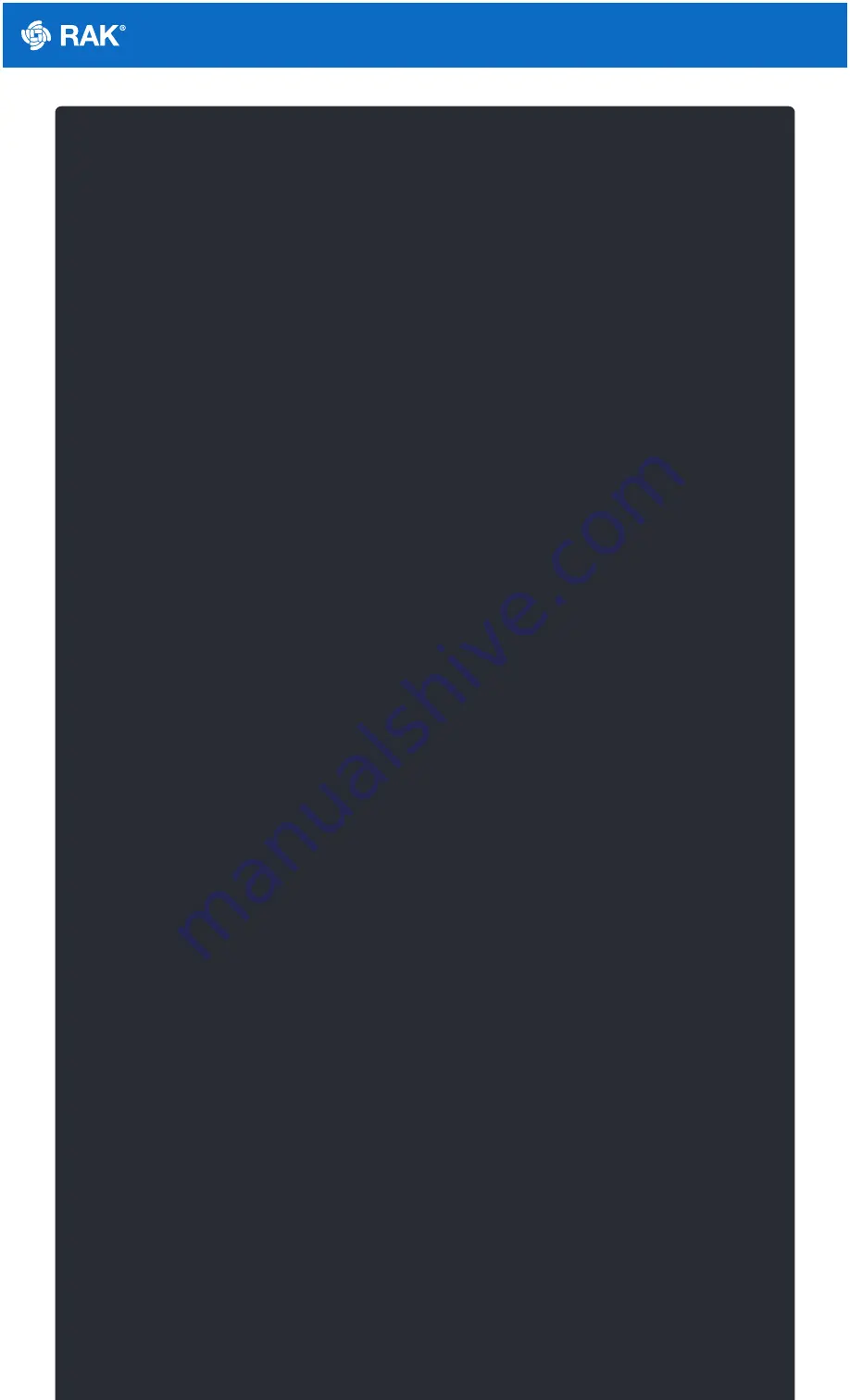
Documentation Center
/**
@file readMifareClassic.ino
@author rakwireless.com
@brief This example will wait for any ISO14443A card or tag, and depending on the size of the
@version 0.1
@date 2021-10-14
@copyright Copyright (c) 2021
**/
#
include
<Wire.h>
#
include
<SPI.h>
#
include
<RAK13600-PN532.h>
// Click here to get the library: http://librarymanager/All#RAK13600-
// If using the breakout or shield with I2C, define just the pins connected
#
define
PN532_IRQ
(
WB_IO6
)
#
define
PN532_RESET
(
WB_IO5
)
// Not connected by default on the NFC Shield
// Or use this line for a breakout or shield with an I2C connection:
NFC_PN532
nfc
(
PN532_IRQ
,
PN532_RESET
);
void
setup
(
void
)
{
Serial
.
begin
(
115200
);
pinMode
(
WB_IO2
,
OUTPUT
);
digitalWrite
(
WB_IO2
,
HIGH
);
delay
(
300
);
while
(
!
Serial
)
delay
(
10
);
// for Leonardo/Micro/Zero
Serial
.
println
(
"Hello!"
);
nfc
.
begin
();
uint32_t
versiondata
=
nfc
.
getFirmwareVersion
();
if
(
!
versiondata
)
{
Serial
.
(
"Didn't find PN53x board"
);
while
(
1
);
// halt
}
// Got ok data, print it out!
Serial
.
(
"Found chip PN5"
);
Serial
.
println
((
versiondata
>>
24
)
&
0xFF
,
HEX
);
Serial
.
(
"Firmware ver. "
);
Serial
.
((
versiondata
>>
16
)
&
0xFF
,
DEC
);
Serial
.
(
'.'
);
Serial
.
println
((
versiondata
>>
8
)
&
0xFF
,
DEC
);
// configure board to read RFID tags
nfc
.
SAMConfig
();
Serial
.
println
(
"Waiting for an ISO14443A Card ..."
);
}
void
loop
(
void
)
{
uint8_t
success
;
uint8_t
uid
[]
=
{
0
,
0
,
0
,
0
,
0
,
0
,
0
};
// Buffer to store the returned UID
uint8_t
uidLength
;
// Length of the UID (4 or 7 bytes depending on ISO14
// Wait for an ISO14443A type cards (Mifare, etc.). When one is found
// 'uid' will be populated with the UID, and uidLength will indicate
// if the uid is 4 bytes (Mifare Classic) or 7 bytes (Mifare Ultralight)
success
=
nfc
.
readPassiveTargetID
(
PN532_MIFARE_ISO14443A
,
uid
,
&
uidLength
);
if
(
success
)
{
// Display some basic information about the card
Serial
.
println
(
"Found an ISO14443A card"
);
Serial
.
(
" UID Length: "
);
Serial
.
(
uidLength
,
DEC
);
Serial
.
println
(
" bytes"
);
Serial
.
(
" UID Value: "
);
c