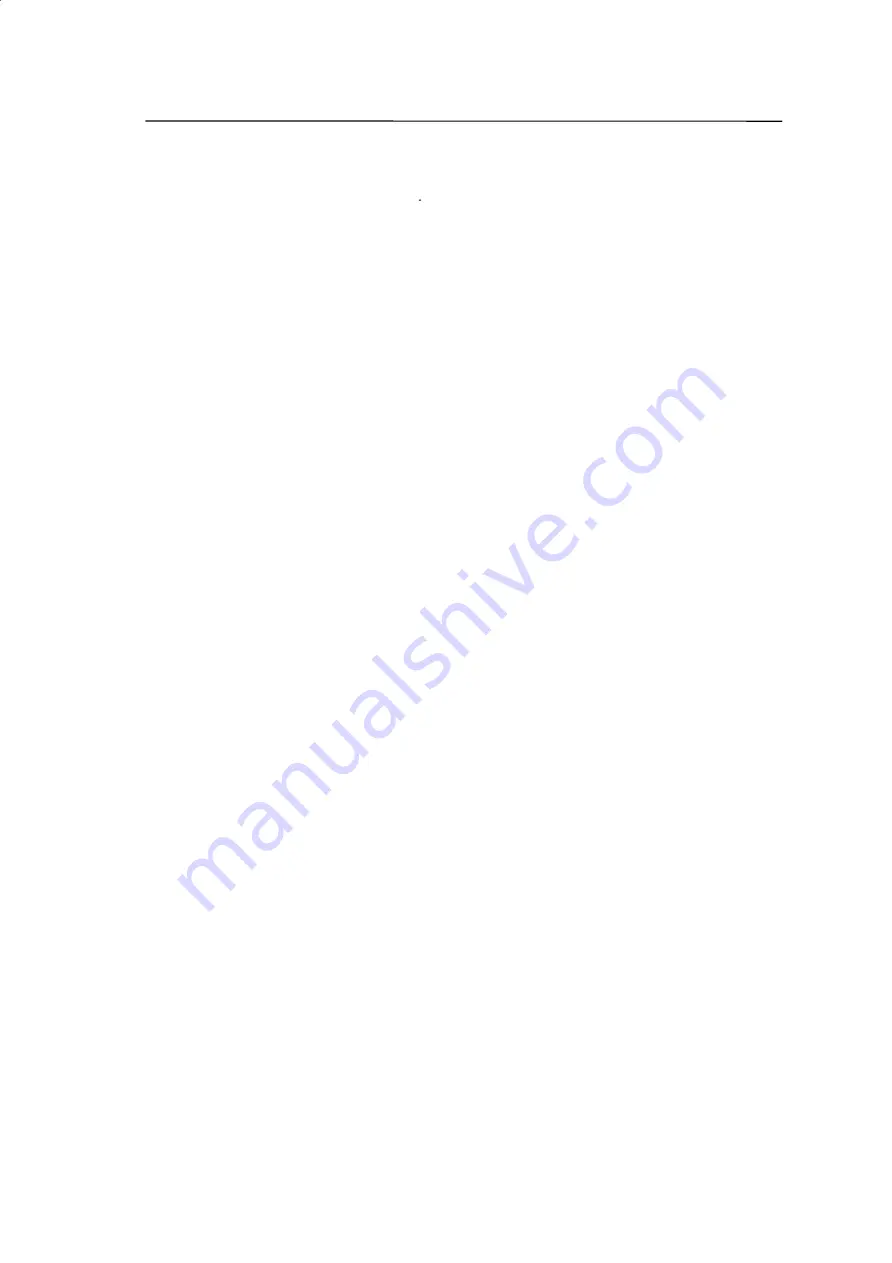
APPENDIX
A. MCU Sample Program
C8051 MCU C Language
//-----------------------------------------------------------------------------
// Includes
//-----------------------------------------------------------------------------
#include<reg52.h>
//-----------------------------------------------------------------------------
// sbit Definitions
//-----------------------------------------------------------------------------
sbit LED=P0^0;
//-----------------------------------------------------------------------------
// Global CONSTANTS
//-----------------------------------------------------------------------------
#define SYSCLK 22118400 // “SYSCLK frequency in Hz”
#define BAUD_RATE 115200 // “Baudrate”
#define uchar unsigned char
#define uint unsigned int
uchar pic[3]={0xAA,0x70,0x08};
/-----------------------------------------------------------------------------
// Function PROTOTYPES
//-----------------------------------------------------------------------------
void Uart0_transmit(uchar i); // “Send a byte to the terminal”
void send_str(uchar *p,uchar s); // “Send a string to the terminal”
void delay_ms(uchar n); // “Delay”
void SysInit(void); // “Initialization of system”
void en(void); // “Frame end”
void pic_str(uchar i); // “Picture switching sub-function”
//-----------------------------------------------------------------------------
// Uart0_transmit
//-----------------------------------------------------------------------------
void Uart0_transmit(uchar i) // “Send 1 byte to terminal”
{
ES=0;
TI=0;
SBUF=i;
// “Send data to uart0”
while (!TI);
//“Wait for the finish of sending a byte”
TI= // “Clear the interruption mark”
ES=1;
}
void send_str(uchar *p,uchar s) // “Send a string to the terminal”
{
uchar m;
for(m=0;m<s;m++)
{
Uart0_transmit(*p);
p++;
}
}
//-----------------------------------------------------------------------------
// delay // “Delay sub-function”
//-----------------------------------------------------------------------------