 'R'
readDPin(); // Read digital pin
break;
case 'a': // If received 'a'
case 'A': // or 'A'
readAPin(); // Read analog pin
break;
}
}
}
// Write Digital Pin
// Send a 'd' or 'D' to enter.
// Then send a pin #
// Use numbers for 0-9, and hex (a, b, c, or d) for 10-13
// Then send a value for high or low
// Use h, H, or 1 for HIGH. Use l, L, or 0 for LOW
void writeDPin()
{
while (XBee.available() < 2)
; // Wait for pin and value to become available
char pin = XBee.read();
char hl = ASCIItoHL(XBee.read());
// Print a message to let the control know of our intentions:
XBee.print("Setting pin ");
XBee.print(pin);
XBee.print(" to ");
XBee.println(hl ? "HIGH" : "LOW");
pin = ASCIItoInt(pin); // Convert ASCCI to a 0-13 value
pinMode(pin, OUTPUT); // Set pin as an OUTPUT
digitalWrite(pin, hl); // Write pin accordingly
}
// Write Analog Pin
// Send 'w' or 'W' to enter
// Then send a pin #
// Use numbers for 0-9, and hex (a, b, c, or d) for 10-13
// (it's not smart enough (but it could be) to error on
// a non-analog output pin)
// Then send a 3-digit analog value.
// Must send all 3 digits, so use leading zeros if necessary.
void writeAPin()
{
Page 18 of 24