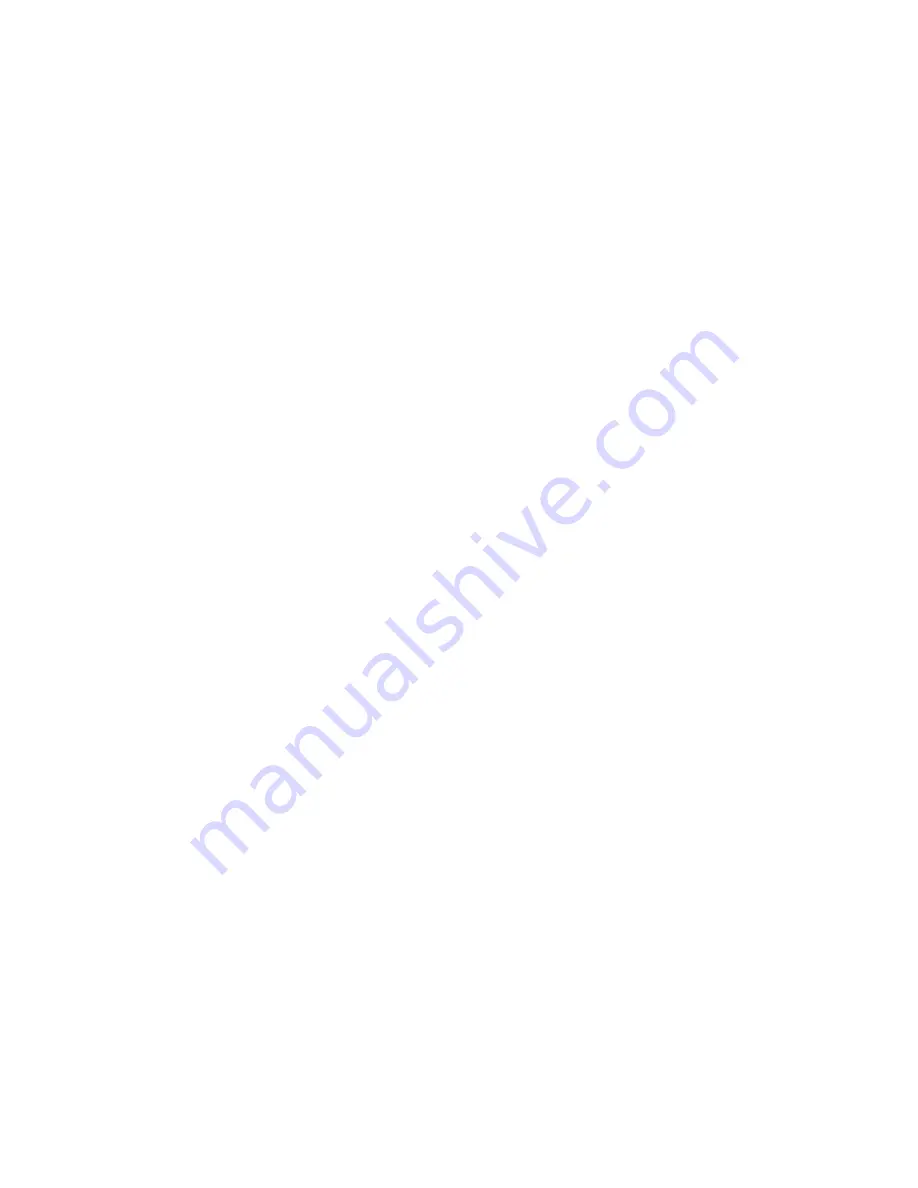
COMMERCIAL IN CONFIDENCE
Page 14
2.6
CRC calculation
NOTE that the CRC algorithm published in the Modicon ModBus Protocol Guide (PI-MBUS-300
Rev G, Nov 1994) IS WRONG!!!!
However, the quick C program using the lookup tables is correct.
The correct algorithm is given here
1. Load a 16 bit register with FFFF (all 1 s). Call this the CRC register.
2. Exclusive OR the first 8 bit byte of the message with the low-order byte of the 16 bit CRC
register, putting the result back into the CRC register
3. Look at the Least Significant Bit of the CRC register and remember it. Call it the
LastBit
4. Shift the CRC register one bit right, putting 0 in the top bit
5. If the
LastBit
was 1, Exclusive OR the CRC register with value A001h (1010 0000 0000
0001)
6. Repeat steps 3,4,5 until 8 shifts have been performed
7. Repeat from step 2 for the next byte of the message until all bytes have been processed
8. The final contents of the CRC register is the CRC value to use
9. When the CRC is placed in the message, the Least Significant Byte is sent first, then the
Most Significant Byte
2.6.1
CRC calculation in C code
There are two ways to implement the CRC, one uses the above algorithm, the other uses pre-
computed lookup tables which make for a faster calculation. This is given correctly in the ModBus
guide, and can be downloaded from the Internet (search for ModBus and CRC and Generation) and is
not repeated here. The long winded way is as follows (where mess[] holds the message):
unsigned short crc;
unsigned short thisbyte;
unsigned short shift;
unsigned char highbyte, lowbyte;
unsigned char lastbit, i;
crc=0xffff;
for (i=0; i<len(mess); i++)
{
thisbyte= mess[i];
crc = crc^thisbyte;
for (shift=1; shift<=8; shift++)
{
lastbit = crc & 1;
crc = (crc >> 1) & 0x7fff;
if (lastbit==1)
{
crc = crc^0xA001 ;
}
}
}
highbyte=(crc>>8)&0xff;
lowbyte=crc&0xff;