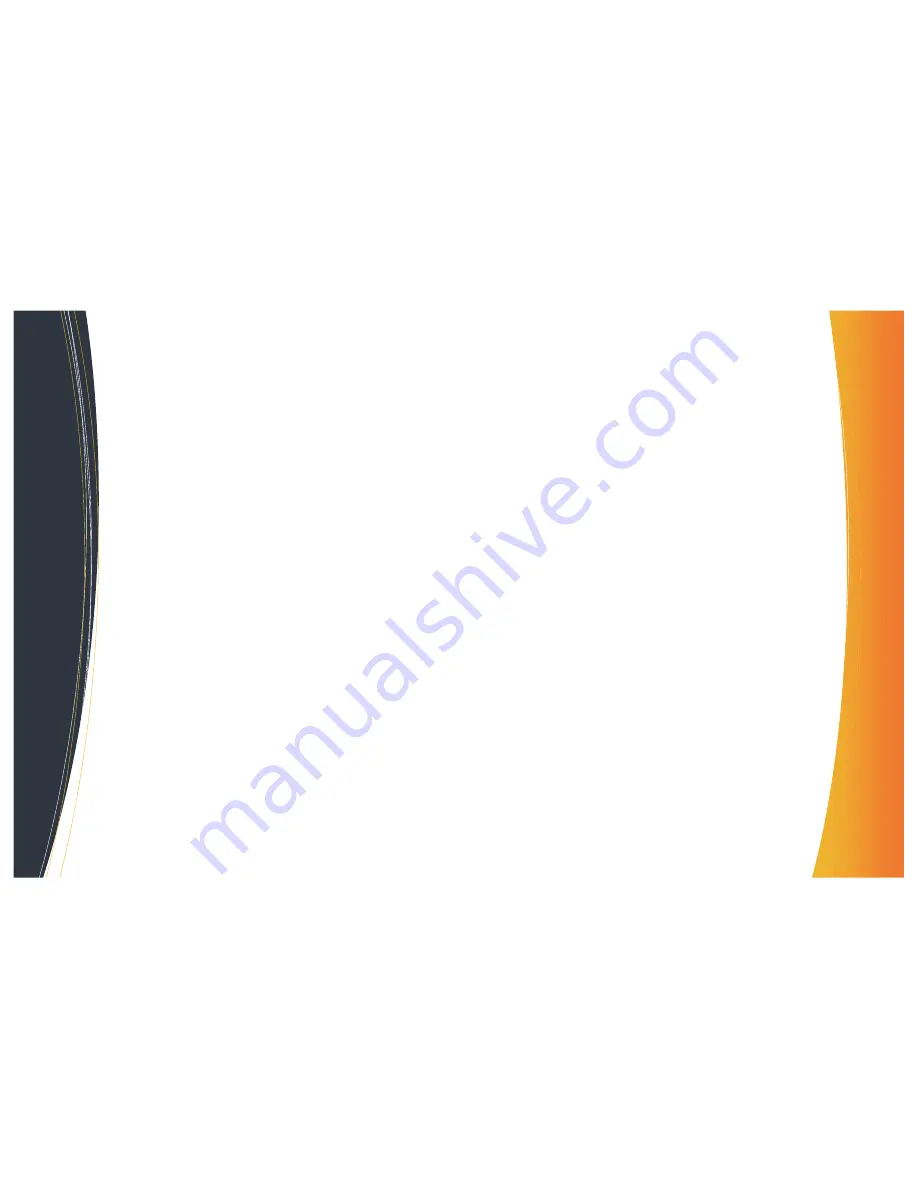
BIG 8051 Manual
34
Sample Project (Cain & Kelly)
Below is a fully functional program that may be run on the simulator. The program is designed to control the water level in a bucket used as part of a hydroponic garden. Switches 1,
2 and 3 on the board serve as stand ins for a trio of float sensors that will activate when the water level reaches a set height. The first four LEDs on the board correspond to the four
devices that the system needs to control. LED 0 represents the input valve, LED 1 represents an aerator that needs to run for 5 seconds each time a switch is flipped for the first time.
LEDs 3 and 4 represent the drain valve that will allow the water out (3), and a light that is required to be turned on while the bucket empties (4).
Recall that, as with the earlier exam-
ple programs, logic 0 is required to “light” the LEDs, so CLR is used to light them instead of SETB.3
main:
JNB F0, initialize
; F0 is a flag that is set when initialization has been run
once
SETB P1.1
; ensure that the aerator is off
JB P0.3, drain
;ifP0.3 is set, the bucket has finished filling and should begin to
drain
CLR P1.0
; Begin filling the bucket
JNB P2.3, Switch3
; is the third switch tripped? (note that the last switch must be the first checked so as to not get stuck)
JNB P2.2, Switch2
; Is the second switch tripped?
JNB P2.1, Switch1
;Is the first switch tripped?
JMP main
; continuously loop
Switch1:
JB P0.0, main
; If this function has been executed before return to main
SETB P0.0
; set as flag that this function has been executed
JMP aerate
; jump if this is the first time the switch read as pressed
Switch2:
JB P0.1, main
; If this function has been executed before return to main
SETB P0.1
; set as flag that this function has been executed
JMP aerate
; jump if this is the first time the switch read as pressed
Switch3:
JB P0.2, main
; If this function has been executed before return to main
SETB P0.2
; set as flag that this function has been executed
SETB P0.3
; set to indicate that bucket is full
JMP aerate
; jump if this is the first time the switch read as pressed
aerate:
SETB P1.0
; stop filling
CLR P1.1
; start aerator
JMP delay
; jump to delay
delay:
MOV R0,#20
; set loop count to 20
JMP countdown
; jump to countdown
countdown:
DEC R0
; Decrement R0
DJNZ R0,countdown
; keep looping until R0 hits 0
JMP main
; return to main
drain:
CLR P1.2
; open drain valve
CLR P1.3
; turn on light
JNB P2.1, drain
; keep looping until the water drops below the first sensor
SETB P1.2
; close drain valve
SETB P1.3
; turn off light
JMP initialize
; Reset the flags so the program can restart automatically
initialize:
; This functions sets flags to ensure certain functions are only run once (aerate once per switch, drain once)
CLR P0.0
; This will be set when the first sensor is tripped
CLR P0.1
; This will be set when the second sensor is tripped
CLR P0.2
; This will be set when the third sensor is tripped
CLR P0.3
; This will be set after the third switch is tripped to confirm that the bucket is full
SETB F0
; Set to confirm that this function has been run once
JMP main
; return to main