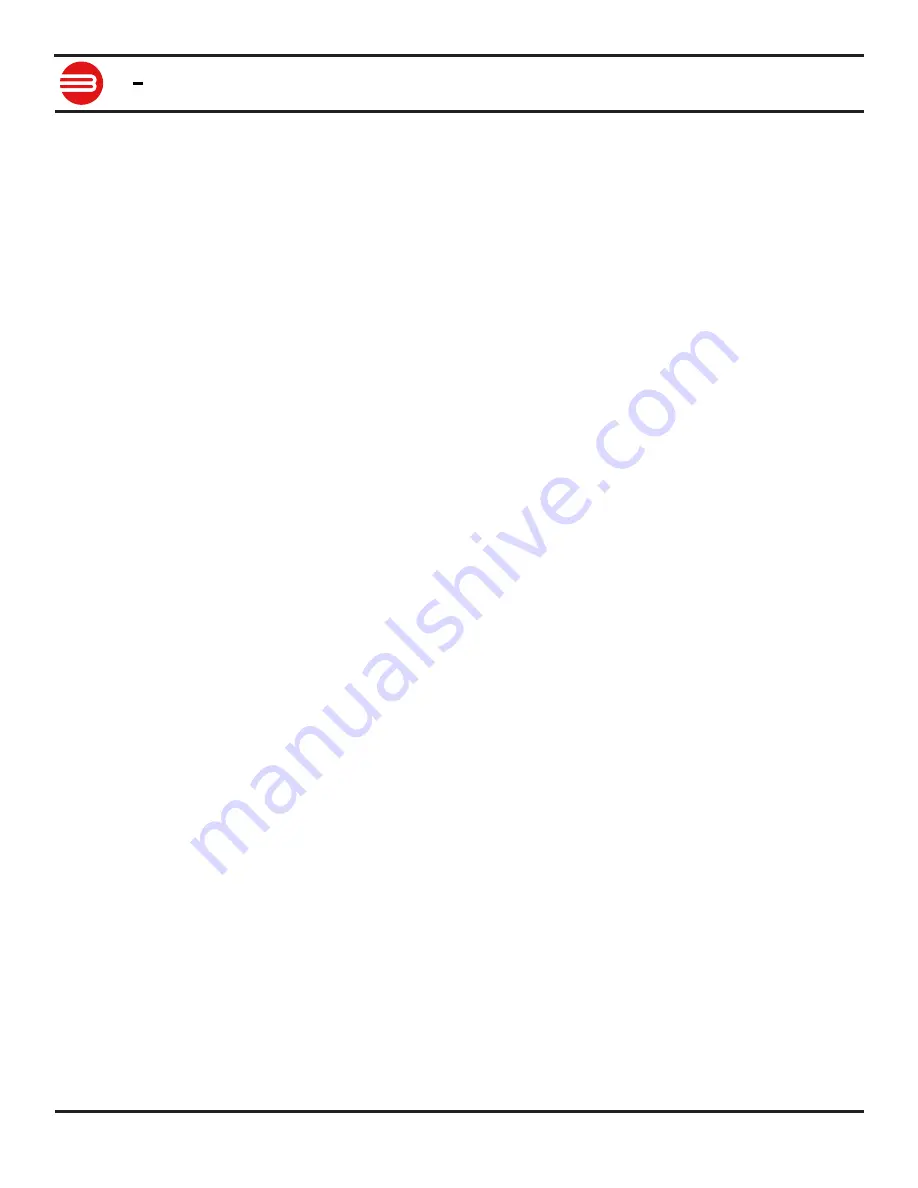
RoboClaw Series
Brushed DC Motor Controllers
RoboClaw Series User Manual
59
BASICMICRO
Packet Timeout
When sending a packet to RoboClaw, if there is a delay longer than 10ms between bytes being
received in a packet, RoboClaw will discard the entire packet. This will allow the packet buffer to
be cleared by simply adding a minimum 10ms delay before sending a new packet command in
the case of a communications error. This can usually be accomodated by having a 10ms timeout
when waiting for a reply from the RoboClaw. If the reply times out the packet buffer will have
been cleared automatically.
Packet Acknowledgement
RoboClaw will send an acknowledgment byte on write only packet commands that are valid. The
value sent back is 0xFF. If the packet was not valid for any reason no acknowledgement will be
sent back.
CRC16 Checksum Calculation
Roboclaw uses a CRC(Cyclic Redundancy Check) to validate each packet it receives. This is more
complex than a simple checksum but prevents errors that could otherwise cause unexpected
actions to execute on the Roboclaw.
The CRC can be calculated using the following code(example in C):
//Calculates CRC16 of nBytes of data in byte array message
unsigned int crc16(unsigned char *packet, int nBytes) {
for (int byte = 0; byte < nBytes; byte++) {
crc = crc ^ ((unsigned int)packet[byte] << 8);
for (unsigned char bit = 0; bit < 8; bit++) {
if (crc & 0x8000) {
crc = (crc << 1) ^ 0x1021;
} else {
crc = crc << 1;
}
}
}
return crc;
}
CRC16 Checksum Calculation for Received data
The CRC16 calculation can also be used to validate received data from the Roboclaw. The CRC16
value should be calculated using the sent Address and Command byte as well as all the data
received back from the Roboclaw except the two CRC16 bytes. The value calculated will match
the CRC16 sent by the Roboclaw if there are no errors in the data sent or received.
Easy to use Libraries
Source code and Libraries are available on the BasicMicro website that already handle the
complexities of using packet serial with the Roboclaw. Libraries are available for Arduino(C++),
C# on Windows(.NET) or Linux(Mono) and Python(Raspberry Pi, Linux, OSX, etc).