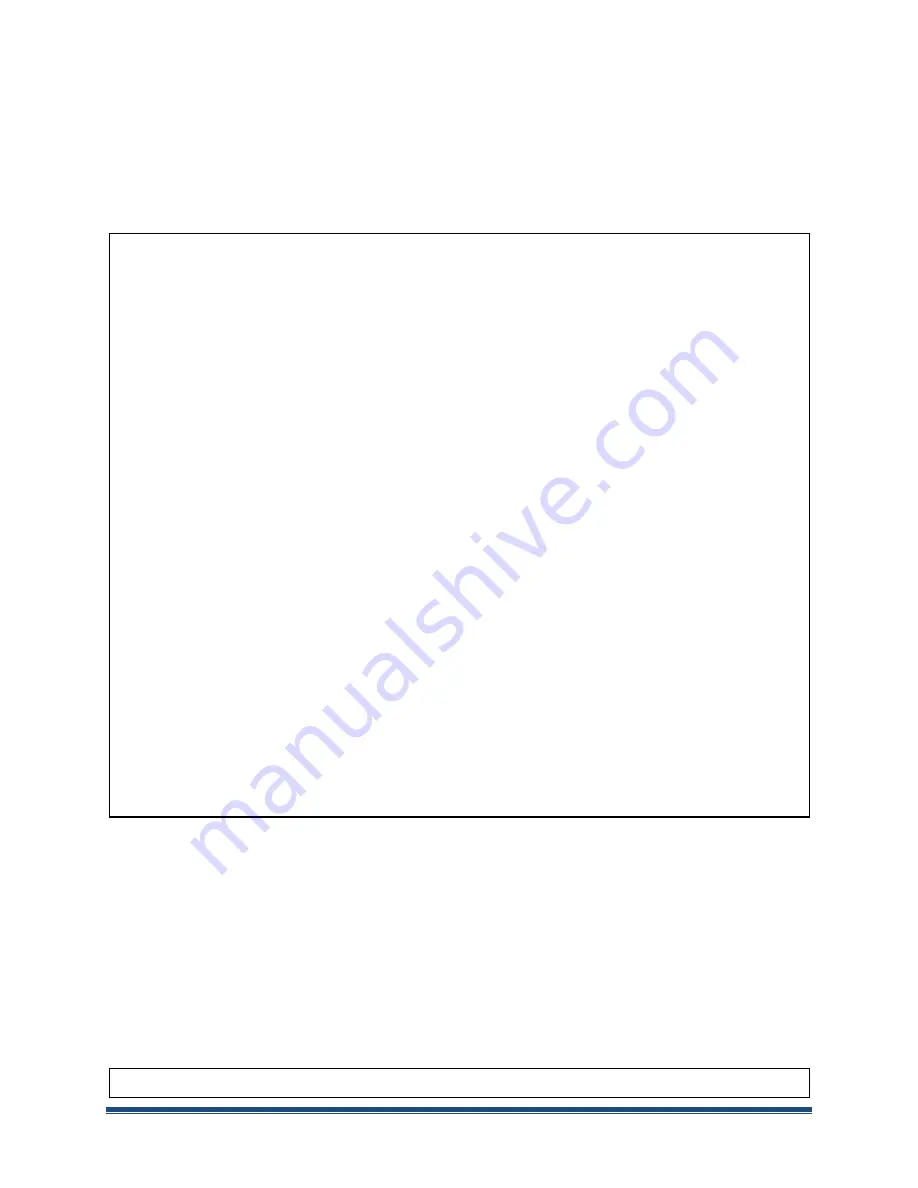
SC5313A Operating & Programming Manual
Rev 1.0.2
23
Function:
sc5313a_CloseDevice
Definition:
int
sc5313a_CloseDevice (
deviceHandle
*
deviceHandle)
Input:
deviceHandle
*deviceHandle
(handle to the device to be closed)
Description:
sc5313a_CloseDevice
closes the device associated with the device handle and turns off
the “active” LED on the front panel if it is successful.
Example:
Code to exercise the functions that open and close the device:
Function:
sc5313a_RegWrite
Definition:
int
sc5313a_RegWrite (
deviceHandle
*
deviceHandle,
unsigned
char
commandByte,
unsigned
long
long
int
instructWord)
Input:
deviceHandle
*deviceHandle
(handle to the opened device)
unsigned
char
commandByte
(register address)
unsigned
long
long
int
instructWord
(data for the register)
Description:
sc5313a_RegWrite
writes the instructWord data to the register specified by the
commandByte. See the register map on Table 4 for more information.
Example:
To set the frequency to 2 GHz:
int
status = sc5313a_RegWrite(devHandle, RF_FREQUENCY, 2000000000);
// set frequency to 2 GHz
// Declaring
#define
MAXDEVICES 50
libusb_device_handle *devHandle;
//device handle
int
numOfDevices;
// the number of device types found
char
**deviceList;
// 2D to hold serial numbers of the devices found
int
status;
// status reporting of functions
deviceList = (
char
**)malloc(
sizeof
(
char
*)*MAXDEVICES);
// MAXDEVICES serial numbers to
search
for
(i=0;i<MAXDEVICES; i++)
deviceList[i] = (
char
*)malloc(
sizeof
(
char
)*SCI_SN_LENGTH);
// SCI SN has 8 char
numOfDevices = sc5313a_SearchDevices(deviceList);
//searches for SCI for device type
if
(numOfDevices == 0)
{
printf(
"No signal core devices found or cannot not obtain serial numbers\n"
);
for
(i = 0; i<MAXDEVICES;i++) free(deviceList[i]);
free(deviceList);
return
1;
}
printf(
"\n There are %d SignalCore %s USB devices found. \n \n"
, numOfDevices,
SCI_PRODUCT_NAME);
i = 0;
while
( i < numOfDevices)
{
printf(
"
Device %d has Serial Number: %s \n"
, i+1, deviceList[i]);
i++;
}
//** sc5313a_OpenDevice, open device 0
devHandle = sc5313a_OpenDevice(deviceList[0]);
// Free memory
for
(i = 0; i<MAXDEVICES;i++) free(deviceList[i]);
free(deviceList);
// Done with the deviceList
//
// Do something with the device
// Close the device
status = sc5313a_CloseDevice(devHandle);