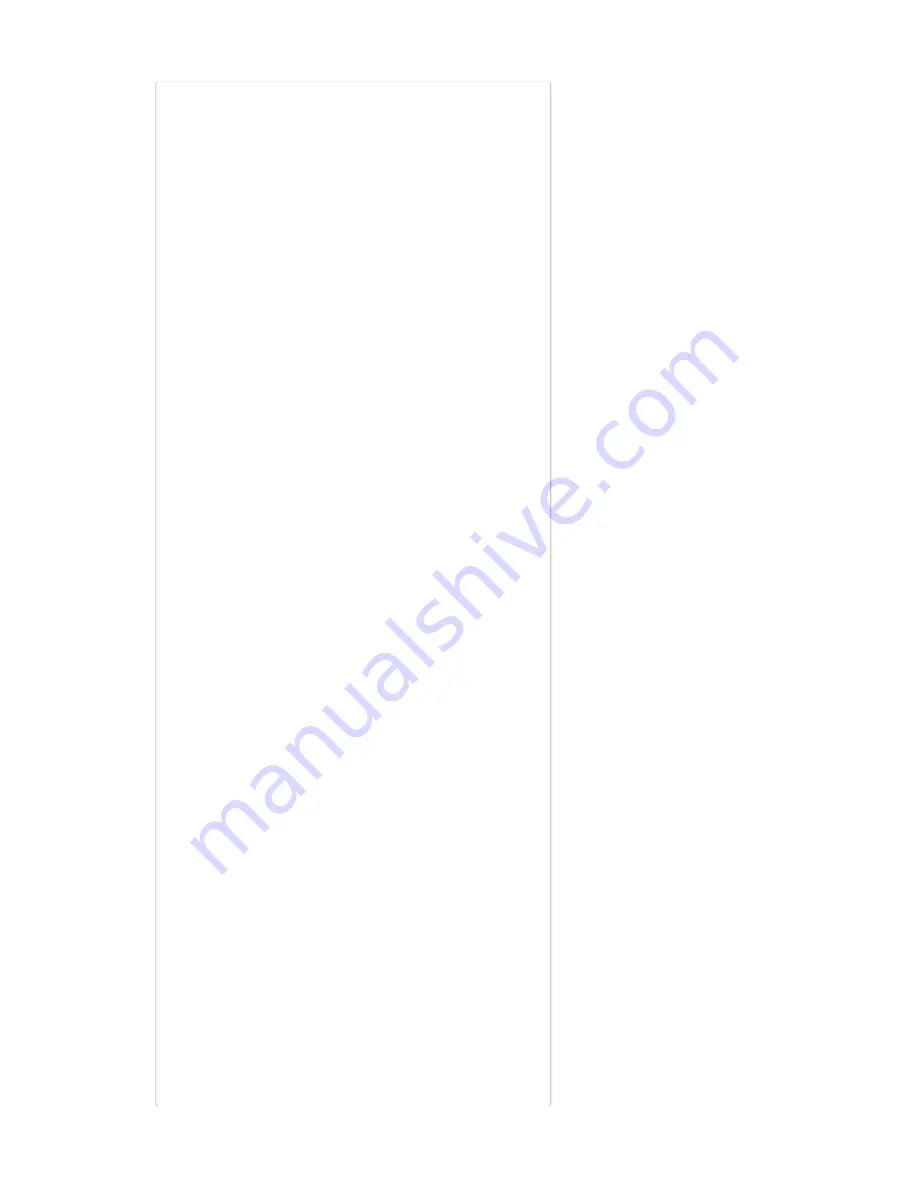
driveArdumoto(MOTOR_B,
REVERSE,
255);
//
Set
motor
B
to
REVE
RSE
at
max
delay(1000);
//
Motor
B
will
spin
as
set
for
1
second
driveArdumoto(MOTOR_B,
FORWARD,
127);
//
Set
motor
B
to
FOR
WARD
at
half
delay(1000);
//
Motor
B
will
keep
trucking
for
1
second
stopArdumoto(MOTOR_B);
//
STOP
motor
B
//
Drive
both
driveArdumoto(MOTOR_A,
FORWARD,
255);
//
Motor
A
at
max
spe
ed.
driveArdumoto(MOTOR_B,
FORWARD,
255);
//
Motor
B
at
max
spe
ed.
delay(1000);
//
Drive
forward
for
a
second
//
Now
go
backwards
at
half
that
speed:
driveArdumoto(MOTOR_A,
REVERSE,
127);
//
Motor
A
at
max
spe
ed.
driveArdumoto(MOTOR_B,
REVERSE,
127);
//
Motor
B
at
max
spe
ed.
delay(1000);
//
Drive
forward
for
a
second
//
Now
spin
in
place!
driveArdumoto(MOTOR_A,
FORWARD,
255);
//
Motor
A
at
max
spe
ed.
driveArdumoto(MOTOR_B,
REVERSE,
255);
//
Motor
B
at
max
spe
ed.
delay(2000);
//
Drive
forward
for
a
second
stopArdumoto(MOTOR_A);
//
STOP
motor
A
stopArdumoto(MOTOR_B);
//
STOP
motor
B
}
//
driveArdumoto
drives
'motor'
in
'dir'
direction
at
'spd'
sp
eed
void driveArdumoto(byte
motor,
byte
dir,
byte
spd)
{
if (motor
== MOTOR_A)
{
digitalWrite(DIRA,
dir);
analogWrite(PWMA,
spd);
}
else if (motor
== MOTOR_B)
{
digitalWrite(DIRB,
dir);
analogWrite(PWMB,
spd);
}
}
//
stopArdumoto
makes
a
motor
stop
void stopArdumoto(byte
motor)
{
driveArdumoto(motor,
0,
0);
}
//
setupArdumoto
initialize
all
pins
void setupArdumoto()
{
//
All
pins
should
be
setup
as
outputs:
pinMode(PWMA,
OUTPUT);
pinMode(PWMB,
OUTPUT);
pinMode(DIRA,
OUTPUT);
pinMode(DIRB,
OUTPUT);
//
Initialize
all
pins
as
low:
digitalWrite(PWMA,
LOW);
Page 13 of 17