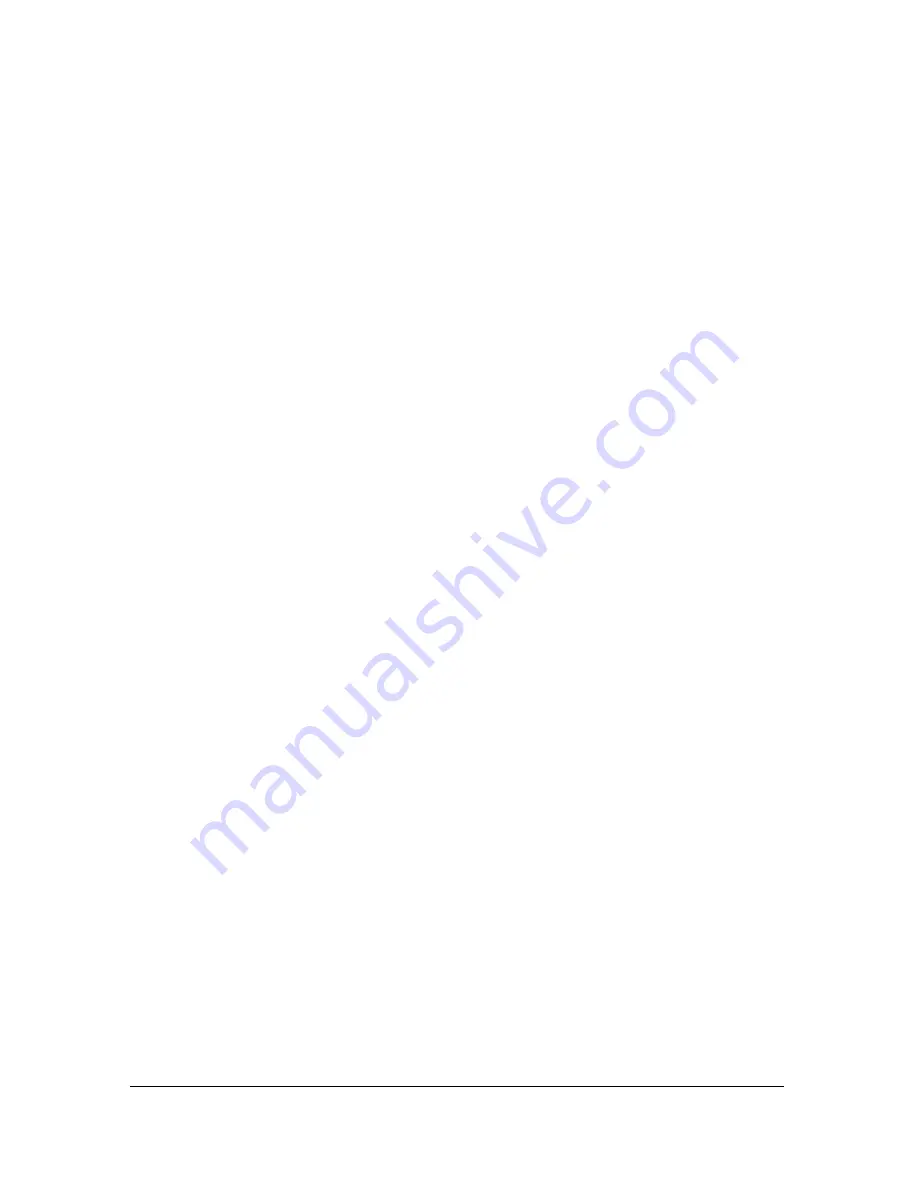
48
Server-Side ActionScript Language Reference
The previous example works, but must be executed every time a client connects. If you want
the same methods and properties to be available to all clients in the
application.clients
array without defining them every time, you must assign them to the
prototype
property of
the Client class. There are two steps to extending a built-in class using the
prototype
method. You can write the steps in any order in your script. The following example extends
the built-in Client class, so the first step is to write the function that you will assign to the
prototype
property:
// First step: write the functions.
function Client_getWritePermission(){
// The writeAccess property is already built in to the client class.
return this.writeAccess;
}
function Client_createUniqueID(){
var ipStr = this.ip;
// The ip property is already built in to the client class.
var uniqueID = "re123mn"
// You would need to write code in the above line
// that creates a unique ID for each client instance.
return uniqueID;
}
// Second step: assign prototype methods to the functions.
Client.prototype.getWritePermission = Client_getWritePermission;
Client.prototype.createUniqueID = Client_createUniqueID;
// A good naming convention is to start all class method
// names with the name of the class followed by an underscore.
You can also add properties to
prototype
, as shown in the following example:
Client.prototype.company = "Macromedia";
The methods are available to any instance, so within
application.onConnect
, which is
passed a
clientObj
argument, you can write the following code:
application.onConnect = function(clientObj){
var clientID = clientObj.createUniqueID();
var clientWritePerm = clientObj.getWritePermission();
};