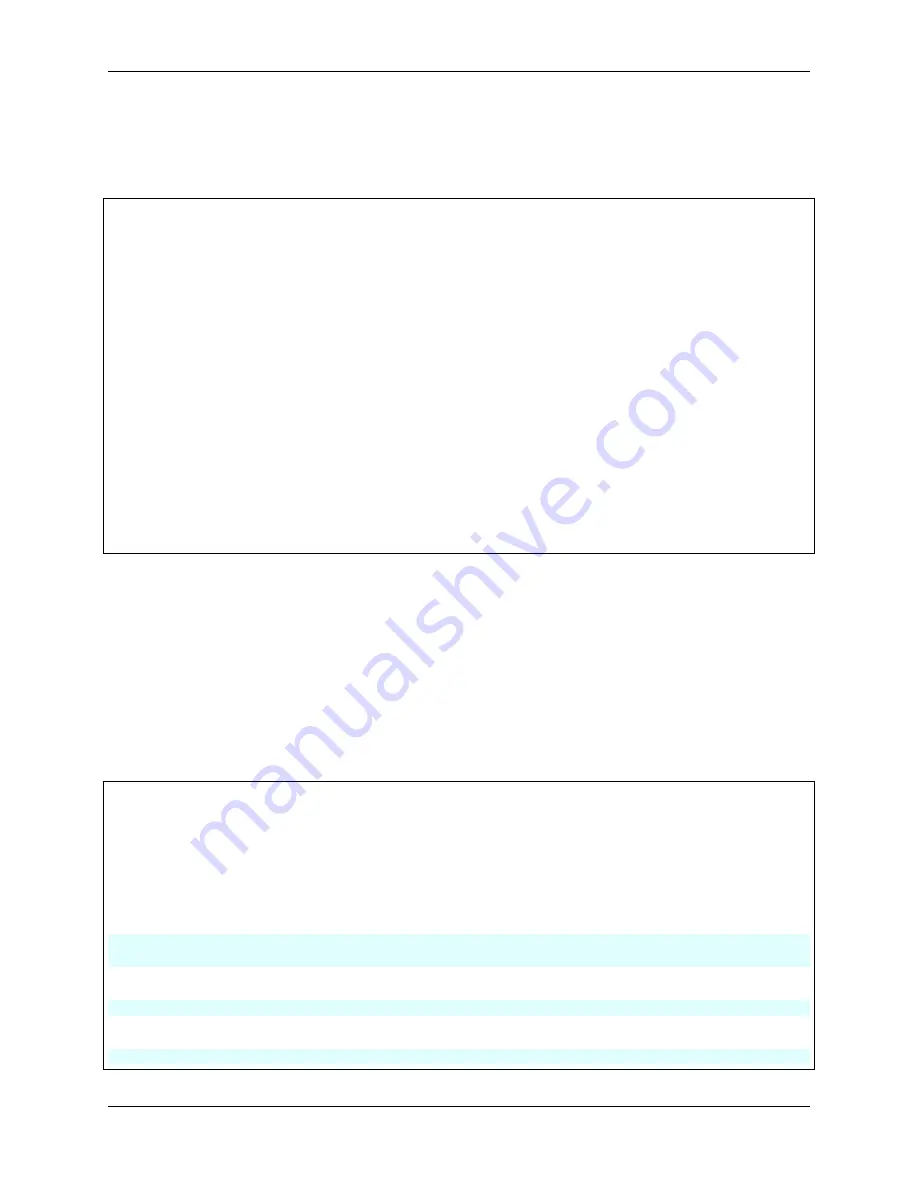
SunFounder Thales Kit for Raspberry Pi Pico, Release 1.0
Here, the
_thread
library is imported first. This module implements multithreading support. Then define a thread
button_thread()
, which is independent of the main thread. It is used here to read the state of the button. Finally
use
_thread.start_new_thread(button_thread, ())
to start the thread.
The following sample code can help you better understand multithreading:
import
machine
import
utime
import
_thread
led_red
=
machine
.
Pin(
15
, machine
.
Pin
.
OUT)
led_yellow
=
machine
.
Pin(
14
, machine
.
Pin
.
OUT)
button
=
machine
.
Pin(
16
, machine
.
Pin
.
IN)
def
led_yellow_thread
():
while
True
:
led_yellow
.
toggle()
utime
.
sleep(
2
)
_thread
.
start_new_thread(led_yellow_thread, ())
while
True
:
button_status
=
button
.
value()
if
button_status
==
1
:
led_red
.
value(
1
)
elif
button_status
==
0
:
led_red
.
value(
0
)
In the main thread, the button is used to control the red LED on and off. In the new thread (led_yellow_thread()), the
yellow LED will change every 2 seconds. The two threads work independently of each other.
Let’s go back to the traffic signal program. We let the main thread change the light and let the new thread read the
button value. However, the threads are independent of each other, and we need a way for the new thread to pass
information to the main thread, which requires the use of global variable.
The variables we have used before are all local variables, acting only in a certain part of the program (Variables
declared in the main function cannot be used in sub-functions, and variables declared in the main thread cannot be
used in the new thread). The global variable can be used anywhere, we change it in one thread, and the other can get
its updated value.
Global variables are in these places:
import
machine
import
utime
import
_thread
led_red
=
machine
.
Pin(
15
, machine
.
Pin
.
OUT)
led_yellow
=
machine
.
Pin(
14
, machine
.
Pin
.
OUT)
led_green
=
machine
.
Pin(
13
, machine
.
Pin
.
OUT)
button
=
machine
.
Pin(
16
, machine
.
Pin
.
IN)
global
button_status
button_status
=
0
def
button_thread
():
global
button_status
while
True
:
if
button
.
value()
==
1
:
button_status
=
1
(continues on next page)
3.4. Projects
67
Summary of Contents for Thales Kit
Page 1: ...SunFounder Thales Kit for Raspberry Pi Pico Release 1 0 Jimmy SunFounder Jun 04 2021 ...
Page 2: ......
Page 4: ...ii ...
Page 6: ...SunFounder Thales Kit for Raspberry Pi Pico Release 1 0 2 CONTENTS ...
Page 140: ...SunFounder Thales Kit for Raspberry Pi Pico Release 1 0 136 Chapter 3 For MicroPython User ...
Page 164: ...SunFounder Thales Kit for Raspberry Pi Pico Release 1 0 160 Chapter 4 For Arduino User ...