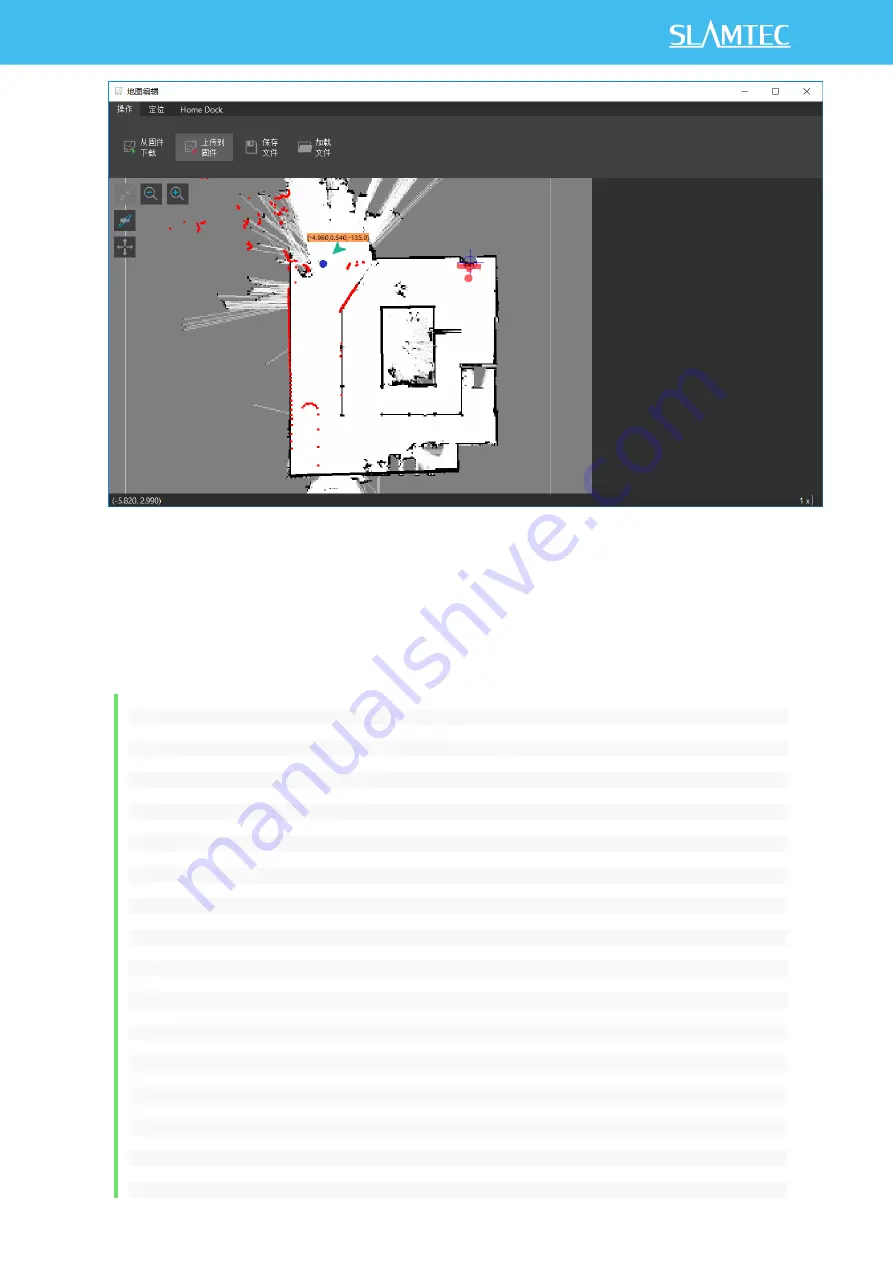
15 / 25
Copyright (c) 2016-2017 Shanghai Slamtec Co., Ltd.
Figure 4-5 RoboStudio Robot Map Editing Window
4.
Launch Host Computer and Load the Map
The following code shows how to finish loading specified map by using SDK on
the upper computer.
1.
/*
2.
* upload map file to apollo
3.
*/
4.
#include <iostream>
5.
#include <rpos\robot_platforms\slamware_core_platform.h>
6.
#include <rpos\robot_platforms\objects\composite_map_reader.h>
7.
#include <rpos\core\pose.h>
8.
9.
using
namespace
std;
10.
11.
int
main()
12.
{
13.
try
14.
{
15.
string map_path =
".\\map.stcm"
;
//the path of map
16.
string apollo_ip =
"192.168.11.1"
;
//the ip of apollo
17.
int
apollo_port = 1445;
//the port of apollo ,default is 1445
18.
19.
rpos::robot_platforms::SlamwareCorePlatform apollo =
20.
rpos::robot_platforms::SlamwareCorePlatform::connect(apollo_ip, apollo_port);
21.
//connect to the apollo
22.
rpos::robot_platforms::objects::CompositeMapReader cmapreader;
23.
//map reader
24.
rpos::core::Pose apollo_pose = rpos::core::Pose(rpos::core::Location(0, 0, 0));
25.
//the Apollo pose in map(apollo_pose should be the apollo's real pose in new map)
26.
//using apollo.getpose() to get the old apollo pose
27.
auto map = cmapreader.loadFile(map_path);
28.
//load map
29.
apollo.setCompositeMap(*map, apollo_pose);
30.
//set compositemap
31.
rpos::core::Pose home_pose = rpos::core::Pose(rpos::core::Location(0, 0, 0));
32.
//the home pose in map(home_pose should be the home's real pose in new map)