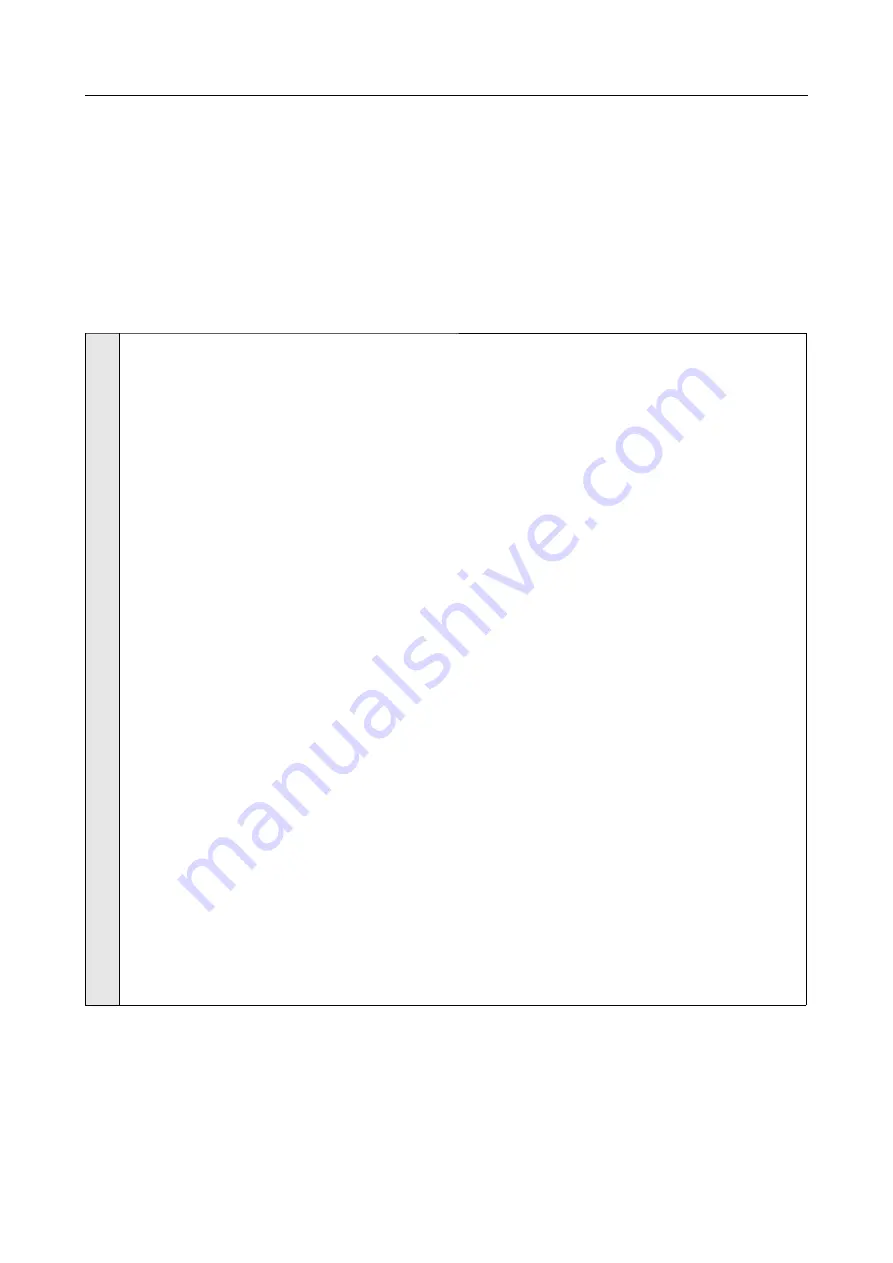
RP6 ROBOT SYSTEM - 4. Programming the RP6
RP6Library offers an universal module for general purpose usage.
Stopwatches allow you to handle a number of tasks “simultaneously” – at least this is
what you will see from your point of view outside of the microcontroller.
The RP6 provides eight 16bit Stopwatches (Stopwatch1 to Stopwatch8), which may be
started, stopped, set and read. As for the mSleep function we have chosen a resolu-
tion of one millisecond, which implies each of these timers will increment its counter in
intervals of 1ms. This method is not useable for very critical timing, as checking the
counter levels may not meet strict accuracy requirements.
The following example demonstrates the usage of the Stopwatches:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
#include "RP6RobotBaseLib.h"
int
main
(
void
)
{
initRobotBase
();
// Initialize the micro-controller
writeString_P
(
"\nRP6 Demo Program for Stopwatches\n"
);
writeString_P
(
"__________________________________\n\n"
);
startStopwatch1
();
// Start Stopwatch1
startStopwatch2
();
// Start Stopwatch2
uint8_t
counter =
0
;
uint8_t
runningLight
=
1
;
// Main loop:
while
(
true
)
{
// A small LED running light:
if
(
getStopwatch1
()
>
100
)
// Did 100ms (= 0.1s) pass by?
{
setLEDs
(
runningLight
);
// Set the LEDs
runningLight
<<=
1
;
// Next LED (shift operation)
if
(
runningLight
>
32
)
// Last LED?
runningLight
=
1
;
// Yes, restart with LED1!
setStopwatch1
(
0
);
// Reset Stopwatch1 to zero
}
// Output a counter level in the terminal:
if
(
getStopwatch2
()
>
1000
)
// Did 1000ms (= 1s) pass by?
{
writeString_P
(
"CNT:"
);
writeInteger
(
counter
,
DEC
);
// Output counter level
writeChar
(
'\n'
);
counter
++;
// Increment the counter
setStopwatch2
(
0
);
// Reset Stopwatch2 to zero
}
}
return
0
;
}
The program is quite simple. Every second, it outputs the counter level via the serial
interface and increments the counter (lines 29 up to 36). At the same time we ex-
ecute a simple running light with the LEDs (lines 19 up to 26), with a refresh interval
of 100ms.
- 84 -