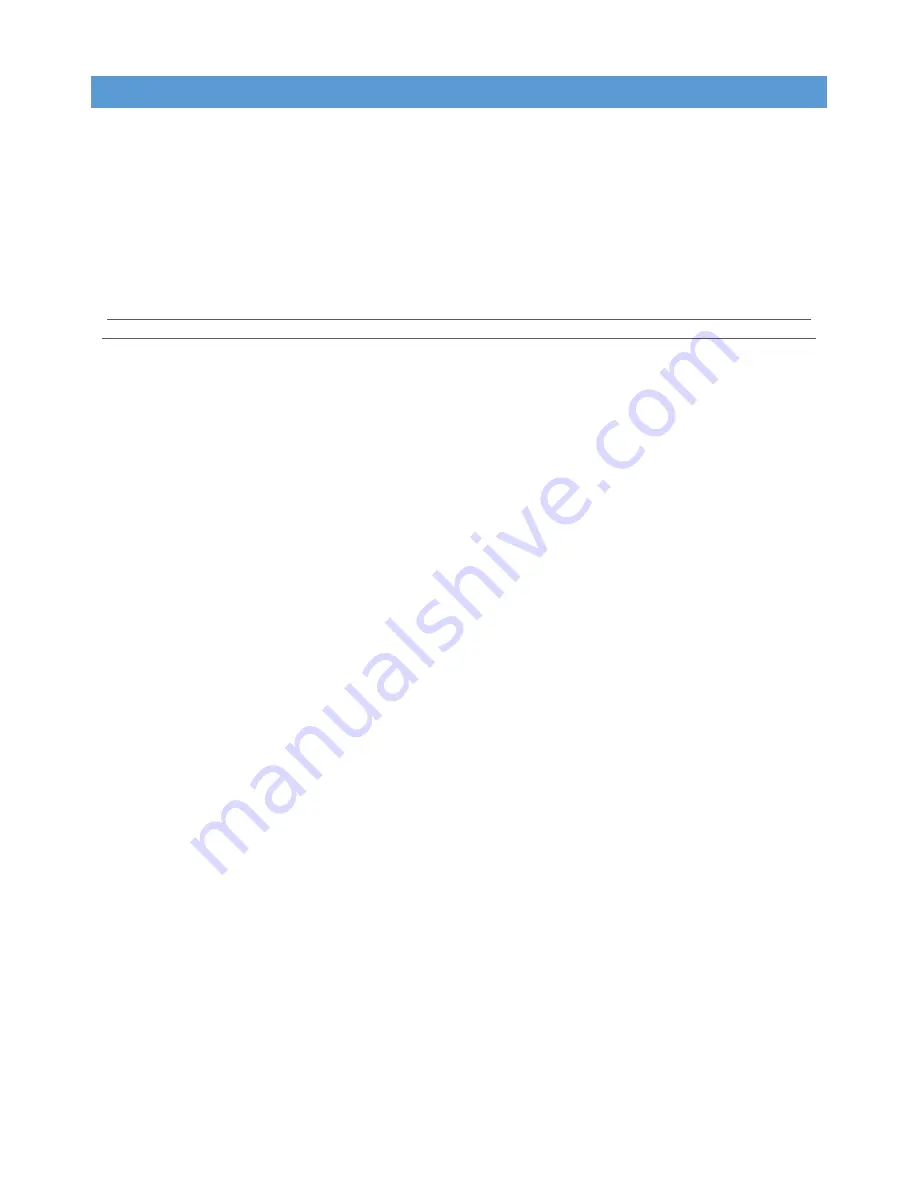
[37]
APPENDIX D – TAP EMBEDDED CONTROL CHARACTERS
To embed a control character in TAP mode,
a <SUB> control character is required followed by an
offset version of the control character you want.
You offset the control character by adding 64 to the control
character’s ASCII Decimal Value to make the character printable. Adding 64 to
Carriage Return (13)
gives you
M (77)
. Adding 64 to
Line Feed (10)
gives you
J (74)
. The character combination of
<SUB>M
causes a Carriage
Return control character to be embedded in the encoded paging message, while
<SUB>J
causes a Line Feed
control character to be embedded.
THE FOLLOWING CODE, IN VB .NET, DEFINES THE EMBEDDED CONTROL CHARACTER STRING TO BE
DELIVERED THROUGH THE SERIAL PORT TO THE PAGING SYSTEM, SENDS IT, AND READS THE REPLY:
Imports System.IO.Ports
‘Imports the code library containing SerialPort among other things.
Dim SerialP As SerialPort = New SerialPort With
{
.PortName = “COM1”,
‘These are the default values for making a serial
.BaudRate = 9600,
connection to a WaveWare v9 Series Paging System.
.Parity = None,
‘The port name changes to the port that is the
.Databits = 8,
connection between the host device and the system.
.Stopbits = 1
}
‘Subs execute code without returning a value to whatever called for it.
Public Sub Write2Lines(ByVal MsgLine1 As String, ByVal MsgLine2 As String, ByVal ID as String)
‘ByVal variables need to have their value assigned to them by whatever section of code calls for this Sub.
Dim CRSub As String = Chr(26) & "M"
‘chr(26) = <SUB>, chr(13) = <CR>, chr(13 + 64) = M
Dim LFSub As String = Chr(26) & "J"
‘chr(26) = <SUB>, chr(10) = <LF>, chr(10 + 64) = J
Dim MSG As String = MsgLine1 & CRSub & LFSub & MsgLine2
‘Combine everything into one string
MSG = CreateTapMsg(ID, MSG)
‘See Appendix C – TAP Checksum Calculation to see changes in the string.
SerialP.Open()
‘Opens the connection between the device running this code and the paging system.
SerialP.Write(MSG)
‘Sends the TAP Message to the paging system
Dim RXstring As String = SerialP.ReadTo(chr(13))
‘ReadTo() reads serial data until you come across a
RX= SerialP.ReadTo(chr(13))
specific character or string. This is done twice bec-
Console.Write(RXstring)
-ause TAP has two <CR> in its replies.
SerialP.Close()
‘Closes the connection between the device running this code and the paging system.
End Sub
When using WaveWare Protocol it is not necessary to make use of the <SUB> control character for
<CR> or <LF>, since the WaveWare protocol doesn’t use either control character in its message format.