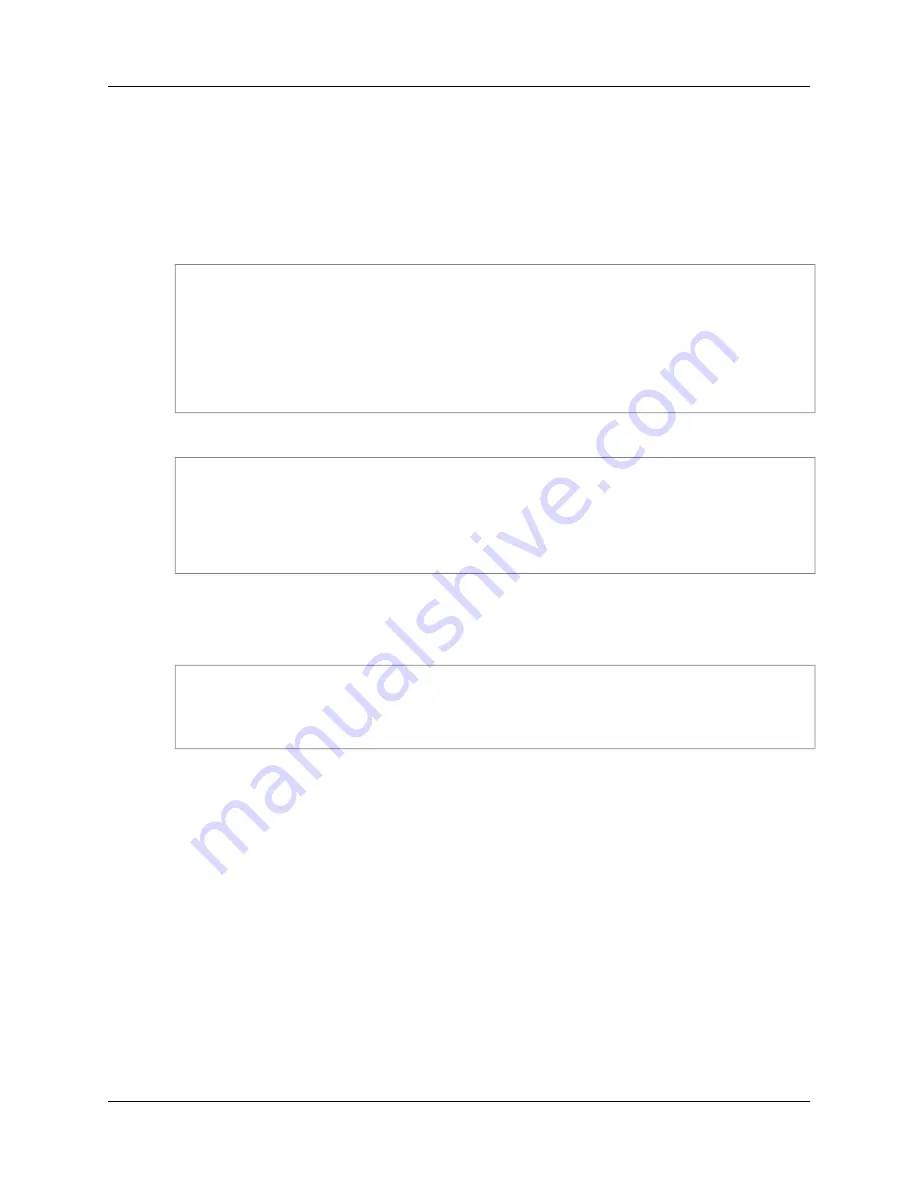
Simplified Callback Method
Each operation supports a simplified callback that can be passed as the last parameter to any low-level
client operation. The callback function should accept an
error
parameter, followed by the
data
from
the response.
For example:
s3.client.listBuckets(function(error, data) {
if (err) {
console.log(error); // error is Response.error
} else {
console.log(data); // data is Response.data
}
});
Prints (assuming the request succeeded):
{ Owner: { ID: '...', DisplayName: '...' },
Buckets:
[ { Name: 'someBucketName', CreationDate: someCreationDate },
{ Name: 'otherBucketName', CreationDate: otherCreationDate } ],
RequestId: '...' }
The error and data parameters accepted are equivalent to the
error
and
data
properties discussed in
the
AWS.Response
response object section above.
If you are passing parameters to the operation, the callback should be placed after the parameters:
s3.client.getObject({Bucket: 'bucket', Key: 'key'}, function(err, data) {
// ...
});
AWS.Request Events
You can alternatively register callbacks on events provided by the
AWS.Request
object returned by each
low-level client operation method. This request object exposes the
success
,
error
,
complete
, and
httpData
events, each taking a callback that accepts the response object.
Note that if you omit the simplified callback parameter on the operation method, you must call
send()
on the returned request object in order to kick off the request to the remote server.
on('success', function(response) { ... })
This event triggers when a successful response from the server is returned. The response contains a
.data
field with the serialized response data from the service.
For example:
Version 0.9.1-pre.2 : Preview
11
AWS SDK for Node.js Getting Started Guide
Simplified Callback Method