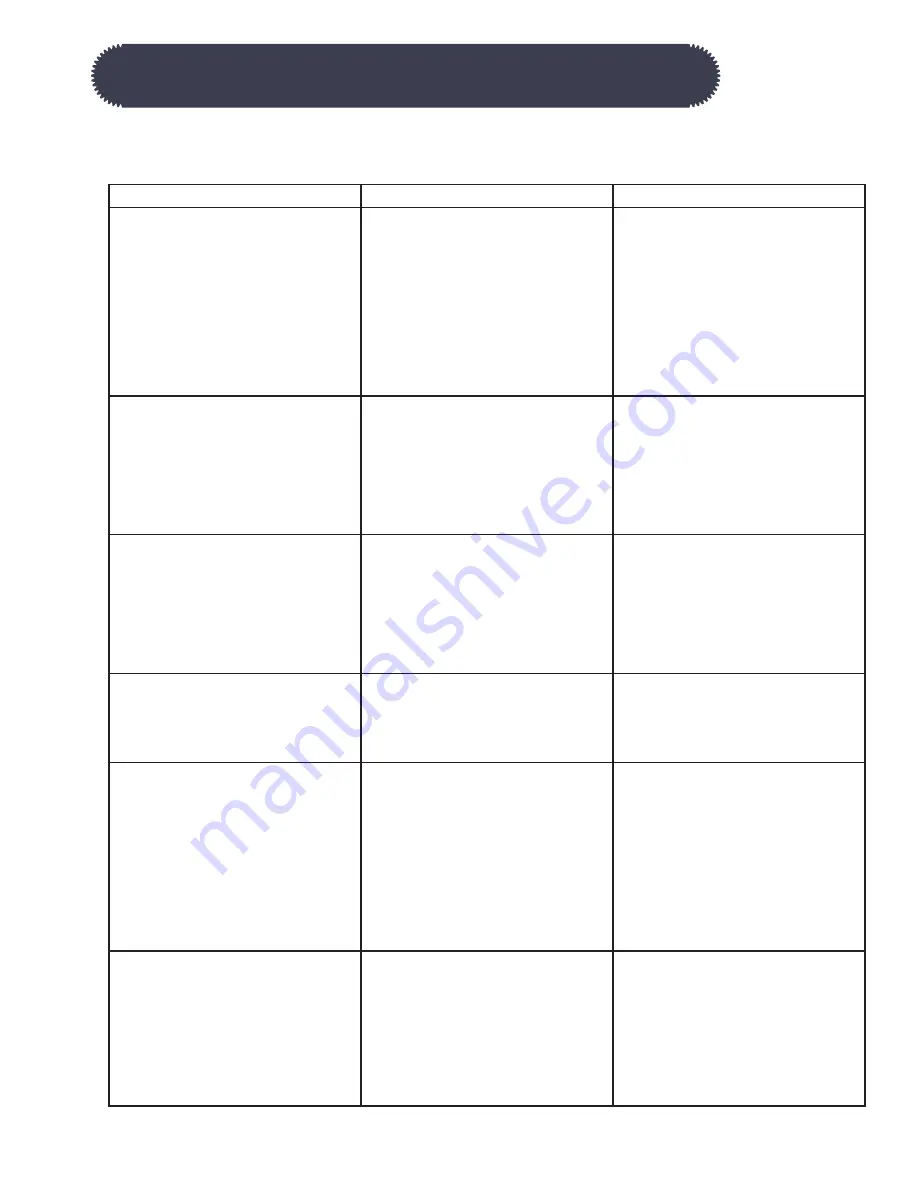
TETRIX Tele-Op Control Module Arduino Library Functions Chart
for the SONY DUALSHOCK 4 Gaming Controller
The Tele-Op module ships with firmware for the PS4 gaming controller installed. Please be sure to download and install
the latest version of the Tele-Op Arduino libraries at
Pitsco.com/TETRIX-Tele-Op-Control-Module#downloads
for the
most up-to-date programming features and functionality.
Description
Function
Coding Example
Get PS4 Controller Data
Reads the status of all analog and
digital buttons and joysticks from a
PS4 game controller connected to the
Tele-Op module.
Be sure to call this function as
frequently as possible in your Arduino
code. Each time it is called, the data
from the PS4 gaming controller is
refreshed.
ps4.
getPS4
();
Data Returned: None
void
loop
() {
ps4.
getPS4
();
}
Check Connection
Checks to see if the PS4 gaming
controller has been successfully
connected to the Tele-Op module.
ps4.
Connected
;
Data Returned:
0 = PS4 has disconnected
1 = PS4 has connected
void
loop
() {
ps4.
getPS4
();
if (ps4.
Connected
) {
// do this if connected
} else {
// do this if not connected
}
}
Check Range
Checks to see if the PS4 gaming
controller Bluetooth signal is in range
of the Tele-Op module.
ps4.
inRange
;
Data Returned:
0 = PS4 is out of range
1 = PS4 is in range
void
loop
() {
ps4.
getPS4
();
if (ps4.
inRange
) {
// do this if in range
} else {
// do this if not in range
}
}
Reset Tele-Op Module
Force resets the module; equivalent to
physically pressing the red Stop/Reset
button.
ps4.
resetTeleOp
();
Data Returned: None
ps4.
resetTeleOp
();
Note:
Call this function
once
in code
if there is a need to reset the Tele-Op
module.
Read Digital Button Status
Returns the status of a PS4 controller
digital button.
Digital button function parameters
are:
L1, L2, L3
R1, R2, R3
UP, DOWN, RIGHT, LEFT
TRIANGLE, CROSS, CIRCLE, SQUARE
SHARE, OPTIONS, POWER, TOUCH
ps4.
Button
(
L1
);
Data Returned:
0 = Button not pressed
1 = Button pressed
void
loop
() {
ps4.
getPS4
();
if (ps4.
Button
(
L1
)) {
// do this if pressed
} else {
// do this if not pressed
}
}
Read Analog Button Status
Returns the status of a PS4 controller
analog trigger button.
Analog buttons are the L2 and R2
trigger buttons.
Analog button parameters are:
L2T, R2T
ps4.
Button
(
L2T
);
or
ps4.
Button
(
R2T
);
Data Returned: Integer
Data Range: 0-255
void
loop
() {
ps4.
getPS4
();
int x = ps4.
Button
(
L2T
);
}
The analog value (0-255) of the L2
trigger button is stored in variable
x
.