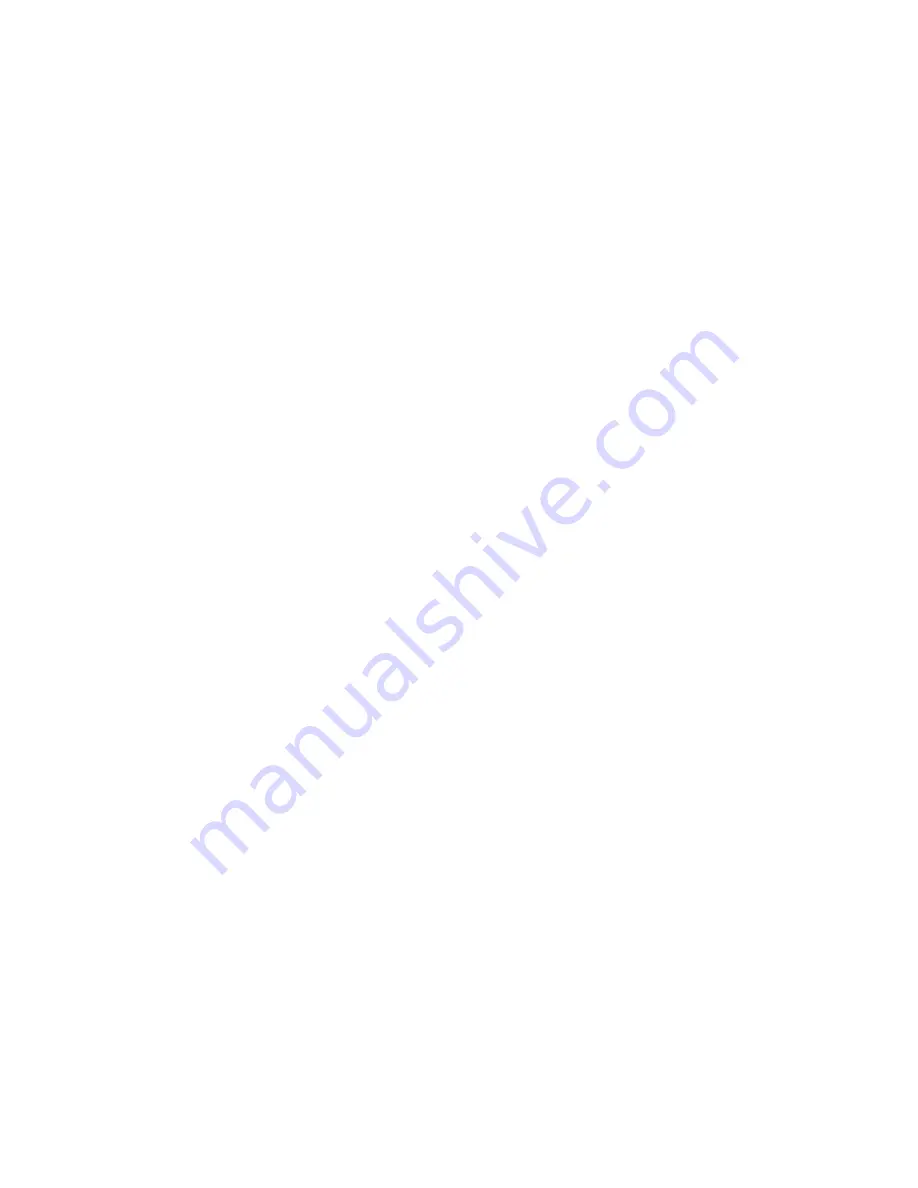
Section Five
Programming the GPIB-LPT
© National Instruments Corporation
5-7
GPIB-LPT User Manual
Using the GPIB-LPT with C and BASIC
The following examples show how to access the parallel port in the C and BASIC programming
languages, respectively.
Accessing the Parallel Port in C
#include <stdio.h>
.
.
FILE *fopen(),
*fp;
char *gpib_dev=
"LPT2";
fp=fopen(gpib_dev,"w");
fprintf(fp, "Hello, world");
fflush(fp);
These lines send the string
"Hello, world"
to the GPIB device connected to the GPIB-LPT.
Accessing the Parallel Port in BASIC
10
OPEN "LPT2" FOR OUTPUT AS #1
20
PRINT #1, "Hello, world"
This program sends the string
"Hello, world"
to the GPIB device. The BASIC commands
LPRINT
and
LLIST
always send data to the first parallel printer adapter in the system (
LPT1
);
these will only send data to the GPIB device if the GPIB-LPT is
LPT1
.
Writing a GPIB-LPT Driver
When you use an IBM BIOS driver, it performs the following steps to send a character out to the
parallel port:
1. Writes the character to the DATA Register.
2. Waits for the signal BUSY* to equal 1; if the timeout period expires, exits the routine. If the
timeout period does not expire, continues the routine.
3. Sets the signal STROBE equal to 1.
4. Waits 1 to 5
µ
sec.
5. Sets the signal STROBE equal to 0.
6. Returns from the routine with the status information from the STAT Register.
Although the IBM BIOS driver is sufficient, a higher performance driver can be implemented by
taking advantage of the GPIB-LPT's interrupt capability. Writing a GPIB-LPT driver to send a
character out to the parallel port requires two parts: a setup and initialization routine, and an
interrupt handler routine.