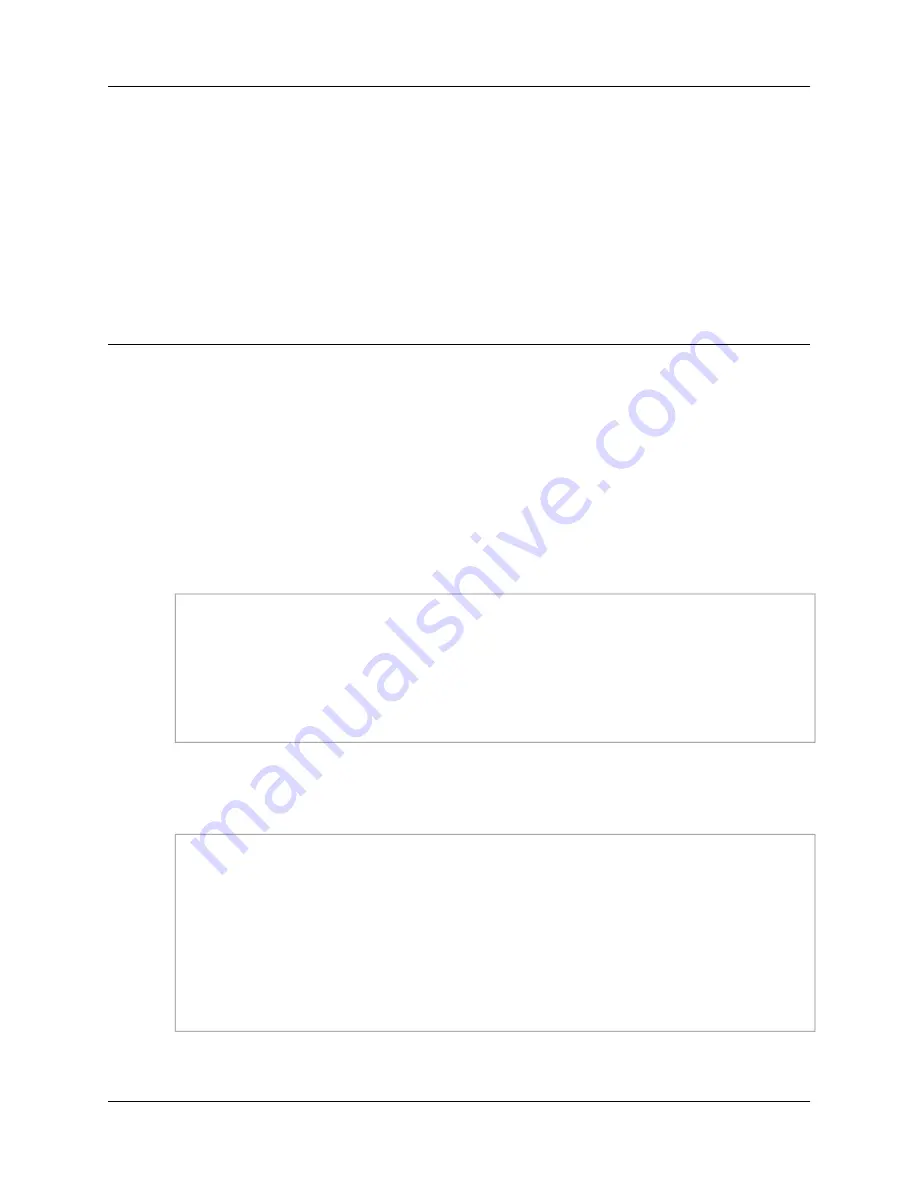
Making Requests
Asynchronous Callbacks
All requests made through the SDK are asynchronous and use a callback interface. Each service method
that kicks off a request can accept a callback as the last parameter with the signature
function(error,
data) { ... }
. This callback will be called when the response or error data is available.
For example, the following service method can be called with a standard callback to retrieve the response
data or error:
new AWS.EC2().client.describeInstances(function(error, data) {
if (error) {
console.log(error); // an error occurred
} else {
console.log(data); // request succeeded
}
});
The
error
and
data
parameters are described in the Response Object section below.
Note that if you do not specify a callback, the operation will return an
AWS.Request
object that must be
manually sent using the
send()
method:
// create the AWS.Request object
var request = new AWS.EC2().client.describeInstances();
// register a callback to report on the data
request.on('success', function(resp) {
console.log(response.data); // log the successful data response
});
// send the request
request.send();
Version 0.9.1-pre.2 : Preview
9
AWS SDK for Node.js Getting Started Guide
Asynchronous Callbacks