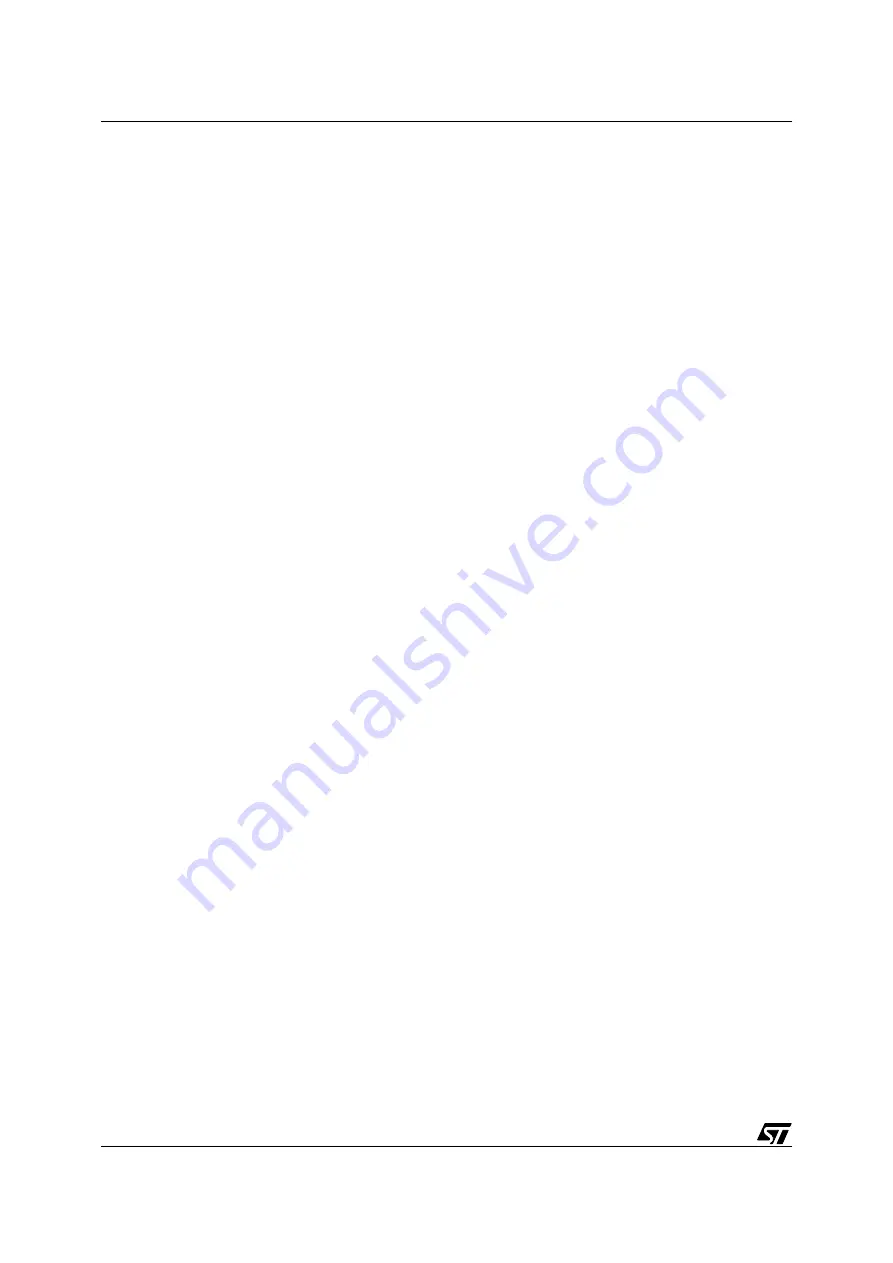
230/317
8 - C Language and the C Compiler
8.3.2 Initialization of variables and constant variables
On starting, a C program initializes all variables to zero, except those that are defined with an
initial value. Example:
int i ;
int j = 3 ;
Here
i
is initialized to zero, and
j
is initialized to 3. This is performed as follows:
Any HIW ARE C program must be linked with an additional file called
Start07
that is available
in the software package. In most cases, this file is suitable as is, and its object file,
Start07.o
can be directly linked with the other object files issuing from the compilation of the C source
files of the project. In case this file should be altered (which can only be done by an expert pro-
grammer), its source text,
Start07.c
, is also available. This file may be copied to the project
directory and altered to meet the requirements of the project. It becomes one of the modules
of the project, and will be compiled along with the other files.
As explained above, all variables are allocated by default to the DEFAULT_RAM, and they are
initialized before the user program starts by copying their values, stored by the compiler in
ROM, into the appropriate RAM locations. If initialized variables are actually constants, and
especially if they are bulky, do not forget to move them into the ROM_VAR segment, using the
const
attribute and the
-Cc
command-line option, otherwise the RAM memory would very
quickly overflow. F or e xa mple, here is the conversion table that, when accessed with a
number between 0 and 15, returns the appropriate character to express that number in hexa-
decimal:
const char HEXADECIMAL[] = {'0', '1', '2', '3', '4', '5', '6', '7', '8',
'9', 'A', 'B', 'C', 'D', 'E', 'F'} ;
If the
-Cc
option is used, this array will not clutter up the RAM.
8.3.3 Inputs and outputs
Inputs and outputs are accessed using their registers that are mapped in the lower data
memory, that is, in page zero. The C compiler has no provision for defining these registers.
However, since they are mapped in memory, they can be considered as mere variables. The
only constraint is that they must occupy precise addresses.