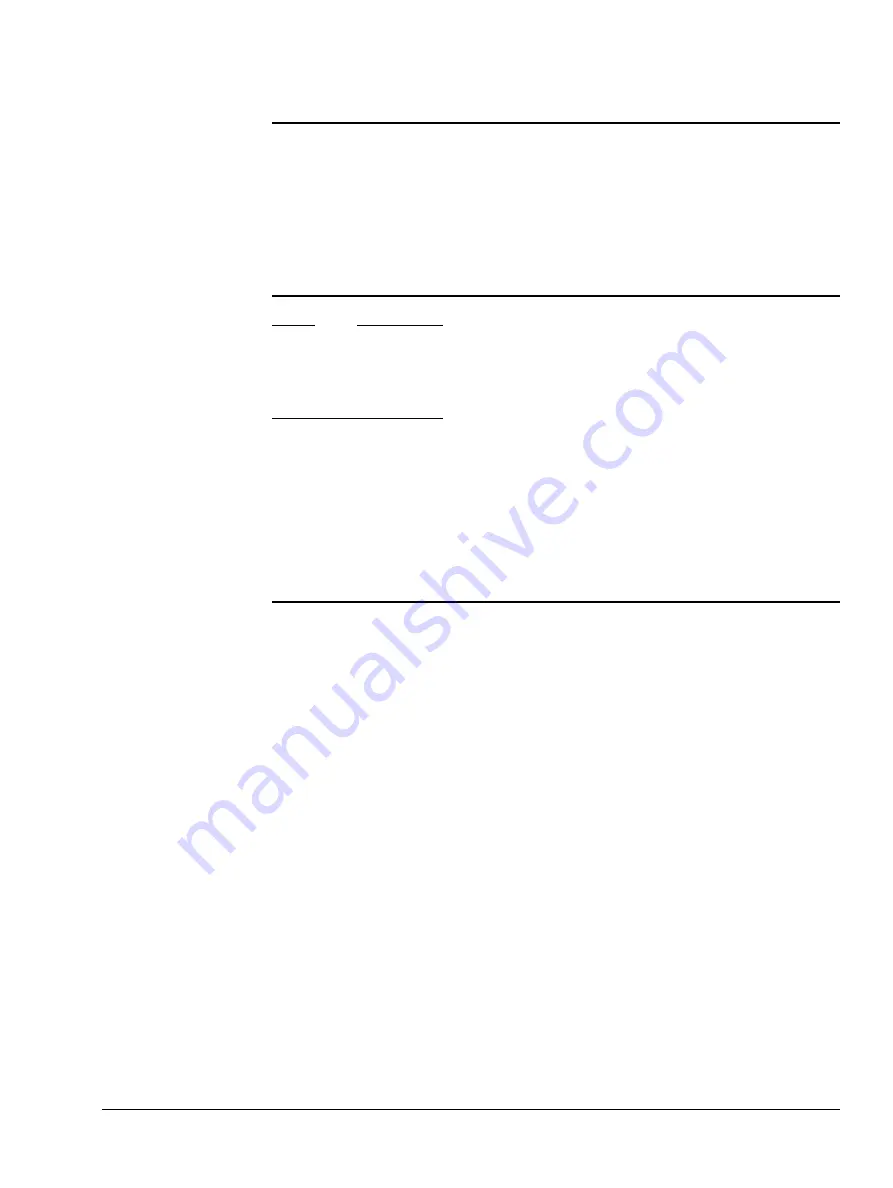
Debug
ARM DDI 0363G
Copyright © 2006-2011 ARM Limited. All rights reserved.
12-71
ID073015
Non-Confidential
Example 12-24 Writing registers in stall mode
WriteRegisterInStallMode(int Rd, uint32 value)
{
// Step 1. Write the value to the DBGDTRRX.
// Write stalls until the DBGDTRRX is ready.
WriteDebugRegister(32, value);
// Step 2. Write the opcode for MRC p14, 0, Rd, c5, c0 to the DBGITR.
// Write stalls until the DBGITR is ready.
WriteDebugRegister(33, 0xEE (Rd<<12));
}
Note
To transfer a register to the processor when in stall mode, you are not required to poll the
DBGDSCR each time an instruction is written to the DBGITR and a value read from or written
to the DTR. The processor stalls using the signal
PREADYDBG
until the previous instruction
has completed or the DTR register is ready for the operation.
Fast memory read/write
This section provides example code to enable faster reads from memory by making use of the
DTR access mode.
shows the sequence for reading a block of words of memory.
Example 12-25 Reading a block of words of memory
ReadWords(uint32 address, bool &aborted, uint32 *data, int nwords)
{
// Step 1. Write the value 0b01 to DBGDSCR[21:20] for stall mode.
SetDTRAccessMode(1);
// Step 2. Save the value of R0.
saved_r0 := ReadRegisterInStallMode(0);
// Step 3. Write the address to read from to the DBGDTRRX.
// Write stalls until the DBGDTRRX is ready.
WriteRegisterInStallMode(0, address);
// Step 4. Write the opcode for LDC p14, c5, [R0], 4 to the DBGITR.
// Write stalls until the DBGITR is ready.
WriteDebugRegister(33, 0xECB05E01);
// Step 5. Write the value 0b10 to DBGDSCR[21:20] for fast mode.
SetDCCAccessMode(2);
// Step 6. Loop reading out the data.
// Each time a word is read from the DBGDTRTX, the instruction is reissued.
while (nwords > 1)
{
*data++ = ReadDebugRegister(35);
--nwords;
}
// Step 7. Write the value 0b00 to DBGDSCR[21:20] for non-blocking mode.
SetDTRAccessMode(0);
// Step 8. Need to wait for the final instruction to complete. If there
// was an abort, this completes immediately.
do
{
dscr := ReadDebugRegister(34);
}
until (dscr & (1<<24));