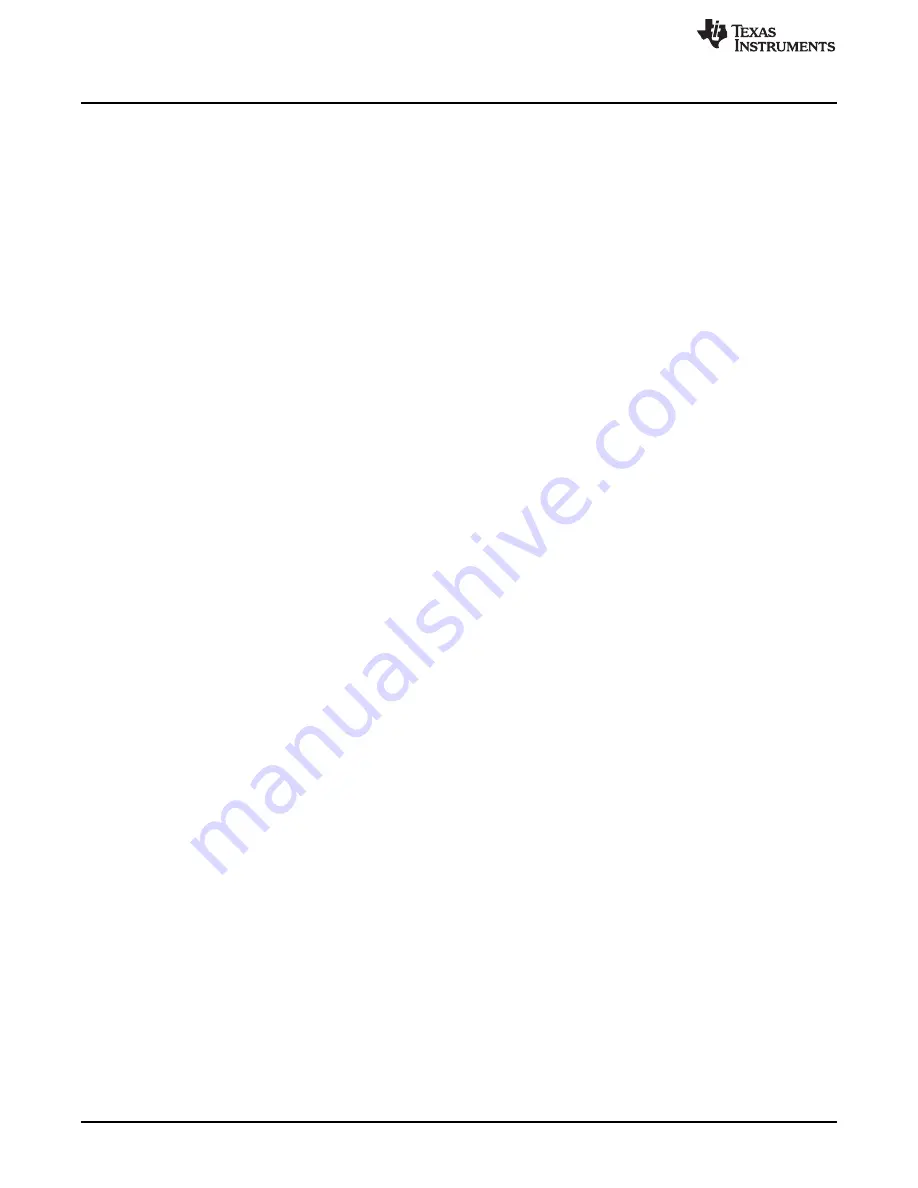
Host HTTP Requests Processing
158
SWRU455A – February 2017 – Revised March 2017
Copyright © 2017, Texas Instruments Incorporated
HTTP Server
The following code demonstrates how to implement an HTTP GET handler that sends a response (a short
text string) immediately. The code assumes that the macro slcb_NetAppRequestHdlr is mapped to
NetAppRequestHandler in file user.h.
#define RESPONSE_TEXT "Example text to be displayed in browser"
void NetAppRequestHandler(
SlNetAppRequest_t *pNetAppRequest,
SlNetAppResponse_t *pNetAppResponse)
{
char *contentType =
"text/html"
;
unsigned char *pMetadata;
unsigned char *pResponseText;
pMetadata = (unsigned char*)malloc(128);
pResponseText = (unsigned char*)malloc(
sizeof
(RESPONSE_TEXT));
if
((
NULL
== pMetadata) || (
NULL
== pResponseText))
{
/* Allocation error */
}
memcpy(pResponseText, RESPONSE_TEXT,
sizeof
(RESPONSE_TEXT));
switch
(pNetAppRequest->Type)
{
case
SL_NETAPP_REQUEST_HTTP_GET:
{
pNetAppResponse->Status = SL_NETAPP_HTTP_RESPONSE_200_OK;
/* Write the content type TLV to buffer */
pNetAppResponse->ResponseData.pMetadata = pMetadata;
*pMetadata =
(_u8) SL_NETAPP_REQUEST_METADATA_TYPE_HTTP_CONTENT_TYPE;
pM+;
*(_u16 *)pMetadata = (_u16) strlen (contentType);
pM=2;
memcpy (pMetadata, contentType, strlen(contentType));
pM=strlen(contentType);
/* Write the content length TLV to buffer */
*pMetadata = SL_NETAPP_REQUEST_METADATA_TYPE_HTTP_CONTENT_LEN;
pM+;
*(_u16 *)pMetadata = 2;
pM=2;
*(_u16 *) pMetadata = (_u16)
sizeof
(RESPONSE_TEXT);
pM=2;
/* Calculate and write the total length of meta data */
pNetAppResponse->ResponseData.MetadataLen =
pMetadata - pNetAppResponse->ResponseData.pMetadata;
/* Write the text of the response */
pNetAppResponse->ResponseData.PayloadLen =
sizeof
(RESPONSE_TEXT);
pNetAppResponse->ResponseData.pPayload = pResponseText;
pNetAppResponse->ResponseData.Flags = 0;
}
break
;
default
:
/* POST/PUT/DELETE requests will reach here. */
break
;
}
}
The following code demonstrates how to implement HTTP GET handler that delegates the request to
some other application. The user must extract any relevant information from the request and save it as the
data buffers are freed when the handler returns.
void NetAppRequestHandler(
SlNetAppRequest_t *pNetAppRequest,
SlNetAppResponse_t *pNetAppResponse)
{
switch
(pNetAppRequest->Type)
{
case
SL_NETAPP_REQUEST_HTTP_GET: