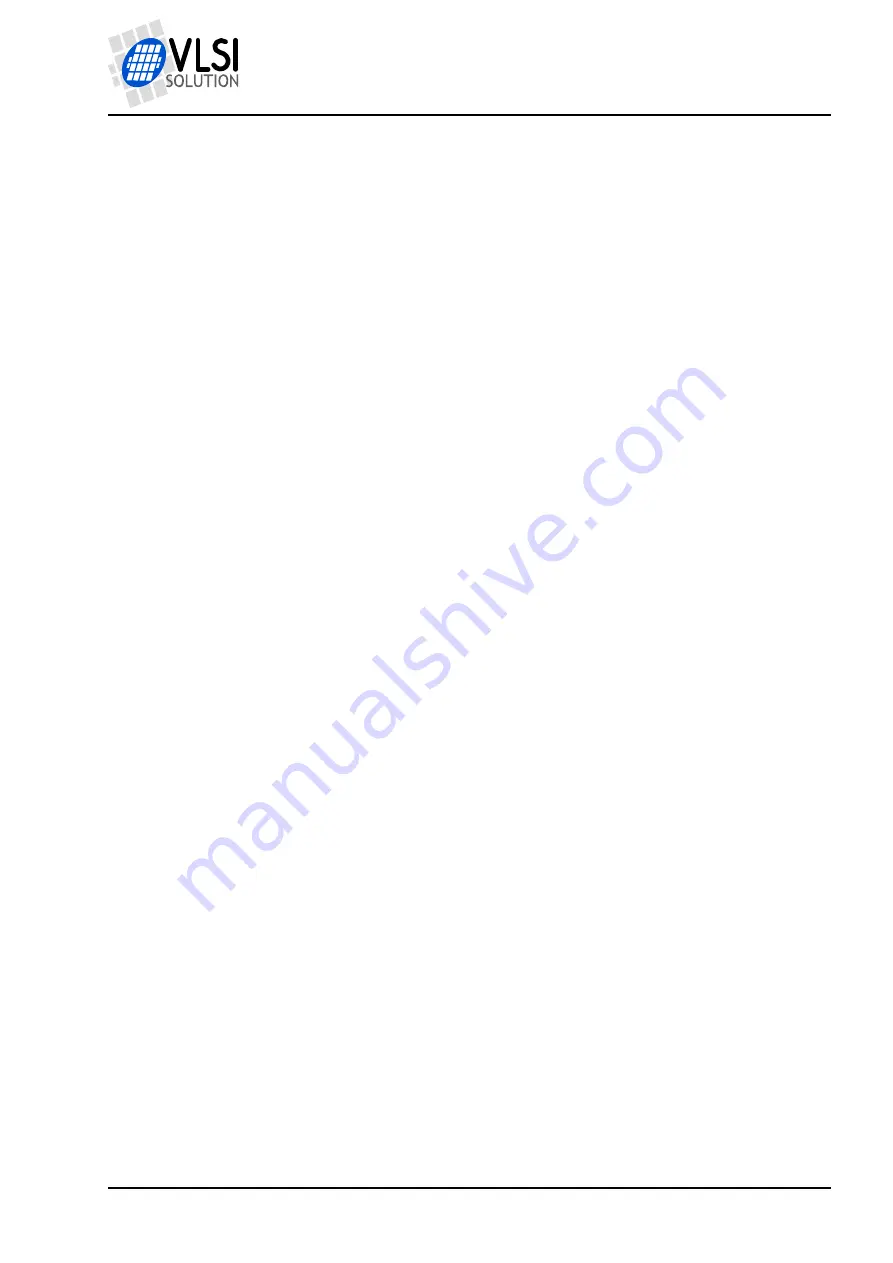
PRELIMINARY
VS1063a Prog. Guide
4
INTERFACING WITH VS1063A USING A MICROCONTROLLER
4
Interfacing with VS1063a Using a Microcontroller
This chapter explaings how to interface with the VS1063a using SCI and SDI buses, which
both are special cases of the SPI bus. This document will show how to access the buses using
microcontroller software written in C language. For details on bus signals, see the VS1063a
Datasheet Chapter “SPI Buses”.
To be able to do SCI and SDI operations, let’s first define a basic one-byte SPI transfer:
#define CONFIGURE_AS_OUTPUT(p){/* Do what's needed*/}
#define CONFIGURE_AS_INPUT(p){/* Do what's needed*/}
#define OUT_SET_HIGH(p){p = 1;}
#define OUT_SET_LOW(p){p = 0;}
#define IN_IS_HIGH(p)(p)
// Software SPI port: Shift 8 bits in and out using a software SPI port
unsigned char SpiTransfer(unsigned char outdata) {
unsigned char b=0;
unsigned char indata=0;
for (b=0; b<8; b++) {
if (outdata & 0x80) { // Leftmost bit first
OUT_SET_HIGH(PIN_MOSI);
} else {
OUT_SET_LOW(PIN_MOSI);
}
outdata <<= 1;
// Shift outdata bits left
OUT_SET_HIGH(PIN_SCK); // Clock high
indata <<= 1;
// Shift indata bits left
if (IN_IS_HIGH(PIN_MISO)) {
indata |= 1;
// Set rightmost bit high
}
OUT_SET_LOW(PIN_SCK); // Clock low
}
return indata;
// Return the result byte to caller
}
4.1
The SCI (SPI) Bus
The SCI (SPI) bus is a control and command bus. Every operation consists of four bytes:
instruction, address, and two data bytes. Instruction and address bytes are always offered by
the microcontroller. If instruction is WriteCommand (2), then also data bytes come from the
microcontroller. If instruction is ReadCommand (3), then data bytes come from VS1063a.
Version: 0.40, 2011-09-02
7