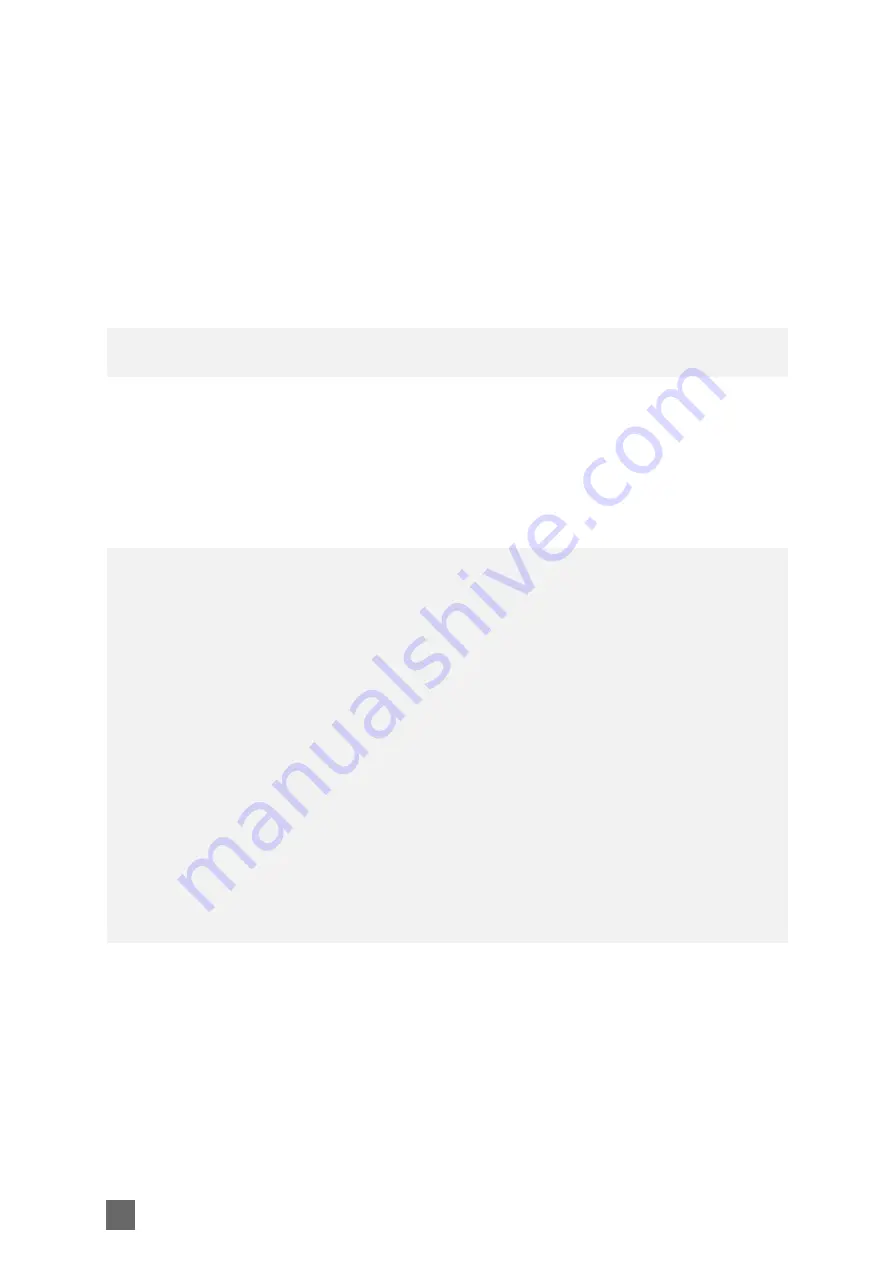
16
4. Use
to find out the ID of the Coaxlink card.
Euresys::InterfaceModule
indicates that we want an answer from the
5. Similarly, find out the ID of the device. This time, we use
Euresys::DeviceModule
to target
the
6. Finally, read the camera resolution.
Euresys::RemoteModule
indicates that the value must
be retrieved from the camera.
7.
Euresys::EGrabber
uses exceptions to report errors, so we wrap our code inside a
try
... catch
block.
Example of program output:
Interface:
PC1633 - Coaxlink Quad G3 (2-camera) - KQG00014
Device:
Device0
Resolution:
4096x4096
5.2. Acquiring images
This program uses
Euresys::EGrabber
to acquire images from a camera connected to a
Coaxlink card:
#include <iostream>
#include <EGrabber.h>
void grab() {
Euresys::EGenTL gentl;
Euresys::EGrabber<> grabber(gentl);
// 1
grabber.reallocBuffers(3);
// 2
grabber.start(10);
// 3
for (size_t i = 0; i < 10; ++i) {
Euresys::ScopedBuffer buf(grabber);
// 4
void *ptr = buf.getInfo<void *>(GenTL::BUFFER_INFO_BASE);
// 5
uint64_t ts = buf.getInfo<uint64_t>(GenTL::BUFFER_INFO_TIMESTAMP);
// 6
std::cout << "buffer address: " << ptr << ", timestamp: "
<< ts << " us" << std::endl;
}
// 7
}
int main() {
try {
grab();
} catch (const std::exception &e) {
std::cout << "error: " << e.what() << std::endl;
}
}
1. Create a
Euresys::EGrabber
object. The second and third arguments of the constructor
are omitted here. The grabber will use the first device of the first interface present in the
system.
2. Allocate 3 buffers. The grabber automatically determines the required buffer size.
Coaxlink
Programmer Guide
5. Euresys::EGrabber