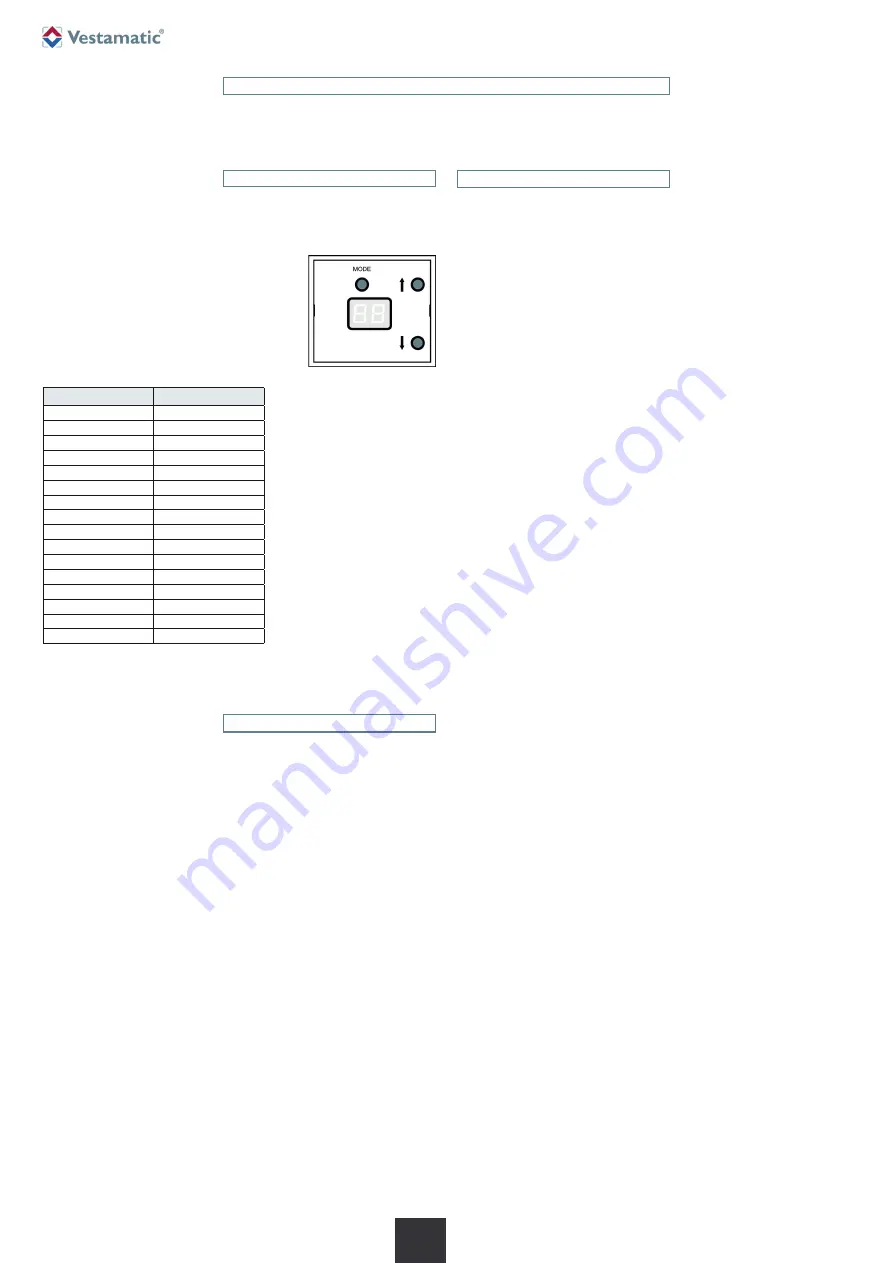
Subject to modifications.
© Vestamatic GmbH
Art.-Nr.:
3060 001 GB 4518 A06 • Vestamatic GmbH • Dohrweg 27 • D-41066 Mönchengladbach
• www.vestamatic.com
4/10
G
IF SMI RS-485 DIN
6.2 CRC16 Calculation
Example code CRC16 calculation in C-language:
#define CRC_CONSTANT
0xA001
word Crc16 (byte* pb, byte len)
{
byte i;
word crc;
for (crc=0xffff; len--; pb++)
{
crc ^= (byte)*pb;
for (i=8; i--; )
if (crc & 0x01)
{
crc
>>=
1;
crc
^=
CRC_CONSTANT;
}
else
crc
>>=
1;
}
// return crc result
return crc;
}
6. Protocol
This chapter describes the communication protocol between the IF SMI RS-485 module
and Host controller.
6.3 Steer commands
#define MSG_UP
0x10
#define MSG_DOWN
0x11
#define MSG_STOP
0x12
#define MSG_STEP_UP
0x13
#define MSG_STEP_DOWN
0x14
#define MSG_SET_POS
0x15
#define MSG_SET_TILT
0x16
#define MSG_SET_POS_STEP_UP 0x17
#define MSG_SET_POS_STEP_DOWN 0x18
#define MSG_GOTO_POS1
0x19
#define MSG_GOTO_POS2
0x1A
#define MSG_GETMANID
0x2C
#define MSG_SMI_TUNNEL
0x2D
#define MSG_GW_OPTIONS
0x50
#define MSG_ERROR
0xE0
6.1 Message structure
[SID] [LEN] [CMD] [DATA] [CRC16]
SID
Slave ID
LEN
Message length (without CRC)
CMD
Command byte
DATA
Optional data bytes
CRC16
16 bits checksum (LSB first)
The IF SMI RS-485 module has 4
DIP switches, which represents the base
address of the module from 0 to 15 (decimal)
or 0 to F (hexadecimal). The base address
is part of the Slave ID:
Base address
Slave ID
0
0xC0
1
0xC1
2
0xC2
3
0xC3
4
0xC4
5
0xC5
6
0xC6
7
0xC7
8
0xC8
9
0xC9
10
0xCA
11
0xCB
12
0xCC
13
0xCD
14
0xCE
15
0xCF
6.3.1 MSG_UP
This message is used to move all, or a set of sun blind, to the
top
position.
Message:
[SID] / [LEN] / [CMND] / [MSK0] / [MSK1] / [CRC16]
[MSK0]:
LSB of 16-bit mask to select motor address 0..7.
[MSK1]:
MSB of 16-bit mask to select motor address 8..16.
When bit
n
in mask is set, SMI motor with address
n
is addressed and
executes a
UP
command.
The IF SMI RS-485 module responds to this request with a general status
message MSG_GETGENSTAT.
The position of each sun blind can be obtained by the detailed response
message MSG_GETDETSTAT.
6.3.2 MSG_DOWN
This message is used to move all, or a set of sun blind, to the
lower
position.
Message:
[SID] / [LEN] / [CMND] / [MSK0] / [MSK1] / [CRC16]
[MSK0]:
LSB of 16-bit mask to select motor address 0..7.
[MSK1]:
MSB of 16-bit mask to select motor address 8..16.
When bit
n
in mask is set, SMI motor with address
n
is addressed and
executes a
DOWN
command.
The IF SMI RS-485 module responds to this request with a general status
message MSG_GETGENSTAT.
The position of each sun blind can be obtained by the detailed response
message MSG_GETDETSTAT.
6.3.3 MSG_STOP
This message is used to stop all, or a set of sun blind.
Message:
[SID] / [LEN] / [CMND] / [MSK0] / [MSK1] / [CRC16]
[MSK0]:
LSB of 16-bit mask to select motor address 0..7.
[MSK1]:
MSB of 16-bit mask to select motor address 8..16.
The IF SMI RS-485 module responds to this request with a general status
message MSG_GETGENSTAT.
The position of each sun blind can be obtained by the detailed response
message MSG_GETDETSTAT. The IF SMI RS-485 module responds to
this request with a general status message MSG_GETGENSTAT.
The position of each sun blind can be obtained by the detailed response
message MSG_GETDETSTAT.