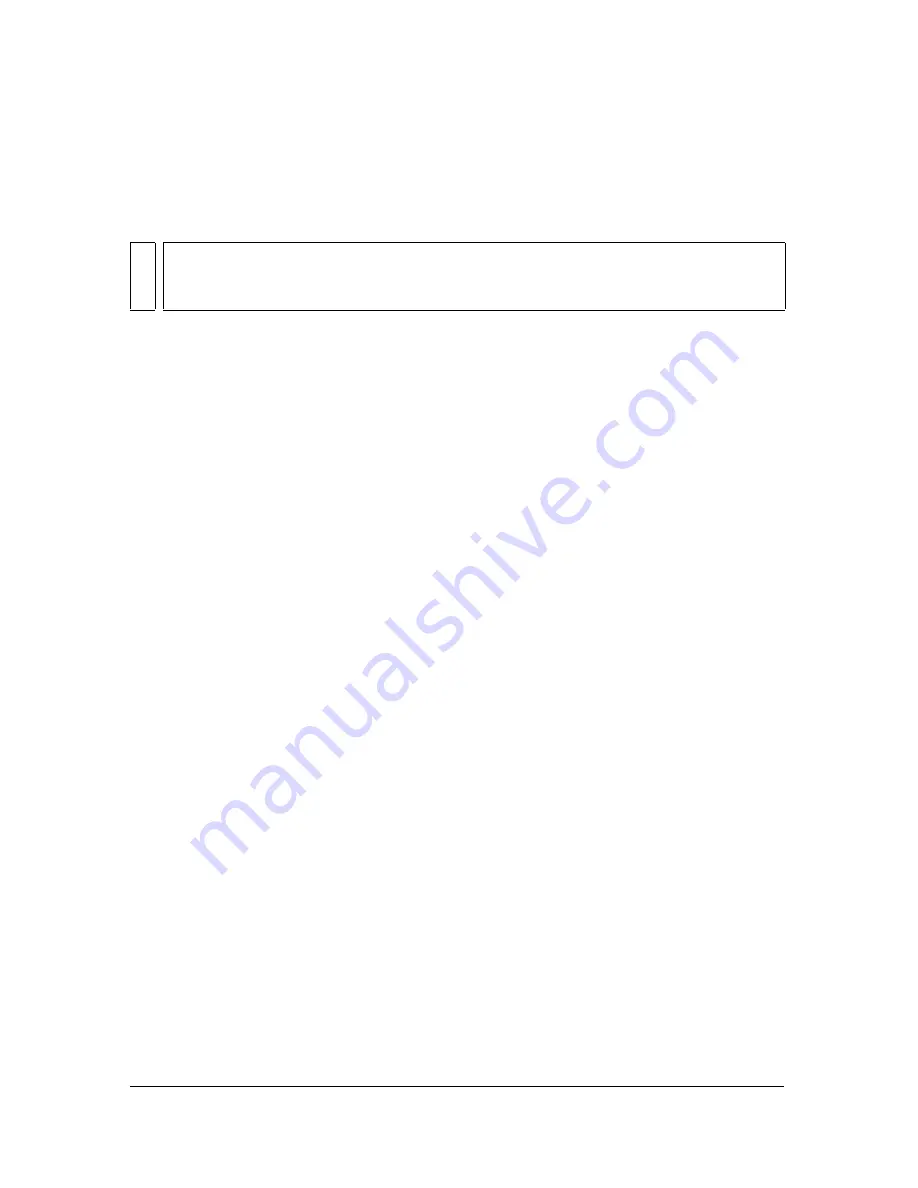
32
Server-Side ActionScript Language Reference
If there are several simultaneous connection requests for an application, the server serializes
the requests so there is only one
application.onConnect
handler executing at a time. It is a
good idea to write code for the
application.onConnect
function that executes quickly to
prevent a long connection time for clients.
Example
The following examples show the three basic uses of the
onConnect
handler:
(Usage 1)
application.onConnect = function (clientObj [, p1, ..., pN]){
// Insert code here to call methods that do authentication.
// Returning null puts the client in a pending state.
return null;
};
(Usage 2)
application.onConnect = function (clientObj [, p1, ..., pN]){
// Insert code here to call methods that do authentication.
// The following code accepts the connection:
application.acceptConnection(clientObj);
};
(Usage 3)
application.onConnect = function (clientObj [, p1, ..., pN])
{
// Insert code here to call methods that do authentication.
// The following code accepts the connection by returning true:
return true;
};
The following example verifies that the user has sent the password “XXXX”. If the password is
sent, the user’s access rights are modified, and the user can complete the connection. In this
case, the user can create or write to streams and shared objects in the user’s own directory and
can read or view any shared object or stream in this application instance.
// This code should be placed in the global scope.
application.onConnect = function (newClient, userName, password){
// Do all the application-specific connect logic.
if (password == "XXXX"){
newClient.writeAccess = "/" + userName;
this.acceptConnection(newClient);
} else {
var err = new Object();
err.message = "Invalid password";
this.rejectConnection(newClient, err);
}
};
NO
TE
If you are using the Component framework (that is, you are loading the components.asc
file in your server-side script file) you must use the
Application.onConnectAccept
method
to accept client connections. For more information see
Application.onConnectAccept
.