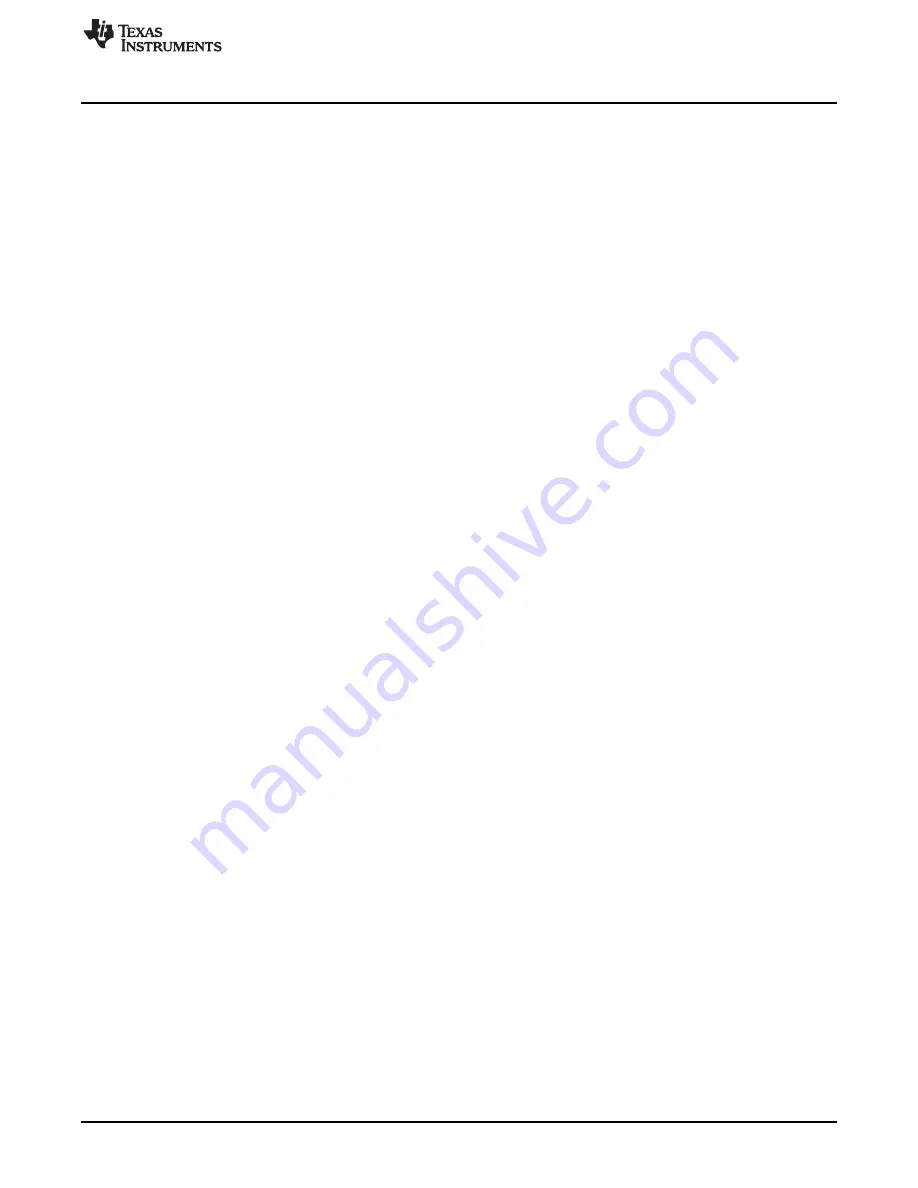
Read a File
119
SWRU455A – February 2017 – Revised March 2017
Copyright © 2017, Texas Instruments Incorporated
File System
Example:
_i32
FileHdl;
_i32
Status;
_u32
Offset = 0;
unsigned char
pData[100];
_u32
Len = 0;
Status = sl_FsRead( FileHdl, Offset, pData, Len );
if
( Status < 0 )
{
/*error */
/* abort */
Status = sl_FsClose(FileHdl,0,'A',1);
}
8.5.3 Close an Opened (for Read) File
When the read is completed, the host must close the file. Closing the file releases the file resources from
the device memory.
An abort file command can be invoked without using the file handle; the reference can be found in the file
commit-rollback function.
An abort file command open for read has the same functionality as the close function. In case of an
unexpected or sudden shutdown, each file opened for read that is not closed is treated as if it is aborted.
The following is a partial list of errors that might be returned by the close after read function:
•
SL_ERROR_FS_INVALID_HANDLE: The input file handle is illegal.
Close file example:
_i32
FileHdl;
_i16
Status;
const
_u32
SignatureLen;
_u8*
pSignature, pCeritificateFileName;
pCeritificateFileName = 0;
pSignature = 0;
SignatureLen = 0;
Status = sl_FsClose(FileHdl,pCeritificateFileName,pSignature,SignatureLen);
if
( Status < 0 )
{
/* error */
/* abort */
sl_FsClose(FileHdl,0,'A',1);
}
Abort file example:
i32
FileHdl;
_i16
Status;
const
_u8
Signature;
const
_u32
SignatureLen;
_u8*
pCeritificateFileName;
pCeritificateFileName = 0;
Signature = 'A';
SignatureLen = 1;
Status = sl_FsClose(FileHdl,pCeritificateFileName,Signature,SignatureLen);
if
( Status < 0 )
{
/*error */
}
8.6
Delete a File
Deleting of a file removes its storage from the file system and updates the files allocation table. Deleting is
done by the host function sl_FsDel().