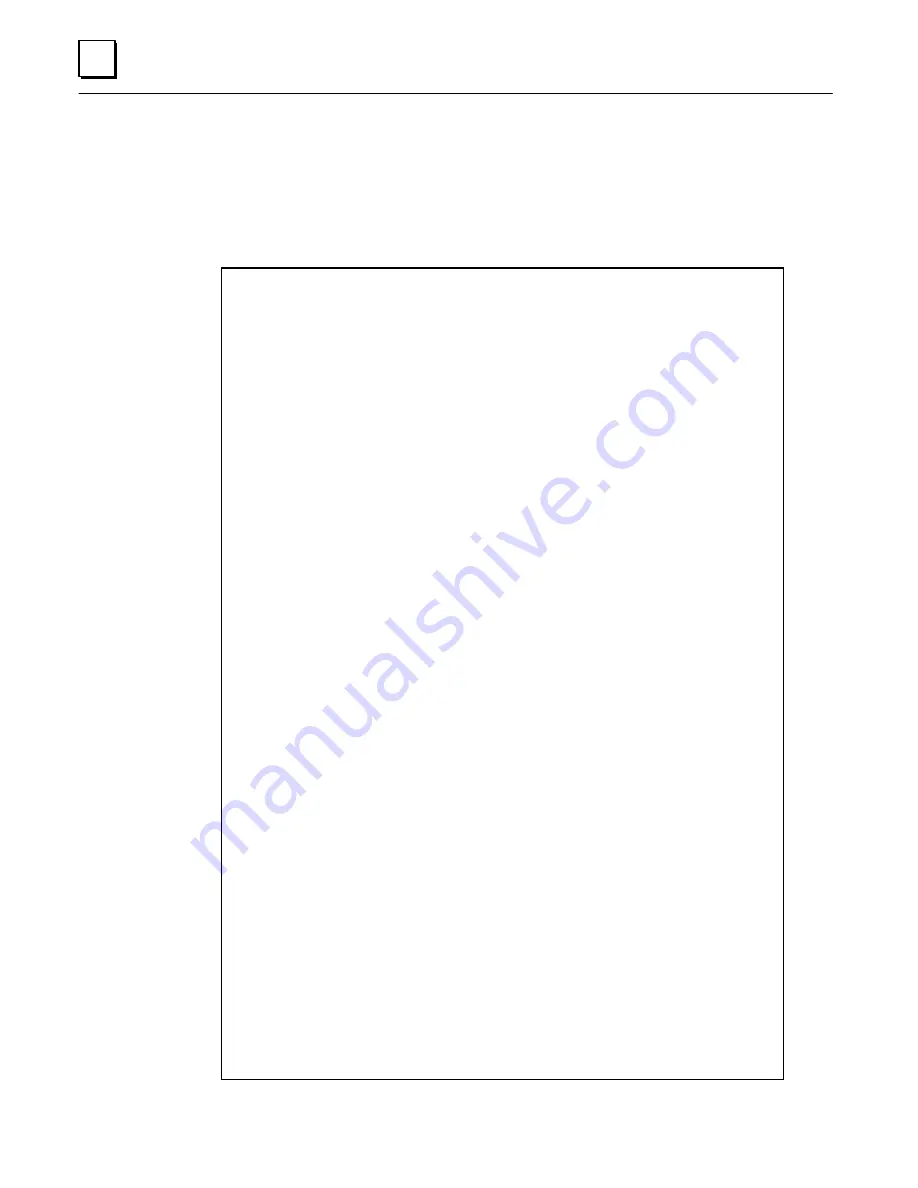
A
A-2
VME DLAN/DLAN+ Interface Module User’s Manual - August 1995
GFK-1044A
The
main
function in DLANTEST.C is quite simple. First, it tests the count of command line
arguments. If there are enough arguments to hold the short and standard address values, the
second and third argument strings are converted to unsigned integers, and the resulting values
replace the default addresses. Then,
main
prints the address values to the standard output
device and calls
vme_setup
to do the actual initialization of the target module. Finally, a
non-terminating loop initializes the target module and then monitors its status.
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
/*
* dlantest.c
*
* Program for testing vme_setup() in a VME DLAN module.
*/
#include <vtos.h>
#include <stdio.h>
#include <stdlib.h>
#include
”
dlaninit.h
”
/*
* Default values that may be replaced by command line arguments.
*/
#define SHORT_START_ADDR
0x1800
#define STD_START_ADDR 0x00020000L
/*
* Run from the PCM command interpreter prompt like this:
*
* > R DLANTEST.EXE 1800 20000
*
* where the two numeric arguments are the short non
–
privileged and
* standart non
–
privileged start addresses, respectively, where a
* target VME DLAN module will be accessed.
*/
void main( int argc, char far* far argv[] )
{
int status, last_status = SETUP_CMPLT;
unsigned short short_start_addr = SHORT_START_ADDR;
unsigned long std_start_addr = STD_START_ADDR;
if (argc > 2) {
short_start_addr = (unsigned short )(atol( argv[1] ) & 0x0000ffffL);
std_start_addr = atol( argv[2] ) & 0x00ffffffL;
}
printf(
”
short non
–
priv start addr = %04x, std non
–
priv start addr = %08lx\n
”
,
short_start_addr,
std_start_addr );
for (;;) {
status = vme_status( std_start_addr );
/*
* If the target module is uninitialized or its status has changed:
* Print the current status value.
*/
if (status != SETUP_CMPLT || status != last_status) {
printf(
”
status of vme module at base address %08lx = %d\n
”
,
std_start_addr,
status );
/*
* If the module is uninitialized:
* Attempt to initialize it.
* Print the result.
*/
if (status != SETUP_CMPLT) {
status = vme_setup( short_start_addr, std_start_addr );
printf(
”
new vme_setup() result = %d\n\n
”
,
status );
}
}
/*
* Save the current status value and wait 1,000 milliseconds.
*/
last_status = status;
Wait_time( MS_COUNT_MODE, 0, 1000 );
}
}