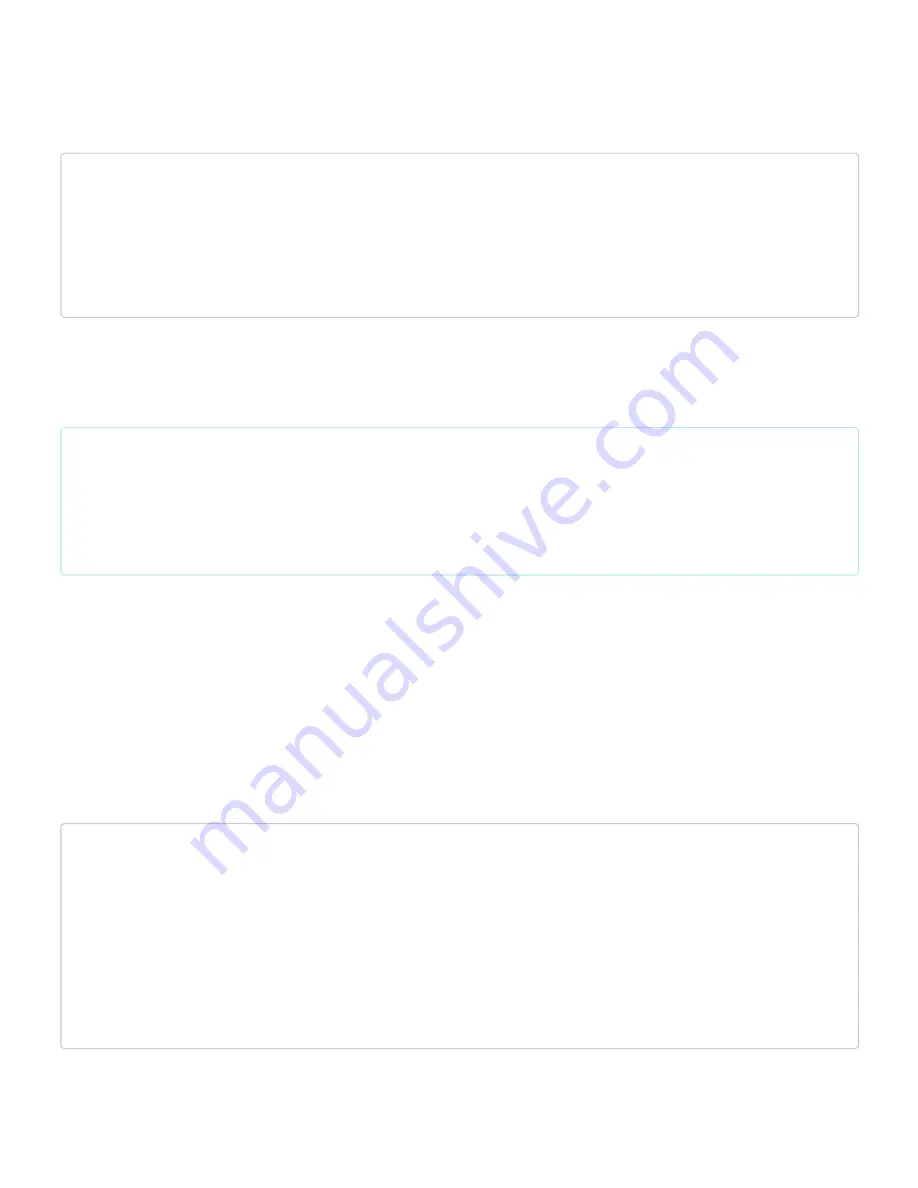
Example 3B: Inversion - Port
Example 3B, as you might expect by now, exhibits how to invert the entire port on the Qwiic GPIO. The code starts
as the other port examples do by defining the number of pins and setting the pin mode of all pins on the port. It
then inverts half of the pins' polarity using a second boolean. The setup can be seen below:
#define NUM_GPIO 8
bool currentPinMode[NUM_GPIO] = {GPIO_IN, GPIO_IN, GPIO_IN, GPIO_IN, GPIO_IN, GPIO_IN, GPIO_IN,
GPIO_IN};
bool inversionStatus[NUM_GPIO] = {INVERT, INVERT, INVERT, INVERT, NO_INVERT, NO_INVERT, NO_INVER
T, NO_INVERT};
The main loop is identical to Example 2B - ReadGPIO - Port and prints out the state of the port register using an
unsigned 8-bit integer.
Example 4: Interrupt
Heads Up!
In this example we use D13 as our interrupt pin since the code was written for use with a
SparkFun RedBoard/Arduino Uno. If you are using a different microcontroller with your Qwiic GPIO, take a
look through its documentation to see which pins can be used as an interrupt and then adjust this definition:
#define INTERRUPT PIN 13
to the selected pin. If you are not familiar with using processor interrupts on a
microcontroller, this tutorial will help you get started.
Example 4 demonstrates how to use the interrupt pin on the TCA9534 when any input pin registers a change. The
interrupt pin is active LOW and will return to HIGH once the input register is read. We've broken the INT pin out to
a latch terminal so you can connect a wire to that terminal and tie it to the interrupt-capable pin on your
microcontroller. If you prefer, the INT pin is also broken out to a PTH pin you can solder to. Whichever option you
choose, connect the interrupt pin on your Qwiic GPIO to D13 on your Arduino (assuming you are using an
Uno/RedBoard).
The code starts similarly to our other Port examples by defining the number of GPIO pins and setting their pin
status. Along with the GPIO pins, we define the interrupt pin on our microcontroller. You can view the pertinent bits
of this code below:
#define NUM_GPIO 8
#define INTERRUPT_PIN 13
bool currentPinMode[NUM_GPIO] = {GPIO_IN, GPIO_IN, GPIO_IN, GPIO_IN, GPIO_IN, GPIO_IN, GPIO_IN,
GPIO_IN};
bool gpioStatus[NUM_GPIO];
bool dataReady = true;
With everything defined, the setup initializes the Qwiic GPIO on the I C bus, sets the interrupt pin on the
microcontroller as an input, "primes" it by writing it HIGH and tells our microcontroller to treat it as an interrupt:
2